So I'm getting ready to make a new game, in which the player will be able to push other objects around in an AOE.
I have a table, ACTORS, which will hold all my actors; each one will have x/y coordinates.
So, to get everything within radius R of point X, Y:
function get_near(x,y,r) for i in all(actors) do --use some math to get the hypotenuse vx=i.x-x --get the distance on X vy=i.y-y --same for Y --SOME TRIG GOES HERE --Sorry, still a little fuzzy on my trig if(result<r)then add(list,i) end end return list end |
Which would return a list containing...what, exactly? This is where I run into the problem.
If adding an item from one list to another copies the original to the new list, then I get a list of actors that's basically useless--I can't push them around, because if I do anything to list, it won't affect actors.
However, if adding an item from one list to another creates a pointer to the first list, then I'll be able to use this to conveniently push stuff within a certain distance.



In general, you can use the rule - if there are braces, you are creating a new object. If there are no braces, you are not creating a new object.
So in this case, since there are no braces, your table ("list") contains actors that are aliased - they're also accessible via the "actors" variable.
So yes, they act like "pointers", but the difference is you don't need special dereference operations, and generally the situation is symmetric between "list" and "actors".
Does that help?


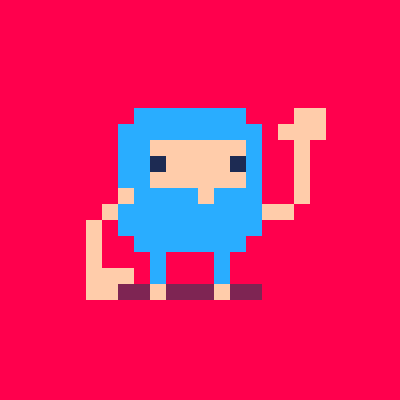
The list is a list of references (like pointers), yes. So get_near() looks like it will work fine.
Not much to add to Johnicholas's reply, but here's the trig I think you want:
if (vx*vx + vy*vy < rad*rad) then -- is inside radius end |
Note that as numbers only range from -32768 .. 32767, this will only work if vx, vy and rad are all less than 180!
An example demonstrating that table variables in Lua are just references:
a = {} -- a is itself just a reference to this newly created table object a.x = 5 a.y = 7 b = a -- a and b now refer to the same thing b.x = 6 print(a.x) --> 6 |


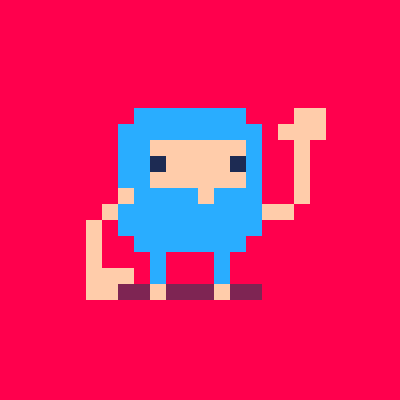
Oh, and don't forget to make the list table at the start of the function:
function get_near(x,y,r) list = {} ... end |


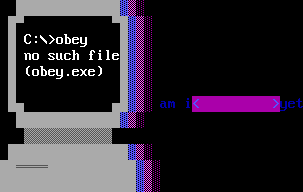
Zep said: Note that as numbers only range from -32768 .. 32767, this will only work if vx, vy and rad are all less than 180! |
Shouldn't be a problem, since the screen is only 128 pixels wide.
Thanks for the help!
[Please log in to post a comment]