I feel like I'm wasting a lot of tokens with counters/timers in my game.
For example, most of my timers work like this:
timer -= 1 if timer <= 0 then timer = timer_reset --do stuff here end |
So I'm using a lot of global variables to store the timer values and timer reset values, as well as it not being a function in itself. I did try to make a function from it with something like:
function(timer, timer_reset) timer -= 1 if timer <= 0 then if timer_reset ~= nil then timer = timer_reset end return true else return false end end |
But that only reduces the timer variable within the function, and not the original. When looking up on if there was a way to reference the original variable, I just found posts saying this isn't possible in Lua.
Is there a better method for this?


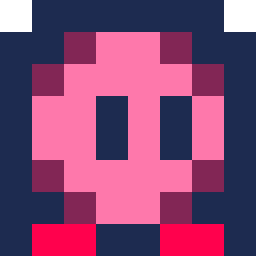
hmmm...
you could make some kinda callback thing
like so
function timer(timers,callback,callparams,calltime) add(timers,{f=callback,p=callparams,c=calltime,t=0}) end function timerupd(timers) --loop through timers for timer in all(timers) do --increment timers timer.t+=1 --if the timer has expired run it with the parameter table if(timer.t>=timer.c)timer.f(unpack(timer.p)) del(timers,timer) end end timers={} --create an array for timers timer( timers,--the timer array print,--any func {"hi"},--table of params 60--60/30fps=2 sec ) --this prints "hello world" after 2 seconds (at 30fps) --remember that you can also do function() -code- end as a parameter. while true do timerupd(timers)--call once per frame flip()--use this to go to the next frame without _update end |
feel free to shorten everything, i was just making it readable



coroutines are a way to achieve that but won’t scale that much.
You can use closures to make it more self contained and more aligned with your initial ifea:
function schedule(time,func,...) local tick=function() time-=1 if(time<0) func(...) return return tick end return tick end — example local print_2s=schedule(60,print,”hi”,64,64,7) function __draw() cls() — update timer until it elapses if(print_2s) print_2s=print_2s() end |


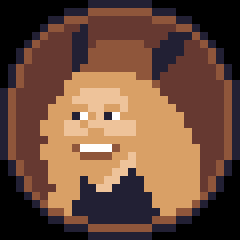
Thanks for the advice guys.
In the end I just stuck with my original timers, as yeah, it didn't scale much.
[Please log in to post a comment]