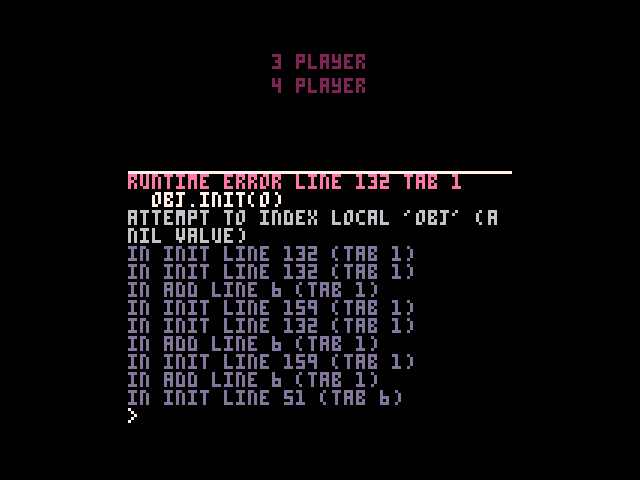
can someone help me?
pico8 is complaining it can't find the obj table on line 132.
it also is printing out weird traces
--objects tab local inst={} --obj manager function inst.add(o,...) o=copy(o) o.init(o,...) add(insts,o) return o end function inst.tick() for i=1, #objs do o=inst[i] o.tick(o) end end function inst.draw() for i=1, #objs do o=inst[i] o.draw(o) end end --obj base local obj = { init = function(o) --team o.team=0 o.weap={} --bars o.hp=100 o.mp=100 o.ap=3 --stats o.pwr=1 o.rng=1 o.rpd=1 o.nrg=1 o.spc=1 --anim o.a=true o.apos=0 o.aoff=0 o.askp=0 o.aspd=1 o.alen=1 --sprite o.s=true o.sobj=o --sync object o.spos=vec(0,0) --position o.soff=vec(0,0) --offset o.svel=vec(0,0) --velocity o.sfrc=vec(1,1) --friction o.smax=vec(4,4) --max speed o.ssiz=vec(1,1) --size needs fix o.srot=vec(1,0) --rotation --hitbox o.h=true o.hobj=nil --sync object o.hpos=vec(0,0) --pos o.hoff=vec(2,2) --offset o.hvel=vec(0,0) --velocity o.hfrc=vec(1,1) --friction o.hmax=vec(4,4) --max speed o.hsiz=vec(4,4) --size o.hrot=vec(1,0) --not implimented end, tick = function(o) --anim if a then o.aoff = wrap(o.aspd,0,o.alen) end --sprite if s then local pos=o.sobj and o.sobj.spos or o.spos local vel=o.sobj and o.sobj.svel or o.svel local frc=o.sobj and o.sobj.sfrc or o.sfrc local max=o.sobj and o.sobj.smax or o.smax local rot=o.sobj and o.sobj.srot or o.srot o.svel =vmull(vel, frc) o.spos =vaddl(pos, vel) o.svel.x= mid(vel.x,-max.x,max.x) o.svel.y= mid(vel.y,-max.y,max.y) o.spos.x=wrap(pos.x, 0, 127) o.spos.y=wrap(pos.y, 8, 120) end if h then local pos=o.hobj and o.hobj.spos or o.hpos local vel=o.hobj and o.hobj.svel or o.hvel local frc=o.hobj and o.hobj.sfrc or o.hfrc local max=o.hobj and o.hobj.smax or o.hmax local rot=o.hobj and o.hobj.srot or o.hrot o.hpos =vaddl(pos,vel) o.hvel =vmull(vel,frc) o.hvel.x= mid(vel.x,max.x,max.x) o.hvel.y= mid(vel.y,max.y,max.y) o.hpos.x=wrap(pos.x, 0, 127) o.hpos.y=wrap(pos.y, 8, 120) end end, draw = function(o) if s then sprr( anim(o.apos, o.aoff, o.askp), o.spos.x+ldirx(vgetr(o.srot))*o.soff.x, o.spos.y+ldiry(vgetr(o.srot))*o.soff.y, o.ssiz.x, o.ssiz.y, vgetr(o.srot) ) end end } --obj thrust local thr= { init=function(o,pos,rot,obj) obj.init(o) o.apos=32 o.spos=pos o.srot=rot o.sobj=obj o.soff=vec(0,-6) end, tick=function(o) obj.tick(o) end, draw=function(o) obj.draw(o) end } --obj player local plr = { init=function(o,pos,rot,team) obj.init(o) o.apos=32 o.spos=pos o.srot=rot o.team=team o.part1=inst.add(thr, pos,rot,o) end, tick=function(o) obj.tick(o) local u=btn(2,o.team) and 0.10 or 0 local d=btn(3,o.team) and 0.99 or 1 local l=btn(0,o.team) and 2 or 0 local r=btn(1,o.team) and 2 or 0 vaddl(o.svel, u, o.tr) vmull(o.svel, vec(d,d)) vaddr(o.srot, r-l) if u==0.10 then o.part1.alen=3 elseif d==0.99 then o.part1.alen=1 else o.part1.alen=2 end end, draw=function(o) obj.draw(o) end } |



it should only be calling the parent's init
so like add calls plr's init which calls add on thr which calls obj init
is there a better way to do this?



Option 1: fix the code (change init/add to avoid inifinite loops)
Option 2: start off clean and really understand pieces of code you probably gathered from some other post
Option 3: keep it simple. Do you really need nested init and such?
[Please log in to post a comment]