Hi everyone!
I am making a little game where you scare off crows to protect your vegetable garden:
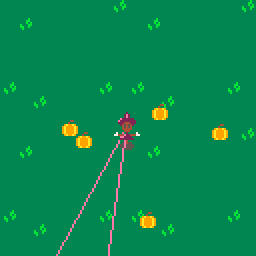
I am trying to make the crows pick a vegetable to target when they spawn. I have a function that will make them chase down a point by entering its X and Y value. My problem is that I don't know how to extract the vegetables' X and Y values because of how I've set things up.
Every vegetable is decided at random at the game's outset, and stored inside another table, like so:
[note: dif is an integer, adding more vegetables at higher difficulties] function setup() for i=1,dif do add(vegetables, { x=originx+(rnd(40)+10)*cos(rnd(360)/360), y=originy+(rnd(40)+10)*sin(rnd(360)/360), w=6, h=6, [more variables and stuff you don't need to care about...] }) end end |
My question is, how would I go about accessing the X and Y values for these? I have been trying something like this...
vegselect=flr(rnd(dif))+1 vegx=vegetables[vegselect][1] vegy=vegetables[vegselect][2] |
...but it has failed as a nil value every time.
Any advice is appreciated. Thanks for considering my problem!
EDIT: I classified the post incorrectly. Apologies!


.png)
That worked! Thank you so much. I sometimes struggle with basic syntax. I appreciate your help!



vegx = vegetables[vegselect].x vegy = vegetables[vegselect].y |
or:
vegx = vegetables[vegselect]["x"] vegy = vegetables[vegselect]["y"] |
or:
some_variable = "x" another_variable = "y" vegx = vegetables[vegselect][some_variable] vegy = vegetables[vegselect][another_variable] |
All combined in a FOR-Loop:
some_variable = "x" another_variable = "y" for vegetable in all(vegetables) do print(vegetable.x) print(vegetable.y) -- Output the same Values again print(vegetable["x"]) print(vegetable["y"]) -- Output the same Values again print(vegetable[some_variable]) print(vegetable[another_variable]) print("---") end |
In my humble Opinion, Tables are a bit "strange" in Lua (and so in PICO8): Think of Tables as a "Key-Value"-Variable. You can use a Key(word), to store Information under that Key inside the Table. For example "x" and "y", or "1", "2", "3" (which will be used by "add" and "del", making the table looks like sequential)...


.png)
This explanation is also quite helpful. Thank you, Astorek. Both of you have put me on the right track!
[Please log in to post a comment]