Hi everyone! First time posting on the BBS, long time fan but relatively new coder!
I've been going through the various tutorials found in fanzines, youtube, etc. and decided I'd start the foundations of a barebones strategy game using a fixed map grid of 8x8.
My logic was like this:
Draw the map using the map editor, then use a for loop to iterate through each cell and add it to a set of nested tables for x and y, getting its sprite value as a foundation for what can be done. Plains, mountains, water, etc.
The code in this cart is very rough but I wanted to print the contents of the tile the cursor was currently on. There's probably other issues but every time I try to call maptable via the this code:
function gamedraw() local xs = curs.x local ys = curs.y cls() map_draw() cursor_draw() location = maptable[xs][ys] print(location) end |
the maptable returns a "?" value and crashes the cart.
For reference here is where maptable gets filled:
function build_map_table() maptable={} for x=1,8 do maptable[x]={} for y=1,8 do maptable[x][y]=mget((x-1),y-1) end end end |
I've attached the cart for reference as well. Really I figure its an issue with mget values being printed but I also wanted to see if this entire concept is ill-conceived or if there is an easier way to accomplish the same task.
Thanks y'all!



I think your problem is that Lua tables are 1 indexed and not 0 indexed so your first call to maptable[xs] is doing maptable[0] which is not valid. You have to start your table indexes at 1.



Hm you're right I need to watch for that, but I tried adding 1 to index to the correct table, 0 > 1, 1 > 2, etc, and its still giving me the error.
Attempt to field index "?"
on lines 36, and 52, the two maptable calls.


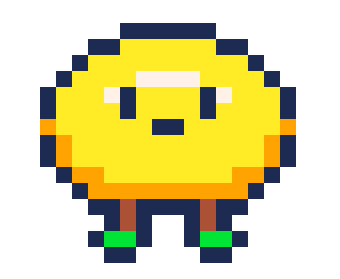
I did a few things to fix this issue.
First i moved all of your constant / initial variable declarations to the _init function to ensure they were being declared 100%, which didn't fix anything but it put my mind at ease. i've encountered weird bugs here and there from scattered variables.
Second i moved your function build_map_table() from the map_update function (which was being called during the _update function) and moved it to the _draw function, just before the value location is assigned using the maptable. that allowed the program to boot, but it was having inconsistent results, throwing that index ? error.
Third i took a look at your build_map_table function, and saw that you were using x=1,8 to to account for sequential table-like arrays in lua starting at 1, instead of 0 like most other languages.
I believe (and someone please correct me if im wrong)_that when assigning a variable to a table using the [] syntax, you're assigning that value as a key to the table, and not in that particular sequence.
In the context that you're using tables for generating a map, map tiles in pico-8 start at 0 and go on from there. x=0-127, y=0-63
so if you're iterating over numbers to build a skeleton of a table to use for storing tile information to be called, you'll probably want to start at 0 instead of 1, and just use key/value tables instead of sequential array-like tables.
Example:
function build_map_table() maptable={} for x=0,7 do maptable[x]={} for y=0,7 do maptable[x][y]=mget((x),y) end end end |
with this i was able to boot up your cartridge with i believe the intended behavior of printing out the tile information from maptable (stored from mget each frame) to the top left of the screen.
I can't really say if this is the best way of doing it, but it works and i hope it helps :)
[Please log in to post a comment]