A clone of The Sound of Sorting I made just to test and diagnose my QuickSort algorithm.
It may sound a little ridiculous on web, since the array access sounds tend to get lumped together into far fewer frames.
- Press z to step
- Hold z to disable step mode
- Press x to switch sorting algorithm
- Use up and down to control the number of entries.
- Use left and right to control the speed.


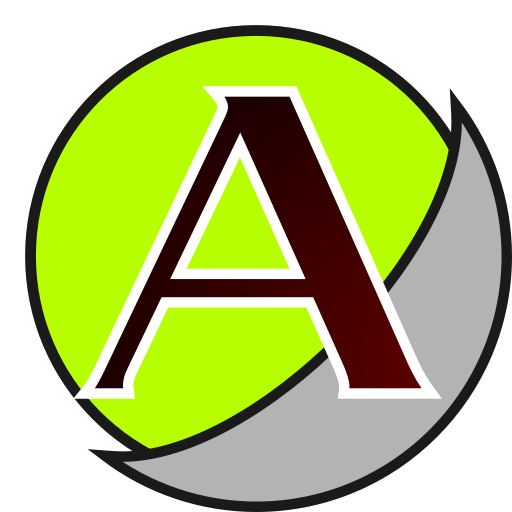
Oh, btw, here's the quicksort algorithm without all the extra baggage.



Who are you??? Some sort of dev that just KNOWS what people want? This is great! Are these all the sorts that will fit in the code? It looks and sounds just like the YouTube video!


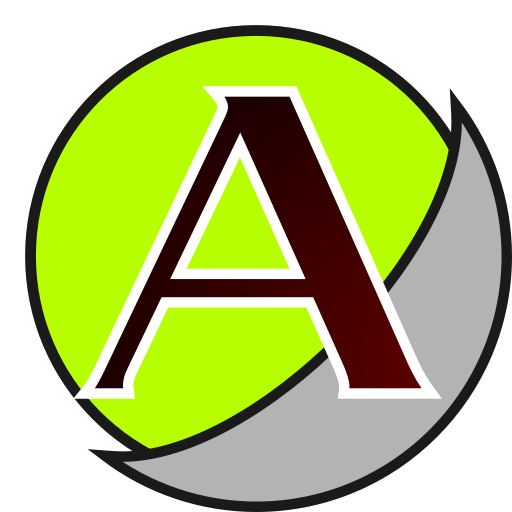
@Turbochop I'm a professional gamedev with many years of practice under my belt. So yeah, kinda.
The code can fit plenty more algorithms, and I designed the cartridge to be modular enough to just add more. I didn't 'cause I don't have the attention span to learn more sorting algorithms, but you are welcome to write one and submit it here, and I'll add it in.



@abledbody While I think sorting algorithms are really cool, I haven't the experience to code one myself. :( Maybe I could try to learn a bit, and see what I can come up with, if anything :D



@abledbody I managed to get a cocktail shaker sort working! For full transparency, I'd like to add that I used ChatGPT to generate this code. I also tried Radix, Heap, and Bitonic sorts but couldn't get them to work.


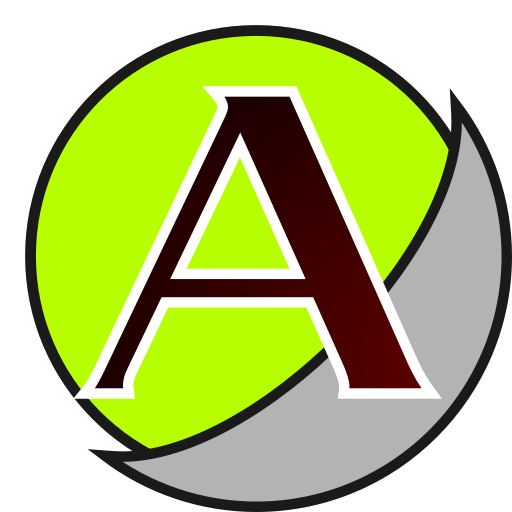
@Turbochop I've added the cocktail shaker algorithm, with a small optimization to move the low and high points to the last known swap so that they don't retread big sorted sections at the low and high ends.
I also fixed the visuals for bogo sort's validation step while I was at it.



BOGO sort is so frustrating to watch and might need a seizure warning lol....
[Please log in to post a comment]