When I go to view the source code for carts, I keep seeing "I=1" pop up, and I can't figure out what it is referring to. Also, I'm not really sure how functions for a variable are created. When the code is using If...Then...Else statements I can generally follow along, but looking at the functions they're assigned to I'm just very lost. To me, it just looks like random words and numbers strung together with no correlation. Thank you in advance, I'm really looking to learn :]



I=1 set a variable I with the value 1. Normaly when a variable has a so short name, it is for a counter or a temporary variable.
It is difficult to explain, you haven't written a question... I don't know where to start to explain. Have you any programming experience?
Also important, many bigger projects codes are complicated especially in Pico8 because of the token-limit. For big project programmers must optimize to a more "unreadable" state.
Maybe some videos like https://www.youtube.com/watch?v=YQzwVDMIfyU&list=PLea8cjCua_P0qjjiG8G5FBgqwpqMU7rBk will help you.
And start simple with your first project - try to program thinks like Master Mind https://en.wikipedia.org/wiki/Mastermind_(board_game) , tic-tac-toe or flappy-birds. Simple things!
Functions are sub routines. For example, when you want the distance from two points ( https://www.redcrab-software.com/en/calculator/distance-of-points ) you can write everywhere:
distance=sqrt((x1-x2)*(x1-x2) + (y1-y2)*(y1-y2)) |
everytime you need it, but it is not easy to read. You must know and reconize the formula for example
you can put this forumla in a function.
function GetDistance(x1,y1,x2,y2) return sqrt((x1-x2)*(x1-x2) + (y1-y2)*(y1-y2)) end |
now you can write everywhere in the sourcecode forexample GetDistance(10,2,5,2) to get the Distance between the points 10x2 and 5x2.


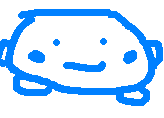
> I'm not really sure how functions for a variable are created.
Could you rephrase this or give an example? I don’t understand the question.


I apologize for being vague, I was very tired when I wrote this. For example, in this excerpt from Celeste 2:
function (px, py) if px > 420 then if px < 436 then c_offset = 32 + px - 420 elseif px > 808 then c_offset = 48 - min(16, px - 808) else c_offset = 48 end else c_offset = 32 end camera_target_x = max(0, min(level.width * 8 - 128, px - c_offset)) end, |
I don't really understand what this does, and how I would figure out what to write if I were making the game.
Not sure if this clears it up. I guess I'm saying I don't know how to translate what I want to happen in the game into math and code. Any tips for branching that gap better?



So without any context, you really can't know for sure what that function does. It's anonymous, so it doesn't even have a name! Which, is why it's always stressed to name your variables and functions something that anyone can understand at a glance, and comment your code where needed to make things clearer. Also, random numbers don't give clues either, so generally it's good to label what the numbers mean, instead of just leaving them as "magic numbers."
Now, I could take a cheap shot, and find the twitter thread of people lamenting over C# Celeste's camera code (and every single other game's public source code), but as GPI said, Pico8's limits and resolution force you to cut character counts. So It's fairly common to see some ugly stuff in Pico8 games, as "good coding practices" get thrown out the window. So don't hold it against anyone. Not yourself for having a hard time understanding it, nor the person who wrote it.
That being said, some of it could be assumed. Generally, anything with x or y at the end is usually a coordinate, so, I'd guess it's either playerX or positionX. Since the last line is setting the camera target, I'd assume c_offset is for the camera's offset position.
So, to be sure, you'll need to understand the context of where and how this is used. Let's dig for it. Going into the code, it seems it is indeed being used to set the camera mode. Your code snippet turns out to be a function inside a table of camera functions.
camera_modes = { -- Camera functions here... -- [your code snippet] -- more camera functions... } |
And in player update, this table of functions is called:
-- camera camera_modes[level.camera_mode](self.x, self.y, on_ground) camera_x = approach(camera_x, camera_target_x, 5) camera_y = approach(camera_y, camera_target_y, 5) camera(camera_x, camera_y) |
So, pX and pY where indeed the player's x and y positions, and the function was for setting the camera position. So, that mean's the numbers in the if-else statements are for checking where the player is, and positioning the camera appropriately. But again, without knowing the outside context of the code, one could only guess (however educated that guess is) what any of it did. For all we knew, pX stood for "Palatine-bone Xray."
So, I hope that helps you when you're looking over code in the future. It's not just what's written down in a single line or function, but the context of where and how it's used too.
While peeking into other carts can be fun and educational, I wouldn't suggest starting there. Following tutorials and other resources would be a better place to look. You'll learn much faster, and gain a better understanding in general. So don't feel too stressed out for not understanding other people's code, that's putting the cart before the horse.



What also is important, in LUA a variable can hold as value a number, text, table, booleon, nil or function.
in fact
function name(parameter)
is a shortcut for
name = function(parameter)
also funny things like here are possible:
offset=10 function f1(p1) local offset local privat=function(b) offset=b end privat(1) print("inside:"..offset) privat(2) print("inside:"..offset) privat(p1) print("inside:"..offset) end cls() ? "outside:"..offset f1(99) ? "outside:"..offset |
there are two "offset"-variables, the global "offset", outside the function "f1", and a local in the function "f1". These are two complete different variables!
Inside the function F1 is a local function "privat". this function use also offset, but this is the offset of the function "f1" - because it is inside "f1". the nested "privat"-function inherited all variables from F1. To confuse more, it is possible, to return the "privat"-function" as a return-value.
Thats a special thing of LUA - in other languages like C this would be total impossible.
@All
is there a list of very basic and easy pico8-projects? I don't think that projects like celest is a good starting point for beginners. A good showcase of course, but not a good project to analyse and learn.



Hey bythemeadow! I'm going out on a limb here and guessing you're new to coding. If that's the case, the best way to learn more about how to make games is to find some good tutorials.
https://www.youtube.com/watch?v=J1wvvbVQ5zo is a good youtube tutorial series where you make a little top-down adventure game.
https://nerdyteachers.com/ is a good resource with lots of different videos covering different topics.
As you learn more about code, eventually you'll be able to look at a game like Celeste 2 and see how they do certain things :)


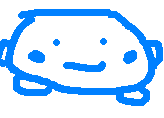
@GPI it’s not: celeste is a featured game, not one of the demos (cast, collide, dots3d, etc) from install_demos.


Thank you so much everyone! I really really appreciate it :]
This helps a lot.
[Please log in to post a comment]