id=stat(31) identifies keypress_q as id="q" and keypress_a as id="a". Btn(5,1) identifies itself as keypress_q and keypress_a. How do I separate keypress_q to work via id=stat(31)="q" and btn(5,1) to work 'only' with keypress_a?
id=stat(31) if btn(5,1) and id~="q" then... --input for keypress_a if id=="q" then... --input for keypress_q |
That 'would' work...but the problem is stat(31) works like btnp() when I need it to work like btn()...but the poke methods of making btnp() work like btn() don't seem to apply to stat(31)! So I'm presently stuck in a situation where I can be pressing keypress_q and yet id~="q"! The obvious way around this is to just use id=="a" for keypress_a; id=="q" for keypress_q....but again, they work like btnp, so this doesn't work if I want to use keypress_a for standard smooth wasd player movement.
The intent is to basically utilize the existing buttons of player 0 and player 1....and add the functionality of stat(31) to get even more keys. I don't necessarily need the other keys to have the same functionality as btn(), they can just be 'single tap' style keys...I'll use them as like rpg skills that go on cooldown right when they are used. Player movement via wasd though needs the functionality of btn(). But again, the problem is the overlap....specifically with those btn(4/5) keys since they have more than 1 natural input (seen below), when I want to choose just 1 natural input and use stat(31) to utilize the other.
For the sake of reminder I'll include the keys below to give an idea of the overlap, specifically btn(4/5):
P0: 0left;1right;2up;3down;4zcn;5xvm p1: 0s/1f/2e/3d/4wtabshift/5aq Chosen usage for p1: a:btn(5,1)|d:btn(3,1)|w:btn(4,1)|s:btn(0,1)|f:btn(1,1)|e:btn(2,1) |
I guess p1:btn(5) is the only case of overlap...since I can't seem to get stat(31) to work for tab/shift/backspace...mainly I don't get anything showing up when I printh(id) with those inputs. I get "9" for backspace...but only keypress_9 activates the 'if id=="9"' case. stat(31) is also sort of weird...not sure if buggy is the right word. Is it single thread or something? If you 'also' print(stat(31)) to the screen it negatively affects id=stat(31), which won't work as reliably....I think stat(31) can only be called directly once per frame? Or something to that effect



First off, you're using stat(31)
wrong. If there's more than one key pressed in the same frame, things will get weird. You have to poll each frame for multiple keys via stat(30)
, which will be true until you've seen every key pressed that frame via stat(31).
abtn=false function _getinput() local c while stat(30) do c=stat(31) --make sure to store in a variable, since this changes each time you call stat(31) if(c=="a") abtn=true --'a' key was just pressed end if(abtn) abtn=btn(5,1) --'a' (or 'q') is still being held down right now. this almost works. end |
Second, there's an issue using WASD with controllers/html exports, even via btn(...,1) calls. When I suggested using these hacky second-player codes for WASD support in POOM, freds72 found that W (or A, not sure) doesn't get detected properly in html/bbs exports when held down.
You may be able to use the newer stat(28)
raw key interface instead of btn(), to get SDL keycodes, like this:
--if btn(5,1) then --'a' (or 'q') btn check if stat(28,4) then --'a' only btn check if stat(28,20) then --'q' only btn check if stat(28,26) then --'w' only btn check |
However, this will be bad for AZERTY keyboards, where the Q key is in the place A is on your QWERTY keyboard. I'd recommend doing if stat(28,4) or stat(28,20) then
specifically to mimic the btn(5,1)
functionality of testing for both, and hope that method was more export friendly. Why do you want to get rid of the Q option?


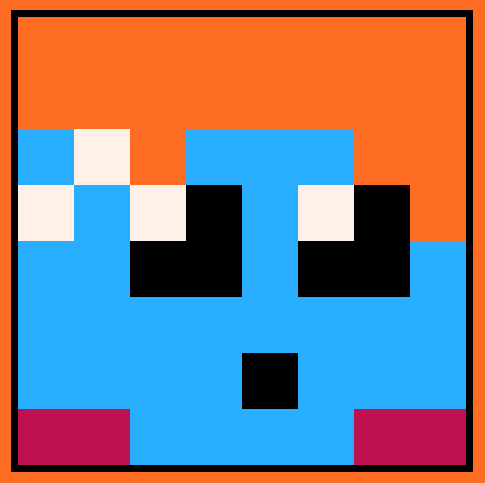
poke(0x5f2d,1) function _update() local c ybtn=false ubtn=false while stat(30) do c=stat(31) --make sure to store in a variable, since this changes each time you call stat(31) if(c=="y") ybtn=true --'y' key was just pressed if(c=="u") ubtn=true --'u' key was just pressed end --abtn=btn(5,1) --'a' (or 'q') is still being held down right now. this almost works. end function _draw()cls() printh("y:"..tostr(ybtn).."u:"..tostr(ubtn)) end |
This doesn't work as stated though. I changed the keys to y and u cause that commented out line of using btn was defeating the point. With your code Only y OR u can be true on any given frame...not both...u can see that by holding both at the same time. My understanding is your point in using stat(30) was to allow 'for both' to register in 1 frame.
The usage of stat(28) instead of stat(31) seems promising. Is stat(28) how btn() was derived (more or less anyways) to begin with? Would it make sense then to forget the usage of btn() and just use stat(28) for all keyboard inputs? not rly much in the wiki about it



The top code was more to demo how you should use stat(31) than a solution to your problem. It only works via the use of btn(5,1) in the lower if statement to detect a "release" event, so arbitrary keycodes won't work there at all.
Yep, stat(28) is what you need if you want direct keyboard access (but doesn't work with controllers like btn() does). Just remember that different configurations of keyboards exist, so if this is for movement you should account for QZERTY/AZERTY.
Edit: Your code is setting ybtn/ubtn to false every frame, inside the update function, otherwise they would both stay on forever. I'm confused, are you trying to show that only one or the other key can be pressed per frame? You might have keyboard ghosting which prevents this, but I can definitely get both keys to show up on the same frame on my computer, specifically by pressing (not holding) both keys on the same frame. I'm unsure what your point was here.


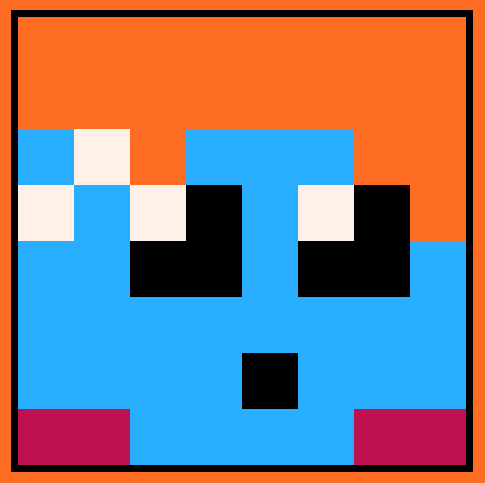
My code is explicit. Run it with a command console and check the printh()...u cannot get both statements to be true on the same frame. I frankly don't believe you did this.
You were showing me how to use stat(31) cause the method in my OP was "wrong" you said. Yet ur method assumes stat(31) is able to send multiple keys on any given frame....which maybe it is! I dont know, but the code u presented doesn't do anything of the sort. And to get it to look smooth like btn()....which it doesnt do!...u used btn()...which just defies the point of even using stat(31) to begin with!!!...just use btn! (But if I said it that way you'd just neglect what we were talking about to begin with so I changed the keys to those that weren't covered by btn and asked for your counterargument.) So you'll forgive 'my' confusion of what 'your' point was...cause I was not wrong, you were wrong. Notice how, unlike you, I didn't say that at the time though. Why? Cause maybe someone will come along and prove me wrong...! GOOD...its good to be wrong so long as you are trying to develop the solution...cause u get to be more right than ever in the end.
And You don't understand something? Ask me to rephrase it next time! Like ur first comment u said some stuff that I literally said in my OP at least 3 times already so I didn't even bother to address it...cause I would just be quoting myself 3 ways and u didn't seem to be able or interested in answering that part anyways. So instead I ignored it and focused on what you did present...and somehow that led to the same outcome, hilarious huh. If u asked for a rephrase tho...that would be valid! I would do that. Instead I made the calculated decision to salvage what I could from your responses, this was intentional, it was not meant to make it appear I somehow didn't read the other parts and u need to repeat them again.
Which you did anyways, especially this semblance of how to use pico8 on me...I don't care! It has nothing to do with my OP. It's fine if u want to say it once as a sidenote, power to that..fine, whatever. But u keep repeating it...why?!?! Stop! Take note how I didn't respond to that part originally....I have no interest...and its so obvious most would only do this as some 'escape route' to throw the table over and blame me again for something cause 'i misuse things according to their madeup community guide'.
Then back to the OP...you provided stat(28) as a solution to my OP. It circumvents the OP's problem, but so what? It honestly looks like a better method and it's still a solution, so thankyou for that part. Encore to that! The rest 'I leave' with you.


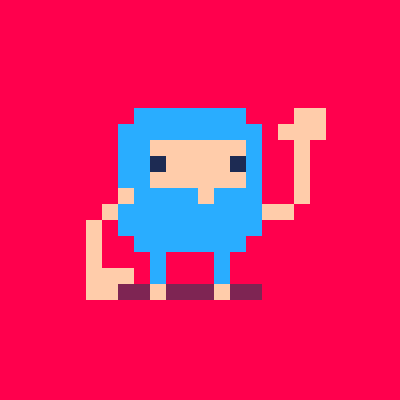
Hi @Cerb043_4
> the problem is stat(31) works like btnp() when I need it to work like btn()
Unfortunately this is impossible. The raw keyboard access is only really intended for devkit purposes (making tools etc), and a general way to use keyboard keys as in-game buttons is absent by design. The reason for this is to standardize and reduce the scope of controls as much as possible, especially for games listed on splore. Youre best bet is probably just to use esdf instead (player 2 key defaults).
In PICO-8 0.2.4b, stat(28) could be used but is still experimental and in future releases its behaviour will also likely change to only respond to new keypresses.


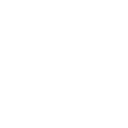
I found stat(28) useful in my carts, for keys (like del, etc.) that aren't otherwise reported by stat(31).
It's also useful for keys like backspace which shouldn't be allowed to queue too much (aka - they should be processed like btn or btnp, not like stat(31), or else someone holding backspace in a slow cart will end up deleting too much.)
I think having stat(28) give raw btn()-style behaviour is useful, though maybe there could be another stat that gives btnp-like behaviour too?
I found btnp() itself problematic in carts that run their own mainloops (potentially slowly - causing btnp to miss returning true), so it is useful to have a raw btn()-like function to fall back on.
[Please log in to post a comment]