For your games, you could have to manage a score greater than 32768 (I like games were I can score millions), and often, you want the score displayed in a fixed length, prepended with zeros (00072635).
But managing this can consume lot of tokens.
I came accros a solution I'm quite proud (I'm still learning Lua...) and wanted to share.
In Lua, if you do : "the score is "..score , score is converted into a string and then concatenated to the first one.
So to convert a number into a string, you just have to write : ''"..score
I wrote a recursive function that takes a string and a number (the length) and returns a string padded with zeros at its beginning to reach the desired length :
function pad(string,length) if (#string==length) return string return "0"..pad(string, length-1) end score=723 print(pad(""..score,7)) -- will display 0000723 |
Elegant and thrifty, isn't it ?
Then, you can use two variables, score2 will store the 10 thousands, score1 anything under 10000
each time your player scores something, you add it to score1 and you test if it's above 9999 :
if score1>9999 then score1-=9999 score2+=1 end |
Be sure the max score won't never be above 327689999 ... or manage it with a score3 variable
Here is the concept in action - score2 is displayed in orange, score1 in red:



Did you see this thread?


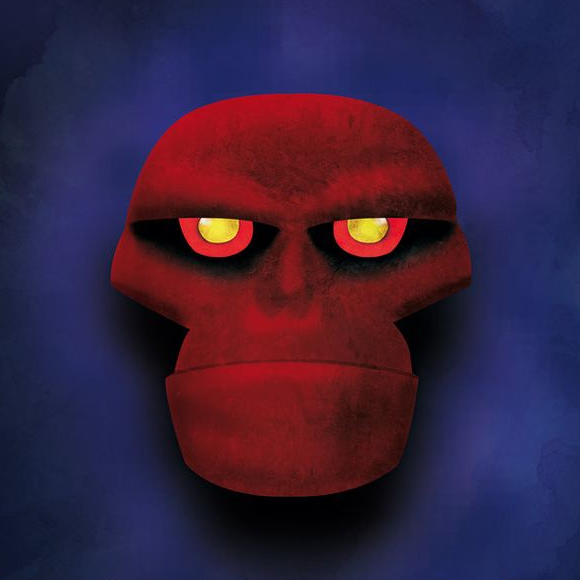
Dear... I didn't. And I'm confused. Would have been more productive if I had posted it there. Sorry
And now, I find my solution not as good as I thought:-(



If it works for you, it's more than good enough :)
But I think we can all learn from each other



Resurrecting an old thread but FWIW, I find this to be a much easier to understand for padding with smaller numbers, so thank you!


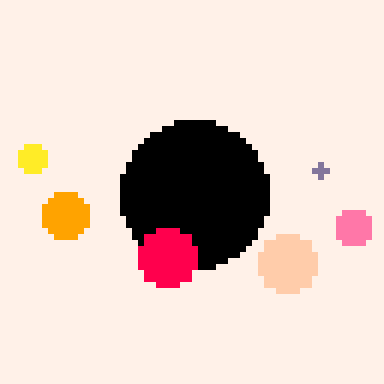
To recap this and the other thread (and possibly save some reading/time), this method with multiple variables is pretty easy to implement and can add a few digits to your "score," "money" or other high value. Or, if you just want the appearance of high numbers (without much precision), you can add some zeroes to the end (print(mynumber.."000")
) This works great, is very easy and may be all you need!
But, if you desire a large range with full precision using one variable, without needing to add any significant additional tokens or outside function, tostr()
now allows us to represent any number variable as a signed 32-bit integer, allowing us to precisely store any whole number from -2,147,483,648 to 2,147,483,647 with one number variable.
However, since this makes use of what are normally the fractional bits of a PICO-8 number (don't worry if this doesn't mean anything to you, just keep reading) we can't simply add regular numbers to our variable, we need to work in multiples of the smallest possible PICO-8 fractional values in hexidecimal, so for example 0x.0001
would be worth 1 point, 0x.000f
would be worth 15 points (because this is a hex value), and 0x.0010
is 16. For, say, 300 points, you would use 0x.012c
. You don't have to know hex, just use a decimal to hex converter to figure out the hex values you'll need.
Example:
mynumber=0 --add 1 mynumber+=0x.0001 --add 100 mynumber+=0x.0064 --add 500 mynumber+=0x.01f4 --add a random amount from 0 to 1800 mynumber+=rnd(0x.0708) --add 65536 mynumber+=1 --or mynumber+=0x1 --add a million mynumber+=0xf.4240 --add five hundred million mynumber+=0x1dcd.6500 |
You can also use the bitshifting operator >>
to convert (non-fractional) decimal values directly in your code, but this uses two extra tokens and an extra cycle or two.
--add 365 mynumber+=365>>16 |
Now, in order to represent our number variable as a 32-bit int, we need to use tostr()
with the second argument 0x2
(or simply 2)
Example:
--set our number variable to the equivalent of 1234 points mynumber=0x.04d2 --print our number as 32-bit int print(tostr(mynumber, 2)) --prints 1234 --try to print normally print(mynumber) --prints 0.0188 (not what we want) |
Much higher numbers with precision down to the individual whole number, without needing any libraries or added tokens!
Thanks to @merwok's post at https://www.lexaloffle.com/bbs/?pid=136497#p
[Please log in to post a comment]