This will loop sprite 1 through 4
T = 1 T += 1 SPR (1 + T % 60 / 30 * 2) |
I was trying to figure out some simple math that basically does what the above code does.
I found that code in a demo cart and was happy to see that I was on the right track (sort of) but I don't understand what is going on here.
If I can't figure this out maybe I'm just not smart enough for this whole programming thing :(


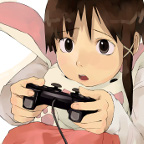
% is the modulus operator; x%y gives you the remainder of x/y. It's commonly used to make numbers loop around a limited range. When t=0, 60, 120, 180..., t%60 is 0. When t=17, 77, 137, 197..., t%60 is 17. When t=59, 119, 169, 239..., t%60=59.
So in short, with t increasing by one each frame, t%60 loops over 0-59 repeatedly.
x/30*2 is the same as x/15. It's simply translating the numbers to a lower value. 7 becomes 0.4667, 33 becomes 2.2, 45 becomes 3. Instead of 0-59, the loop is now 0-3.9333 in increments of 0.0667.
Then you just add one to make it 1-4.9333. spr() rounds down (e.g. spr(3.5) is the same as spr(3)), so it's effectively looping over 1-4 repeatedly. Since it counts up 1 each frame and loops at 60, the cycle takes 60 frames (which might be either one second or two).
Try using print() instead to see the values it produces.
function _init() t=1 end function _update() t+=1 print(1+t%60/30*2) end |
That works well enough, but I'd prefer something more straightforward, along the lines of
-- init tile=1 timer=0 -- update timer+=1 if timer==8 then timer=0 tile+=1 if tile==5 then tile=1 end end -- draw spr(tile, 60, 60) |



Thank you. That last example is more my speed. I need to get over feeling like I'm doing something wrong by using more tokens than necessary.
When I am trying to work things out there is this voice in my head that tells says "You idiot, if a real programmer saw that they would be disgusted and if you keep this up you'll run out of tolkens. Now feel ashamed of yourself"


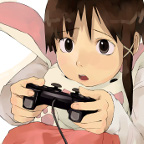
Token usage can certainly be an issue sometimes, but in general, a good programmer would favor simplicity and clarity over something "clever" and hard to understand. Of course, what's easy to understand does depend on experience to some degree, but no one's going to think less of you for not being an expert from the start.
Don't worry about tokens too much. If you do run out, you can always go back and change things. Making things more difficult when you have a good reason to do so is never a good idea.


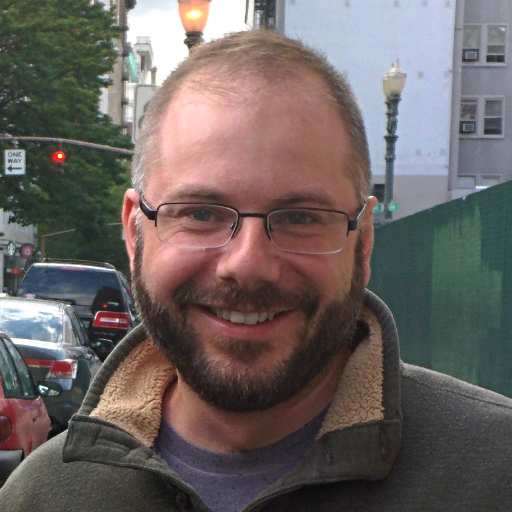
When I am trying to work things out there is this voice in my head that tells says "You idiot, if a real programmer saw that they would be disgusted and if you keep this up you'll run out of tolkens. Now feel ashamed of yourself" |
Please tell that voice to kindly bugger off. Of course writing clean, concise code is the goal, but if you saw the kind of dumb crap "real programmers" put in their code just because it works or because we just need to get on to the next thing, you'd feel so, so much better. 😉


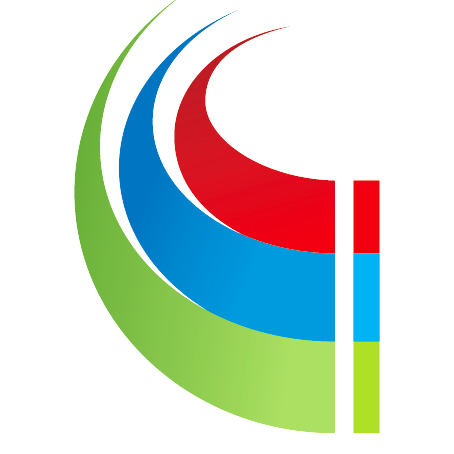
Oh god, what MBoffin just said in spades. Tell that voice to do one, for sure.
I've seen so much spectacularly stupid code, not all of which I wrote myself, that over time you realise that some things you get, some you don't, some were never meant to be 'got', and some things just need patience. :-)
I do use modulo in my code to loop around an array, which will contain sprite numbers for anim frames. But that code at the top is pretty impenetrable given what it's actually doing.
Neat, sure, but unless you're really up against a limit, it's not very helpful and so it's not a good thing to be trying to learn from. IMHO :-)



Definitely +1 to what MBoffin said.
Here's the other thing... if you start with something that you think is ugly or naïve, but works, then you literally have what's commonly called a "proof of concept". You don't have to leave that code written in stone.
Instead, you can:
-
Ponder it over time while you work on other things—coming back to something frequently lets you see obvious things you missed the last time around.
-
Look for similar work by other programmers and see what sorts of tweaks they made to improve theirs. I often learn new tricks by doing this.
- Show your code to other programmers—most of us love an opportunity to offer ideas/improvements where we've seen a code pattern before. It feels good to help pull other programmers up the rocky cliff of work experience.
I can only speak for myself, but when I show another programmer my code and they show me a way to improve it, I'm always very pleased. Sure, it would have been satisfying to get there myself, but either way, I've learned the trick for the next time I need it.
[Please log in to post a comment]