I wrote a function that shows the mouse coordinates and sprite number of the map tile you are hovering over. I originally wrote this for myself to make checking the tiles on my map easier but figured I could share it in case anyone else finds it useful.
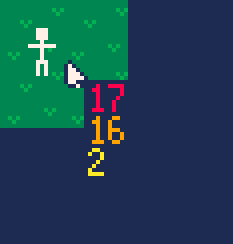
function debug_mouse() local mx,my = mouse() local x_offset=5 local y_offset=5 --window width and height local w=480 local h=270 --offset if box leaves screen if mx>w-20 then x_offset=-15 end if my>h-29 then y_offset=-24 end --draw debug text box rectfill(mx+x_offset-1,my+y_offset-1,mx+x_offset+14,my+y_offset+23,1) print(mx,mx+x_offset,my+y_offset,8) print(my,mx+x_offset,my+y_offset+8,9) print(mget(mx,my),mx+x_offset,my+y_offset+8*2,10) end |


Thank you for the script, however it wasn't working correctly because it looks like coords for mget work off cell coords rather than pixels, I modified the script to correct that and also add flag output
function debug_mouse() local mx,my = mouse() local x_offset = 5 local y_offset = 5 -- tile size local tw=16 local th=16 -- window width and height local w=480 local h=270 -- offset if box leaves screen if mx>w-20 then x_offset=-15 end if my>h-29 then y_offset=-24 end -- draw debug text box local tile_x = mx/tw local tile_y = my/th local sprite = mget(tile_x,tile_y) local flag = fget(sprite) rectfill(mx+x_offset-1,my+y_offset-1,mx+x_offset+14,my+y_offset+23+8,1) print(mx/tw,mx+x_offset,my+y_offset,8) print(my/th,mx+x_offset,my+y_offset+8,9) print(sprite,mx+x_offset,my+y_offset+8*2,10) print(flag,mx+x_offset,my+y_offset+8+8*2,10) end |


have you found out how to round the coordinates to integer? I've been trying to use the flr() function but it's still showing the decimal part
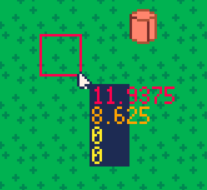


@MaddoScientisto as you see mget should function with non-integers. If you still want to round flr seems to work fine in my tests.
print(flr(mx/tw),mx+x_offset,my+y_offset,8) |
The other option is to use the floor division operator //
print(mx//tw,mx+x_offset,my+y_offset,8) |


@instring thank you, yes it did work but I wanted to format the output string without the decimals, in the end I found out that using the integer division operator "\" works as well, plus I learned how to use string.format so here's the updated code:
function debug_mouse() local mx,my = mouse() local x_offset = 5 local y_offset = 5 -- tile size local tw=16 local th=16 -- window width and height local w=480 local h=270 -- offset if box leaves screen if mx>w-20 then x_offset=-15 end if my>h-29 then y_offset=-24 end -- draw debug text box local tile_x = mx\tw local tile_y = my\th local sprite = mget(tile_x,tile_y) local flag = fget(sprite) rect((tile_x*tw)+tw,(tile_y*th)+th,tile_x*tw,(tile_y*th),8) rectfill(mx+x_offset-1,my+y_offset-1,mx+x_offset+14,my+y_offset+23+8,1) print(string.format("%d (%d)",tile_x,mx),mx+x_offset,my+y_offset,8) print(string.format("%d (%d)",tile_y,my),mx+x_offset,my+y_offset+8,9) print(sprite,mx+x_offset,my+y_offset+8*2,10) print(flag,mx+x_offset,my+y_offset+8+8*2,10) end |
As a bonus I even added a square around the affected tile, I only didn't bother changing the size of the coords box
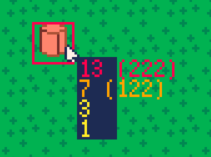


@369369369 I would put the call to it at the end of your draw function. The actual function definitition can go anywhere in the file.
function _draw() cls() map() debug_mouse() end |
If you haven't already make a few test sprites and make a sample map. It doesn't have to be fancy, just so you have something appear on screen when map() is called.


This is nice! Here's my version with some edits:
- works with games that use camera()
- works with games that use different vid() modes
- text will always stay onscreen
- more descriptive text
function debug_mouse() -- tile size local tw,th = 16,16 local mx,my = mouse() local cam_x,cam_y = camera() --reset temporarily -- find window size local scrw,scrh = 480,270 local video_mode = @0x547c if (video_mode==3) scrw\=2 scrh\=2 if (video_mode==4) scrw\=3 scrh\=3 -- gather tile info local tx = (mx+cam_x)\tw local ty = (my+cam_y)\th local tile = mget(tx,ty) or 0 local flags = fget(tile) -- highlight tile camera(cam_x,cam_y) rect(tx*tw-1,ty*th-1,tx*tw+tw,ty*th+th,7) camera() -- draw mousemap info local text = --string.format("\#1mouse():%d,%d",mx,my).."\n".. string.format("\#1mget(%d,%d):%d",tx,ty,tile).."\n".. string.format("\#1fget(%d):%d",tile,flags) local textw,texth = print(text,0,10000) --measure texth -= 10000 -- clamp onscreen local x = mid(0,scrw-1-textw, mx+4) local y = mid(0,scrh-1-texth, my+4) print(text,x,y,13) --draw -- restore camera camera(cam_x,cam_y) end |
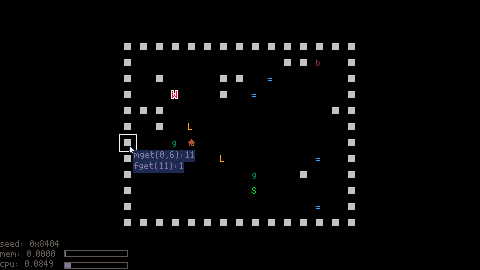
[Please log in to post a comment]