------------------------------------------------------------------------ -- takes a string describing a map and the width of the map -- other parameters reimplement map() -- example "0123456789abcdef",4 represents this 4x2 map: -- [[0x01,0x23,0x45,0x67],[0x89,0xab,0xcd,0xef]] function mapstring(mapstr, mapw, celx, cely, sx, sy, celw, celh, layer) -- remove[] to save tokens by making parameters mandatory ms, celx, cely, sx, sy, celw, celh, layer = ms or "", celx or 0, cely or 0, sx or 0, sy or 0, celw or 1, celh or 1, layer or 0 for y=cely, cely+celh-1 do for x=celx, celx+celw-1 do local sprnum = tonum("0x"..sub(mapstr,(y*mapw+x)*2+1,(y*mapw+x)*2+2)) if sprnum>0 and band(layer, fget(sprnum))==layer then spr(sprnum, sx+(x-celx)*8, sy+(y-cely)*8) end end end end |
This might be useful for games that define a large number of small maps (like metroid or zelda screens).


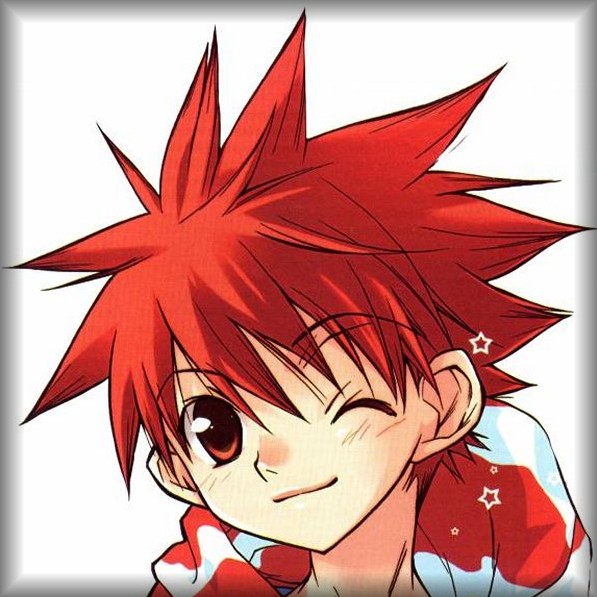
If you want to add an argument to ensure the area beneath the map is always cleared first, you can use this:
------------------------------------------------------------------------ -- takes a string describing a map and the width of the map -- other parameters reimplement map() -- example "0123456789abcdef",4 represents this 4x2 map: -- [[0x01,0x23,0x45,0x67],[0x89,0xab,0xcd,0xef]] function mapstring(mapstr, mapw, celx, cely, sx, sy, celw, celh, layer, solid) -- remove[] to save tokens by making parameters mandatory ms, celx, cely, sx, sy, celw, celh, layer = ms or "", celx or 0, cely or 0, sx or 0, sy or 0, celw or 1, celh or 1, layer or 0 if (solid) palt(0,false) for y=cely, cely+celh-1 do for x=celx, celx+celw-1 do local sprnum = tonum("0x"..sub(mapstr,(y*mapw+x)*2+1,(y*mapw+x)*2+2)) print(sprnum) if band(layer, fget(sprnum))==layer then spr(sprnum, sx+(x-celx)*8, sy+(y-cely)*8) end end end palt(0,true) end |
As it is an optional argument if you do not use it, it will not be enabled. Any non FALSE or NIL value such as TRUE, 1, or "B" will enable it.
Mode resets black to transparent afterwards.
Gold star for your innovative function, @sparr !



I feel like setting transparency in this function is unlikely to provide any significant time or token savings over the user just using their existing palt settings, or setting new ones themselves.
Also I prefer to avoid resetting the pal and palt settings; they might have been customized by the user before calling, and other draw functions don't overwrite them.
Also worth noting is that both of our functions actually draw sprite #0, unlike map() which skips it. I'll edit mine to change that.



Alternately you could just load data into the map, so you can use map() to draw it.
------------------------------------------------------------------------ -- takes a string and width describing a map, two hex digits per tile -- replaces existing map data, starting at celx,cely in the main map space -- example: string_to_map("0123456789ab",3,2,1) will do the following: -- mset(2,1,0x01) mset(3,1,0x23) mset(4,1,0x45) -- mset(2,2,0x56) mset(3,2,0x89) mset(4,2,0xab) function string_to_map(mapstr, mapw, celx, cely) -- remove[] to save tokens by making parameters mandatory mapstr, mapw, celx, cely = mapstr or "", mapw or #mapstr/2, celx or 0, cely or 0 for y = 0, #mapstr/mapw/2-1 do for x = 0, mapw-1 do mset(celx + x, cely + y, tonum("0x" .. sub(mapstr,(y * mapw + x) * 2 + 1,(y * mapw + x) * 2 + 2))) end end end |
[Please log in to post a comment]