What am I doing wrong? I'm sure I'm being dumb.
I can go down ladders fine, but not up them.
Cartridge
Code
function _init() p={x=0,y=0,spr=0} end function _update() if(btn(0)) then p.x-=1 -- left end if(btn(1)) then p.x+=1 -- right end -- ladder down if (btn(3) and fget(below)==2) then p.y+=1 end -- ladder above [b]if (btn(2) and fget(above)==2) then[/b] p.y-=1 end end function _draw() cls() map(0,0,0,0,6,6) spr(p.spr,p.x,p.y,1,1) below=fget(mget(flr(p.x/8),flr(p.y/8+1))) [b]above=fget(mget(flr(p.x/8),flr(p.y/8-1)))[/b] behind=fget(mget(flr(p.x/8),flr(p.y/8))) print ("behind "..fget(behind),90,100) print ("below "..fget(below),90,110) print ("above "..fget(above),90,120) end |
Sprites & Flags
Sprite #0 - Flag #0 - Player
Sprite #1 - Flag #1 - Platform
Sprite #2 - Flag #2 - Ladder
Any help would be gratefully appreciated!
(Note: I have a larger version in which collision and falling works,
but stripped this down to the bare bones to try to solve this issue)


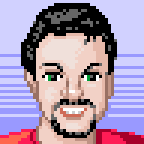
Once you move one pixel up the ladder, "above" will start referring to the block two tiles above the player - flr(p.y/8-1) will change value immediately.
What you probably want is allow ladder movement if any part of your player touches a ladder, or there is a ladder one pixel under him (standing "on" a ladder).
If you imagine the player as an 8x8 box, you have to check all tiles intersecting this box for "ladderness". If you make the box 8x9 pixels, this will take care of standing atop a ladder, too. Since tiles are 8x8, we only have to check the corners to make sure we've hit all relevant tiles.
Something like this should work:
function tile_at(x, y) return mget(flr(x/8),flr(y/8)) end function is_ladder(tile) return tile == 2 end local player_touches_ladder = is_ladder(tile_at(p.x, p.y)) or is_ladder(tile_at(p.x+7,p.y)) or is_ladder(tile_at(p.x, p.y+8)) or is_ladder(tile_at(p.x+7,p.y+8)) |
Only the corners of the box have to be checked here - in a more general case, I suggest reading up on simple collision detection (bounding boxes etc.).
Hope that helps :)



Thank you so much Krajzek! I used your example and it works much better than my attempt.
It still needs to be tinkered with some more, but I'm sharing it here for anyone interested.
function _init() p={x=0,y=0,spr=0} end function _update() if(btn(0)) then p.x-=1 -- left end if(btn(1)) then p.x+=1 -- right end -- ladder down [b] if (btn(3) and ladder_below) then p.y+=1[/b] end -- ladder above [b] if (btn(2) and ladder_above) then p.y-=1[/b] end end function _draw() cls() map(0,0,0,0,6,6) spr(p.spr,p.x,p.y,1,1) [b]ladder_below = tile_at( p.x, p.y+8 )==2[/b] [b]ladder_above = tile_at( p.x, p.y+7 )==2[/b] end function tile_at(x,y) return fget(mget(flr(x/8),flr(y/8))) end |
[Please log in to post a comment]