Hello,
I'm a noob at programming and need some help please.
I'm try to write a for next loop that randomly fills an 2D array (my game grid), but I also want it to check that if that cell already has been used, then knock the counter back -1 and tries again. So in this way, at the end of the loop there should be a set number of cells filled.
My code works great except that when it detaches a duplicate cell, it doesn't roll the counter back.
So say at loop count 50, there's a duplicate cell, it doesn't alter the cell (which is correct), but it doesn't roll the loop counter back, it just continues.
Here's my code. I was hoping that the i-=1 would do the trick, but it doesn't.
function make_grid()
for i=1,level*100+1 do
y=flr(rnd(gridheight-6))
x=flr(rnd(gridwidth-6))
--is the space empty? then add
--a bomb. if not rollback counter
if grid[1][y+3][x+1]==0 then
grid[1][y+3][x+1]=2
else
i-=1
end
end
end
I've had print statements in there print the value of I as it went along, but the I-=1 doesn't effect the loop counter I
Any ideas and thanks in advance.


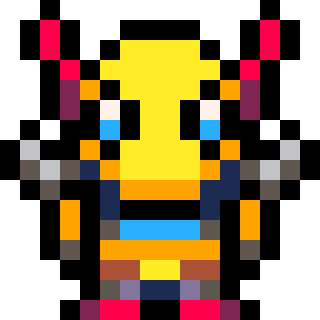
Try doing a while loop instead:
function make_grid() bombcount=0 while bombcount<6 do y=flr(rnd(gridheight-6)) x=flr(rnd(gridwidth-6)) --is the space empty? then add --a bomb. if not rollback counter if grid[1][y+3][x+1]==0 then grid[1][y+3][x+1]=2 bombcount+=1 end end end |



As an aside, this feature of lua, where the loop counter is basically internal and you're only given a copy of it on each iteration and which you can mangle all you like, is quite handy. It can be especially nice when mixing 0-based stuff with 1-based stuff, e.g.:
_all_chars = "\x00\x01\x02 ... \xfe\xff" _char_to_int = {} _int_to_char = {} for i=1,#_all_chars do local c = sub(_all_chars, i, i) i -= 1 _char_to_int[c] = i _int_to_char[i] = c end |
Notice I start each iteration with the 1-based index into the character set string, but finish it with a proper 0-based byte/character value. In C/C++ this would make the loop infinite, but in Lua, it works fine.
[Please log in to post a comment]