Beginner here, so forgive me if I'm missing something obvious. I'm currently working on a simple clone of Twin Bee to learn a little bit about design. I'm running into a blockade due to my lack of programming experience, so I'm hoping someone may have some answers from me.
I've got clouds that fall from the top of the screen. The function that sets all the variables for the cloud table looks like this:
function spawncloud() local c = { x = rnd(95)+16, y = -16, dy = rnd(.5)+.75, sp = 5, hb = {x1=0,y1=0,x2=13,y2=1} } -- bell properties - is it necessary for me to declare -- these properties within the spawncloud function -- because the bell position depends on the cloud position? [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=64663#p) |
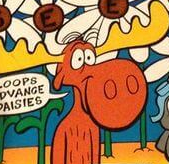

