I am attempting to make the screen flash and shake. However, I would like it to be randomly generated. I vaguely researched the use of srand/rnd, but I ultimately do not know how to implement the proper code into what I currently have.
Warning: I have no idea what I'm doing.
player = {} player.x = 60 player.y = 60 player.sprite = 0 player.speed = 1 player.moving = false player.timer = 1 music(0) cam_x = 0 cam_y = 0 fliph = false flipv = false shake = 0 function _rand function _draw() cls() camera (cam_x,cam_y) cam_x = player.x-60 cam_y = player.y-60 map(0,0,0,0,16*8,16*2,0) --map(0,0,0,0,16,8) --map(0,0,0,64,16,8) spr (player.sprite, player.x, player.y,1,1,player.fliph,player.flipv) end function movex() player.moving = true player.sprite += 1 if player.sprite > 3 then player.sprite = 2 end end function movey() player.moving = true player.sprite += 1 if player.sprite > 5 then player.sprite = 4 end end function _update() player.moving = false if btn(0) then player.x -= player.speed movex() player.fliph = true elseif btn(1) then player.x += player.speed movex() player.fliph = false elseif btn(2) then player.y -= player.speed movey() player.flipv = true elseif btn(3) then player.y += player.speed movey() player.flipv = false end -- if not player.moving then --player.sprite = 0 -- end if not player.moving then player.timer = player.timer+1 end if player.timer >=30 then player.sprite = 1 end if player.timer >=60 then player.sprite = 0 player.timer = 0 end if player.moving then player.timer = 0 end end |


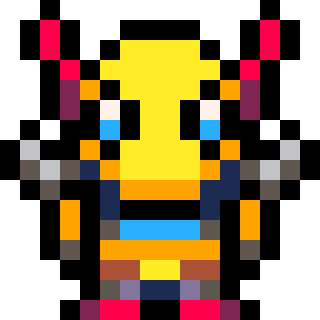
Okay so this is actually ~3 problems. The way you want to think when it comes to programming is that you're methodically breaking up big problems into smaller problems until you're at a level where you can get an idea of how to solve each thing step by step. And then you put it all together.
First, to get a chance of something happening, you can do something like this:
if rnd(100) <=10 then chance=true else chance=false end |
The way rnd() works is that it gets resolved to a number less than the number inside the parenthesis (and greater than 0.) This will usually not be a whole number (it will be 66.798 or something like that.) If you wanted it to be a whole number, you can do flr(rnd(number)) -- flr() stands for floor() and that strips off the numbers to the right of the decimal place so you're left with a whole number.
By doing 'if rnd(100) <= 10', we're saying "if this random number that's less than 100 is 10 or less, we're doing this". Or there's a ~10% chance of it being true.
Anyway, you'd want to check that every once in a while, and then show the flash effect if chance is true. To check 'every once in a while', you'll want to use a timer:
--first, setup a timer in your _init --if you're counting this down every frame, at 30 frames per second, the check will be run ever ~2 seconds timer=60 |
--then, whenever you're wanting to have the check done (say, if a 'raining' flag is set to true), lower the timer every frame --you do this by putting this in your _update() function: timer-=1 --then check to see if the timer has hit 0 if timer <= 0 then --if it has, then reset the timer and check set a flag to do your action timer=60 if rnd(100) <=10 then --the same as the 'chance' above, we're just giving it a more specific name do_flash=true else do_flash=false end end |
To have it 'flash' when your do_flash flag is set, you'd add something like this to your _draw function. You probably want to add it near the END so that it will cover up anything else that was drawn that frame:
if do_flash then rectfill(0,0,127,127,7) --reset the do_flash flag, or else it will blank the screen /every/ frame do_flash=false end |
Hope that helps!



This has helped very much! I appreciate you taking the time to respond so throughly. (I noticed that you had responded to my "Dead or Alive" post. :-P) I am an artist, so this is very new territory for me. This is how I am interpreting your instructions:
function _update() timer -= 1 if timer <= 0 then timer = 60 end if flr(rnd(100)) <= 10 then flash = true else flash = false end end function _draw() cls() if flash then rectfill (0,0,127,127,7) end end |
I wasn't certain of what to add to the global variables and the _init() function.
flash = false timer = 0 function _init() timer = 0 end |
As you mentioned, the screen keeps flashing. I'm trying to problem-solve my way towards resetting the timer. How can I make it so that the flashing randomly begins every few minutes? In essence, the flashes are random and the effect itself is also random.


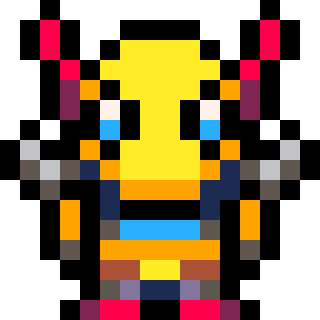
You want to change the part that you have like this:
function _update() timer -= 1 if timer <= 0 then timer = 60 end if flr(rnd(100)) <= 10 then flash = true else flash = false end end |
Right now, that's making it so there's a 10% chance every FRAME of creating a flash. There are 30 frames a second. So probably 3 times a second on average you're getting a flash.
So instead have it be like this:
function _update() timer -= 1 if timer <= 0 then --increasing this means you check every 15 seconds timer = 450 --450 is for (30 fps * 15 seconds) --You want the random (rnd()) check INSIDE the check for the timer running out --This makes it so you ONLY check your random chance when the timer runs out if flr(rnd(100)) <= 10 then flash = true else flash = false end end |



How would one code the random check inside the timer check? Whenever I try to switch things around it stops functioning.


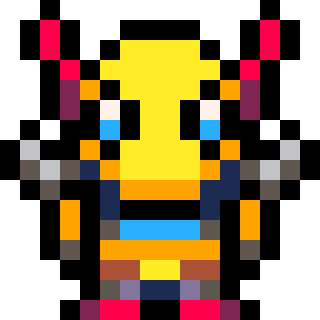
Yeah, it can be hard to tell where it all should go when it's sort of broken up out on the forum. Here, I updated your cart to show what I'm trying to describe.
Check out the new variables (flash_chance and flash_check_frequency) -- there's some notes that explain what they're doing. Hopefully seeing it in action will make things more clear. But the basic idea is that the frequency is how often you check. And the chance is what % of 100 there is for it make a flash when you check. You may have to mess with those values a bit before you find what works best for you. But just try the defaults I put in (40% chance to flash once every 5 seconds) and see what you think.
Btw, I really like the music you put in there! Keep at it, this looks like it will be a fun game.



Thank you! It works perfectly. :-)
As if I couldn't ask more questions... What would be the best route to set up a timer for the flashes? So, the flashes would occur within a frame of one minute. And then have that event of minute-flashing be a randomized occurrence?


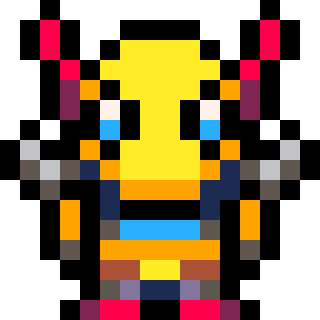
Well you just have to take what you've done so far and plan it out a little to get it tuned the way you would like...
- We've made a timer that counts down every frame
- NOTE: It's to know that a 'FRAME' here is referring to a single 'pass' over your _update and _draw functions. This happens ~30 times per second.
- We've made logic that runs a check once the timer hits zero
- We've made logic so that, if that check is a certain value, an event happens (i.e., the lightning 'flashes')
- We've made logic that 'resets' the timer to a default value once it hits zero
From your description it sounds like you want to implement 'weather', right? At random times, a storm will last a minute, during which there will be occasional flashing. To do this, you just want to break down what you want to happen into simpler steps and rules:
- At random times, we want a 'storm' to happen
- We only want the storm to last 60 seconds (60 seconds * 30 frames per second --> 1800
- After the storm is done, everything should go back to normal (until another random storm happens)
And then you want to see what you know how to do, and use/extend those things to fit those rules:
- At random times, we want a 'storm' to happen [Set a timer, and do a check to see if an event should occur. If the check passes, then set a flag so that certain behavior happens. NOTE: When that flag is already set, we also want to make sure we do NOT run this random check]
- We only want the storm to last 60 seconds (60 seconds * 30 frames per second --> 1800 [If the check passes, we want to track a NEW timer. This will last 1800 frames so that once it hits zero, the storm will have gone on for ~60 seconds]
- After the storm is done, everything should go back to normal (until another random storm happens) [Once that storm timer hits 0, we turn off the 'make random flashes' flag. This turns that behavior off, so that the storm stops.
Also, at this time we reset the timer for the random check and start counting it down/doing the random check when its timer hits zero to see if a new storm should start.]
Basically you're taking what you've already done, and sort of putting /that/ behavior inside of its own timer with its own flag.
Hopefully that makes sense. Learning to think this way -- breaking up problems logically and methodically into rules and then figuring out how to use patterns to implement those rules/behaviors using code -- is programming. Don't be frustrated if you don't get it all at once. It's a cumulative skill, so it's normal to feel overwhelmed at first. But as you solve problems you're going to have more resources in your mental toolkit to solve future problems.



Just wanted to give some props to @enargy ! These are awesome explanations of not just how to do the code, but how to design a program. I'm a programmer by accident, I really wanted to be an artist. You can actually use the same kind of process in art, building a vision by working on the little parts of it one at a time. Same with code. You're trying to represent a honey bees world within a very limited form: a grid of pixels that can change 30 times per second. So far it looks great, but when you get to the code part too, exactly what enargy has been saying... Break the problems down into parts and write that code. How you compose it is important too, but if you have created your data model thoughtfully, it should reveal how to compose the program
[Please log in to post a comment]