
I'm just starting out in PICO-8 and messing around with OOP-style prototypal inheritance, so I tried to run the following code:
myclass = {str = "printme"} function myclass:new(o) o = o or {} setmetatable(o,self) self._index = self return o end subclass = myclass:new() print(subclass.str) |
...and it prints [nil] .
Should it not print "printme"? What am I doing wrong here?



I must confess, I never use "setmetatable" to do some OOP-Things. I always do something like:
function actor(x, y, sprite) local self = {} self.x = x self.y = y self.sprite = sprite or 0 function self.draw(self) spr(self.sprite, self.x, self.y) end return self end myactor = actor(0, 0, 1) myactor.draw(myactor) |
(Surely not optimal, but better than nothing...)
Also, don't forget that PICO-8 does not have the OOP-'Syntactic Sugar' with Dot and Colon! In Lua, the following
mytable:myfunction(x, y) |
is the equivalent of
mytable.myfunction(mytable, x, y) |
But on PICO-8, a Colon is just another allowed Char like any Letter...


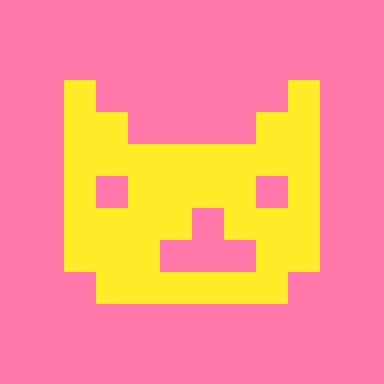
> Also, don't forget that PICO-8 does not have the OOP-'Syntactic Sugar' with Dot and Colon!
hmm I'm not sure what led you to believe that, but I am happy to report that PICO-8 does support that colon syntactic sugar and I have used it for years :)


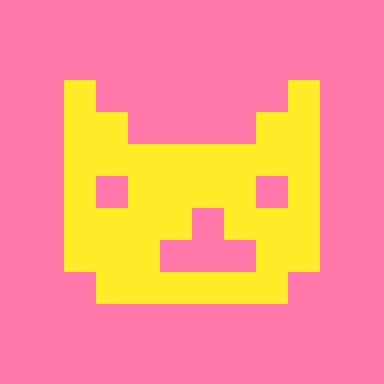
@FuzzyDunlop
it looks like you are just missing an underscore :) The metatable key you are trying to use is called __index
Note that you can simplify by just setting the __index for the class once; there's no point in setting it again every time a new object is created.
so you could do something like this:
myclass = {str = "printme"} myclass.__index = myclass function myclass:new(o) o = o or {} return setmetatable(o, self) end subclass = myclass:new() print(subclass.str) |
(also the setmetatable method returns the object, so I simplified that part too)
btw the code snipped you pasted might be incomplete, but I would not call that variable "subclass"; it's just an instance, not a subclass.



God damn it. Thanks.
As for assigning the __index property inside of the "new" method, I believe there is a point to it. It allows you to create subclasses of subclasses, like so:
myclass = {str = "printme"} function myclass:new(o) o = o or {} setmetatable(o,self) self.__index = self return o end subclass = myclass:new() subclass.str = "printme2" subclass2 = subclass:new() print(myclass.str) --"printme1" print(subclass2.str) --"printme2" |
If you only define the __index of myclass at the top instead of inside of the "new" method, then subclass2.str will print "[nil]".


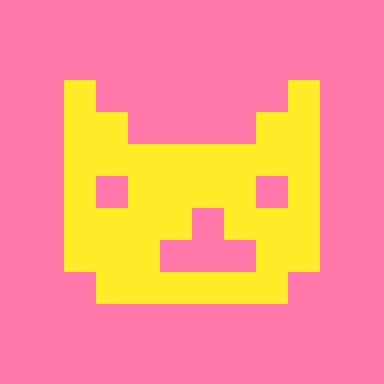
@FuzzyDunlop
haha glad to help
oh interesting; i never use any inheritance in Lua so I never realized that would be necessary for that. Thanks for the explanation.
[Please log in to post a comment]