Hi, I'm new to the scene here- I'm just writing a text adventure game and I'm looking for a way to scroll the text or just get the player to the "next page" of text? The map I load into the game is only a drawn border or fancy frames for the game... This is the code I have as of now, but the program skips to the last update function when I press the 'X' button/key in the game is the problem I currently am faced with. I also have yet to figure out an inventory system or anything similar. I know my code isn't clean- nor is it efficient, but I'm just looking to do a text adventure. Any help is appreciated however!
-- main
cls()
-- prints map
map()
-- beginning
print("my story text here",15,20)
print("my story text here")
print("\n")
print(" (hit x to continue)")
print(" other buttons displayed here",20,100)
print(" ")
-- button pressed
function _update()
if (btn(X)) then
-- scroll text
cls()
-- prints screen
map()
-- beginning
print("my story text here",15,20)
print("my story text here")
print("\n")
print(" (hit x to continue)")
print(" other buttons displayed here",20,100)
print(" ")
end
end
function _update()
if (btn(X)) then
-- scroll text
cls()
-- prints screen
map()
-- beginning
print("my story text here",15,20)
print("(hit the < key to (do "something")")
print("(hit the > to (do "something")")
print("️ other buttons displayed here",20,100)
print("my story text here")
end
end



You can only have one _update() function. When you define more than one, the later definition replaces the previous one. This program runs as if the first _update() definition isn't there.
You will want to think about how to keep track of the current state of the game in variables and data structures. For example, you're attempting to describe two possible scenes, each with different behavior. Your single _update() function will need to keep track of which scene to display in a variable, then use conditional logic based on that variable to decide how to display that scene and listen for appropriate input.
Your final game will have many possible states captured in variables and data structures, with tons of conditional logic. You will want to think of ways to represent these things efficiently. For example, instead of two functions with print statements, you could use a table of scene descriptions, and a variable storing an index into that table. There are many interesting ways to do this! Experiment and find out.
Keep in mind the call flow of the game loop: PICO-8 calls _init() once when the cart is run, then calls _update() and _draw() 30 times per second. What will those functions have to do to support an adventure game?
Feel free to ask more questions. You might also enjoy joining the PICO-8 Discord. (See the Resources page.) Good luck!


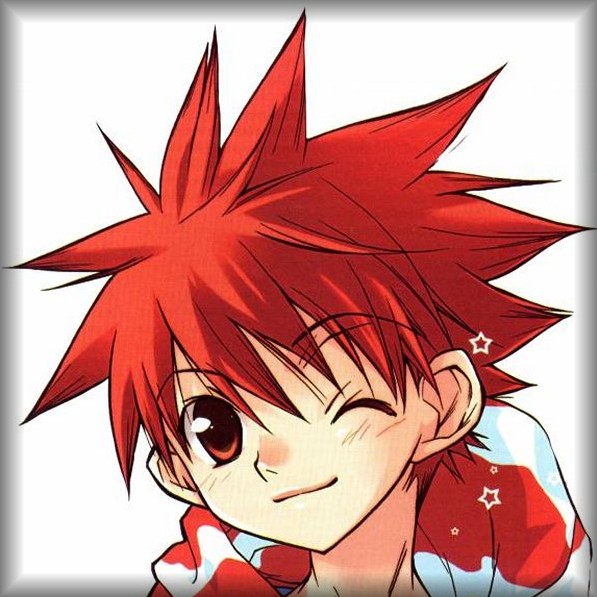
You might be interested in learning from the master, Scott Adams, @erayers. He used VERB NOUN in his input selections and wrote a great many text adventures for the Apple ][ computer.
CHOP TREE, GO EAST, USE SWORD, GO STUMP, LIGHT MATCH, etc.
You can also use shortcuts like single keypresses followed by ENTER. N, S, E, W, U, D.
https://en.wikipedia.org/wiki/List_of_Scott_Adams_Adventure_video_games
You can play one of his most famous text adventures HERE:
https://www.ifiction.org/games/playz.php?cat=44&game=150&mode=html
Click in the WHITE FIELD to type followed by ENTER, just below the GREEN >_
To start in this game, try:
CLIMB TREE, D, E, E, GET AXE.
Also type HELP followed by ENTER to get help.
If you can develop a game with a format like this for Pico-8 I imagine it will receive a very warm reception, including myself. Good luck !


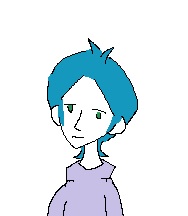
update
I now have it as something like this:
function _init() --stores txt & formatting story={{txt="1st page",fmt=",15,20,14"},{txt="2nd page",fmt=",15,20,14"},{txt="3rd page",fmt=",15,20,13"}} counter=1 end function _update() --increments story page counter by +1 if (btnp(X)) counter+=1 end function _draw() --clears the screen cls() --if statement to print output of stored txt if counter >= count(story) then counter = count(story) print(story[counter][txt],story[counter][fmt]) print(counter) map() else print(story[counter][txt],story[counter][fmt]) print(counter) count(story) map() end end |
returns no errors but doesn't print anything in the form of text as that output now seems to be empty
except that map() still works and outputs onto the screen


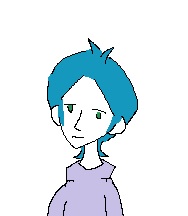
I've taken a look at their game and it Reminds me of a MUD! dw817 (https://en.wikipedia.org/wiki/MUD)
Thank you both! @dddaaannn @dw817


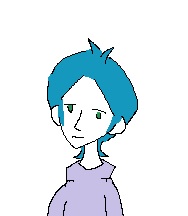
@dw817 Richard Bartle is also notable when discussing text adventure games!
(https://en.wikipedia.org/wiki/Richard_Bartle)



You're close!
The tbl={txt="some text"} syntax creates a table entry with a string key. To refer to this entry, use a quoted string value as the index: tbl["txt"]
There actually is a way to do what you're trying to do with the print formatting parameters, though it's a fancier feature of Lua that might be difficult to understand. Instead of a string like ",15,20,14", make that value a table: {15,20,14}. Then in the print() function call you can use unpack(...) to convert the table to parameters.
This does what you're trying to do:
function _init() story={ {txt="1st page",fmt={15,20,14}}, {txt="2nd page",fmt={15,20,14}}, {txt="3rd page",fmt={15,20,13}} } scene = 1 end function _update() if (btnp(➡️)) scene += 1 if (btnp(⬅️)) scene -= 1 if (scene > #story) scene = 1 if (scene < 1) scene = #story end function _draw() cls() print(story[scene]["txt"], unpack(story[scene]["fmt"])) end |


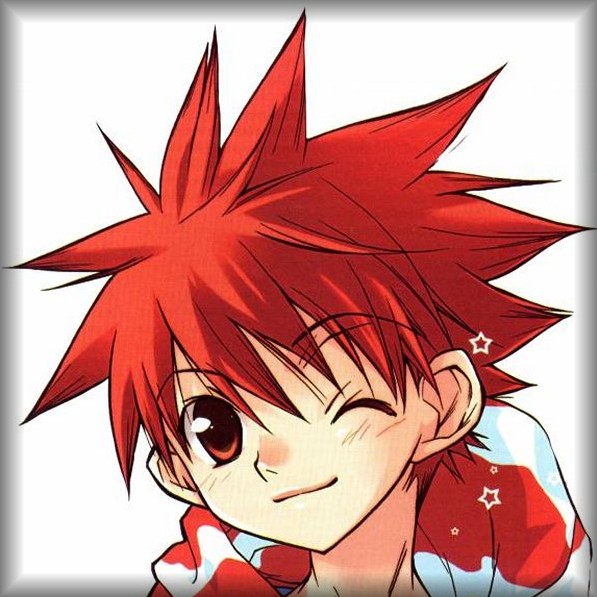
Well now MUD is nothing like old Apple ][ adventure games, @erayers. MUD stands for Multi-User-Dungeons. Remember back then there weren't even any BBSs, not to begin with - and certainly no internet. No, Scott Adams' adventures were for one-player, solo exploration. Originally read off of audiocassette and text only.
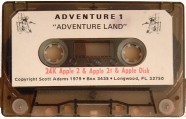
Here, let me see if I can put together some code. It doesn't do much but does separate verb from noun and is written using the conventional notation of _INIT() and _UPDATE().

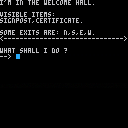
In the code variable TRUN has a value of 4. This means when you type in a VERB and NOUN for the input, it will truncate it to 4-characters. So "Examine" becomes "Exam" and "Certificate" becomes "Cert."
The other arrays are:
1. ROOM{} ... Contains room descriptions. 2. ITEM{} ... All items in room separate by comma "," 3. EXIT{} ... The visual exits to the room separated by comma "," 4. DOOR{} ... The actual ROOM # to go to - separated by comma "," roomno= room number to start in |
I have disabled the P and ENTER key for pausing, you can use them for regular typing and added PAUSE to appear when you hit the TAB key.
So try it out. See what you think.


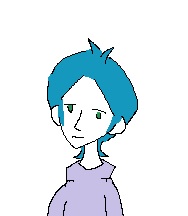
wait
if (btnp(➡️)) then map(02) |
(or second map?)
is there a way I can do something like this
plus where do I store different maps- sorry I'm still fairly fresh to Pico



@erayers What are you trying to accomplish by calling map()? Are you drawing a picture on the screen in addition to your text?
map() is a way of drawing a large image on the screen. You draw 8 pixel x 8 pixel tiles called "sprites" in the sprite editor (the 2nd icon in the upper right of the editor), then paste those sprites into the 128 x 64 cell "map" with the map editor (the 3rd icon). In your program, you call the map() function to draw a portion of the map onto the screen, giving it the cell coordinates of the upper left tile and the screen pixel coordinates where you want it drawn.
For an example of how map() is used in a full game, see the Jelpi demo game included with PICO-8. (Type "install_demos" at the command prompt to install Jelpi and other demo carts, then "cd demos" then "load jelpi".)
You might want to try some of the introductory video tutorials and read through the manual. The manual explains all of the functions, and the wiki goes into more detail. See the Resources page.


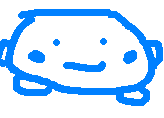
The problem with
if (btnp(➡️)) then map(16,16,0,0,16,16) |
is that it means: draw the second screen of map data only for the frame where button was released. In other words: the 'if' condition will be false for many seconds, then true for 1/30rd of a second (one frame), then immediately false again.
This is why we have _update and _draw. In _update, you check the buttons and update some variables (to know how many lines of text to print, to move the player, to see if we are on map 1 or map 2). In _draw, you use these variables to decide what map and sprites to draw.
[Please log in to post a comment]