Hi I'm currently working on my first project in pico-8 and I loosely followed a tutorial on collision detection and I wrote this function
function colliderect(x1,y1,w1,h1,x2,y2,w2,h2) xs = w1/2 + w2/2 ys = h1/2 + h2/2 xd = sqrt((x1)^2+(x2)^2) yd = sqrt((y1)^2+(y2)^2) if ((xs > xd and ys > yd)) then return false //colliding else return true //not colliding end end |
which takes the x and y coordinates and the width and height of two objects and calculates if they collide. The function itself seems to work as it should and gives the correct result when manually inputting the data but when I implement it like this
function update_player() for i in all(bullets) do if (not colliderect(player.x,player.y,player.w,player.h,i.x,i.y,i.w,i.h)) then sfx(1) end end end |
it doesn't work anymore. Even if I am standing right on the bullet it doesn't play the sound it should when colliding (and yes I did check if it's the right SFX). I am also not getting any errors when running the program so I don't really know what's wrong. Any help would be very much appreciated.
Figured out what's wrong already I had a small mistake when calculating xd and yd that is I forgot to add the width and height to them when calculating them. This is how it should have been.
xd = abs((x1+(w1/2))-(x2+(w2/2))) //also learned that an abs function exists for pico yd = abs((y1+(h1/2))-(y2+(h2/2))) |


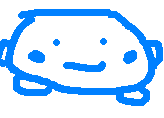
I think you can do rectangle collisions with a few comparisons, without sqrt or exponent which can be expensive for pico8 and sometimes risk overflow too. for example: https://mboffin.itch.io/pico8-overlap


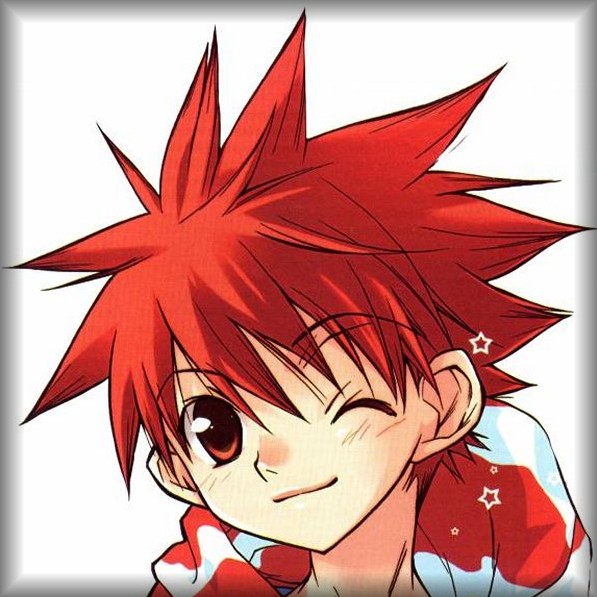
@merwok is correct, @Ben0981. You do not need sqrt()
or ^
to determine collision.
Here is a simple example:

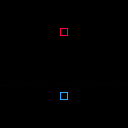
Click on the Code ▼
beneath the frame above to see the source-code and the collision()
function.
In the demo above use the arrow keys to move and the 🅾️ key to swap between moving the red or blue square.
A collision will register if they are touching.
If you need more advanced collision detection by pixel for instance, I did this some years back found HERE:
[Please log in to post a comment]