Thought I would share some code I've been using for my pico-8 game. It's a simple class library that also includes inheritance.
139 Tokens
617 Characters without comments
How to Use:
Create a new instance with function "new".
The first variable is always the table that holds all the variables, 2nd and onwards are used for the function "__init('table','...')"
Example:
player:new({variablea=1,variableb=2},initarg1,initarg2) |
Variables are copied over just fine, however for tables if you don't want each instance to share the exact same table with each other then I would recommend defining them in the function "__init('table','...')".
Example:
--do this function newclass:__init(varc,vard) self.vartable={varc,vard} self.varemptytable={} end --if you don't do that then instance1.varemptytable==instance2.varemptytable |
When creating a class, you can inherit from other classes upon it's creation by simply including classes after the first argument.
Example:
newclass=cwi:new({vara=3,varb=4},classtoinheritfroma,classtoinheritfromb) |
You can also inherit from other classes by running function "inherit('...')".
Classes have an "init" function that is run every time a new instance is created.
Classes that have been inherited from will NOT run their function "init('...')" upon creation of a new instance.
Function "oftype('class')" allows you to check each if one class is of the type of another.
Classes that have been inherited will also return true for function "oftype('class')".
Finally I present the code:
--Classes With Inheritance! cwi={} cwi.__index=cwi function cwi:__init(...) self.__types={} if #{...}>0 then self:inherit(...) end self.__init=function() end --create an empty function so all classes function "__init" doesn't inherit end function cwi:new(r,...) r=r or {} r.__index=r setmetatable(r,self) if r.__init then r:__init(...) end return r end function cwi:inherit(...) local function c(a,b) for k,l in pairs(b) do if not a [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=70752#p) |
I've been trying to use the new (although not that new now) #include command but I can't seem to get it working.
Code on pico-8 cart
#include testfile.lua test() |
Code on testfile.lua
function test() print("test",100,0,8) end |
When I run I get the error:
testfile.lua line 3 syntax error line 1 (tab 0) endnd syntax error near `test` |
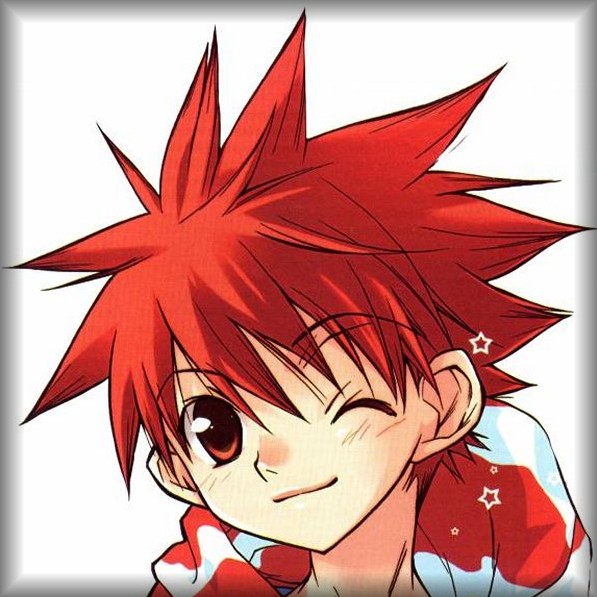