Hi,
I'm sussing out some basic map collision utilizing fget(mget()) as one would for Pico-8 and I'm running into an issue where either large sprites aren't working correctly, or it's PEBKAC.
Specifically, it seems checking flags on any sprite that isn't 16x16 doesn't return the correct values, and any larger map sprite only reports correct flags for its upper left 16x16 corner. Smaller sprites
I've created a barebones sample to test this out after noticing in a proper project.
The gray squares are 16x16 for visual alignment. Yellow is a 32x32 player sprite, with each collision point along its edges visualized with pink. The other colored sprites are directly part of the map and and go from 16x16, 32x32, and 64x64. On collision, the point of contact (with the player's edge points) is added to a global render list for its own visualization, simple pink dots as well. We can see on each sprite that the TL 16x16 is the only point of contact.
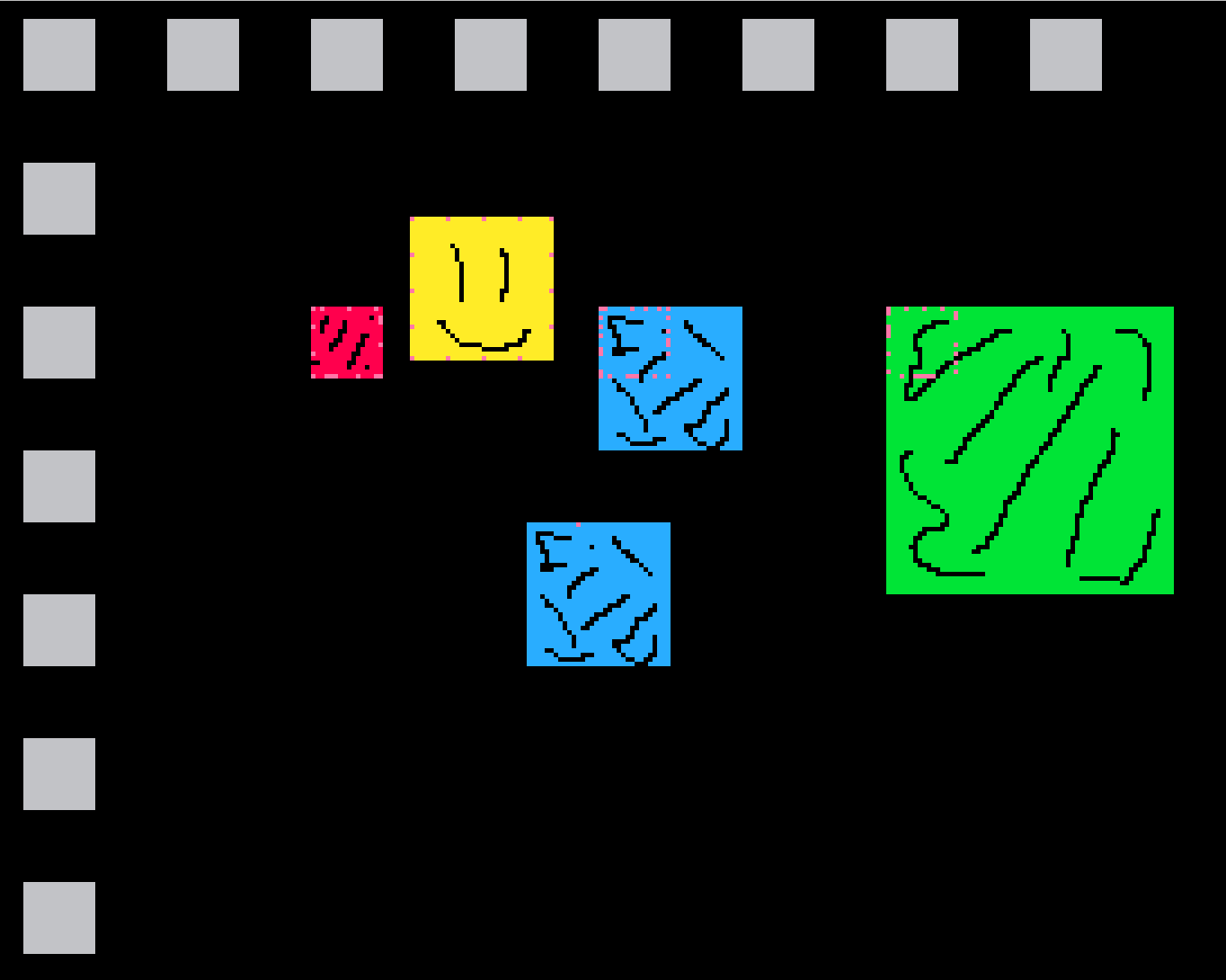
Outside of the provided image, I also have my cart now rendering the actual sprite contacted in the upper left of the screen to confirm it's hitting what it thinks it is. I also did some toying around with further visualizations to get an idea of where my collider/mget thinks we're hitting, but it all seems cogent.
My biggest concern is the arguments to mget(). Pico-8 documentation simply suggests x,y coordinates without specifying pixels or map tiles, and any Picotron documentation doesn't really suggest things correctly.
Out of curiosity, I added this snippet https://www.lexaloffle.com/bbs/?tid=141387 to see what it does with mget. It seems to pass plain pixel coords to mget() for sprite info, but it also doesn't seem to think I'm hovering over a sprite 99% of the time. Passing the tile coords instead is more fruitful, but produces the same issue. Am I wrong in assuming map tiles are 16x16? The map editor certainly suggests it, though curiously it also fails to render larger sprites beyond their 16x16 TL corner.
Anyone else run into this, or not have this issue? I understand custom sprite sizes are new to Picotron so it's been difficult to find resources. Hope I covered everything well enough. Thanks!
function check_map_collision(actor) local hit_resolution = 8 checkpoints = {} -- generate a set of points on each edge of the actor for px = actor.x, actor.x+actor.w-1, hit_resolution do add(checkpoints, {x=px, y=actor.y}) end for px = actor.x, actor.x+actor.w-1, hit_resolution do add(checkpoints, {x=px, y=actor.y+actor.h-1}) end for py = actor.y, actor.y+actor.h-1, hit_resolution do add(checkpoints, {x=actor.x, y=py} ) end for py = actor.y, actor.y+actor.h-1, hit_resolution do add(checkpoints, {x=actor.x+actor.w-1, y=py}) end -- check map sprite flags at each point for collision for each in all(checkpoints) do -- find nearest map sprite increment mapx = (each.x)/16 mapy = (each.y)/16 sprite_at_point = mget(mapx, mapy) flags_at_point = fget(sprite_at_point, flag) if (flags_at_point & (2^flag)) == 1 then -- collision happened at this edge point return {x=each.x, y=each.y} end end -- no collision! return nil end |


While this doesn't entirely solve your issue, you can change the default map tile size by changing sprite 0's dimensions. If you change sprite 0 to have a 32x32 canvas, the map tile size will change to that as well. Hope that helps :)


Good to know, thanks. I did manage to observe this recently as well. Unfortunately it still doesn't get around the need for support for higher-resolution sprite detection, I think for now any large map sprites will have to be split up into respective component sprites.
Perhaps it's more telling when looking at the map editor -- larger sprites are visually cropped to map tile dimensions exactly as they are with collision.
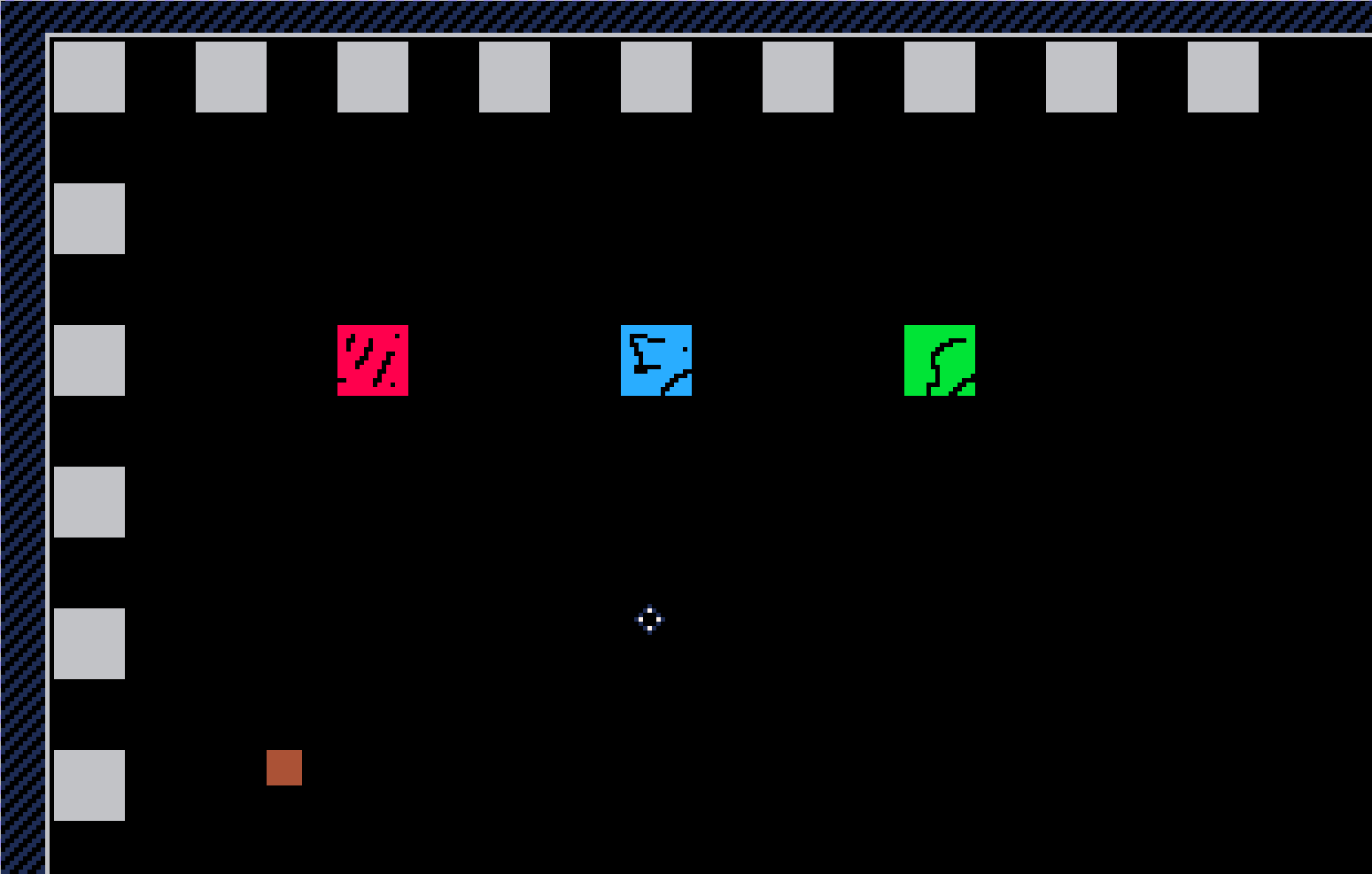
0.1.0f, ho!
[Please log in to post a comment]