So maybe this is old news to everybody but me but I just discovered it by accident. Turns out you can define local functions! It makes sense but it somehow never occurred to me to try before.
Here's a quick example:
do local function a() print('in a') end function b() print('in b: calling a') a() end print('in local scope') print(a) print(b) a() b() print('leaving local scope\n\n') end print('in global scope') print(a) print(b) b() |
Output:
in local scope [function] [function] in a in b: calling a in a leaving local scope in global scope [nil] [function] in b: calling a in a |
That's it. Just thought it was interesting.


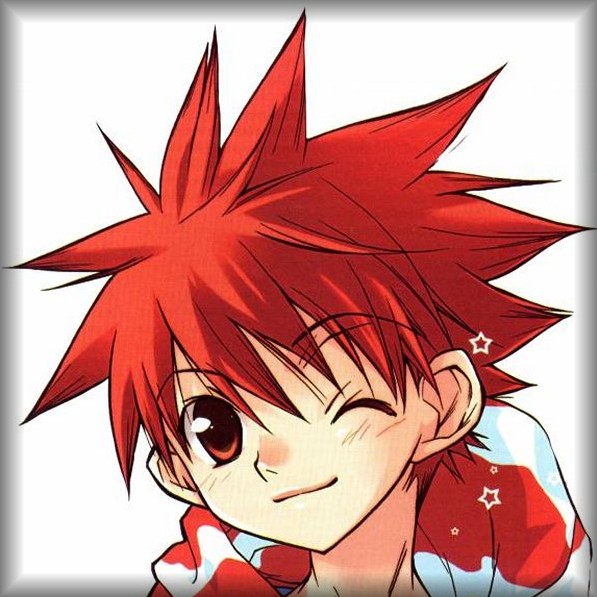
I'm - err - not entirely understanding what you are doing here.
I have a question regarding this maybe.
Can you do this ?
local function addup(a) b=a+1 return b end |
Where by defining a local function all variables defined inside are also local, such as b
?



no, all variables are global by default. You must use the "local"-keyword for local variables.
btw.
function name (para) |
is an alternate form of
name = function (para) |
"Functions" are in lua a value like numbers, strings, tables...
edit:
also important, variables defined by "for" are local and only available in the for-end-loop.
a="outside" for a=1,2 do print(a) end print(a) |
will output:
1
2
outside


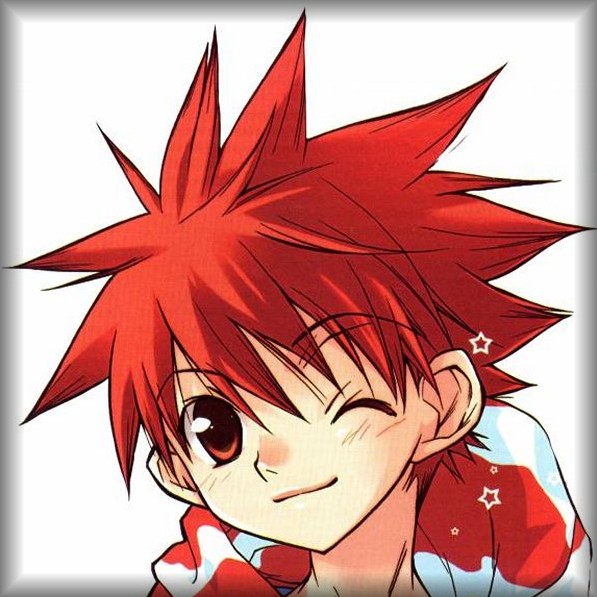
Yep. I knew about that last one but only recently, @GPI. My brain can't really wrap around that so instead I use defined local variables if I need something inside a For/End
loop.



To be precise, the translation of this:
local function f() --contents end |
is this:
local f; f=function() --contents end |
The separate declaration is important for ensuring recursion works properly. I don't know for sure, but I suspect that's one of the more common uses of local functions.
Also, in case you haven't thought of it as well, I'll throw in that functions can also be passed as parameters too.



No you would still need to declare b as a local variable for it to be local to the function. So as you've written it b
will still end up being a global variable.
One thing local functions potentially give you if you want to go to the trouble—and you may not want to—is a little bit of encapsulation.
For example:
do local function helper() -- this is useful to one or more functions inside the do...end -- block but probably shouldn't be called from anywhere else. end function useful_function() -- does some stuff that you'll want to be able to do in other -- parts of your code. But it needs some help. helper() -- and then maybe does some other stuff end function other_useful_function() -- this might do something else but also need help. helper() -- and then do other stuff end end -- now we're outside the do..end block so: useful_function() -- this can be called from anywhere in your code other_useful_function() -- so can this helper() -- but this will cause the program to crash because it -- doesn't exist outside of the do..end block |
The point being: for whatever reason helper() shouldn't be called from just anywhere or it might cause an error (mess up some game state or whatever.) Defining it as a local function inside a do..end block means that we can't call it from anywhere except inside that block so we avoid any potential errors. It's sort of similar to having private methods on objects in Java, for instance: usable within the class but not usable outside of it.
It's by no means necessary to do this but every error that you make impossible (for a suitable definition of impossible) is one less bug you have to hunt down later.
[Please log in to post a comment]