Hello all...
Would someone be kind enough to point me in the direction of where I should be looking to solve my screen boundary problem.
I'm slowly working on a scrolling platform game and all's working quite well. I have bad guys that bounce about when they get hit. I wanted to have these bouncing guys hit and bounce back from the screen edge when they collide rather than the map edge. Much like having a wall all around the area you're currently looking at.
I may be over thinking it but... when the screen scrolls to the left, for example, is the left most edge of the screen still 0 or is there some sort of display edge I should be looking into??
Thanks in advance and I hope this makes sense.
Cheers.
Peej


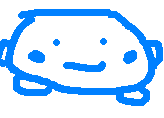
The screen coordinates go from 0 to 127, and you can draw things with negative coords or coords higher than 127.
Can you say more about your program? maybe share a screenshot or GIF (pico8 can make these, see manual for shortcuts)
> I wanted to have these bouncing guys hit and bounce back from the screen edge when they collide rather than the map edge
It’s possible for sure! It’s your code that bounces from map edge, given that pico8 does not have any collision built-in, so you can change what is being checked to change behaviour.
Are you using 'camera' to do your scrolling?
https://mboffin.itch.io/pico8-camera-follow



It's 1:13 in the morning here so no doubt I'll be thinking about this as I doze off but I was just wondering, as my screen is like a window to a wider map, so the screen edges change as the map scrolls?
Say the map scrolls left 10 pixels, is 127 still the right edge or has it moved 10 left? So the edge is now 137?
Yes I'm using camera to scroll.



Since you're using camera, yes, the screen boundaries will change. Move the camera 10 pixels to the right and the screen boundary will be 10-137. You can see this behaviour with this.
function _draw() cls() --camera(10) for i=0,100,10 do print(i, i, 0, 7) end line(137, 0, 137, 127, 7) end |
With the camera line commented out you'll see the numbers 0, 10, 20...100 spaced at 10-pixel intervals and there's a line drawn offscreen.
Uncomment the camera line and the numbers start at 10 and the line has come into view at the right edge of the screen.



Thanks for the replies peeps :-)
Yeah, I have a variable that stores the x on the camera to do the scrolling. SO I figured if I had this:
if enemy[1].mode=="dying" then if enemy[1].x>119+cam_x or enemy[1].x<0+cam_x then enemy[1].dx=-enemy[1].dx end end |
Then all would be well.
Yes, it works when the screen scrolls the baddie bounces off the new edges, BUT if he bounces at the right hand side, the camera glitches to the player (I think) in a sudden cut.
Not sure what's happening there...
Peej


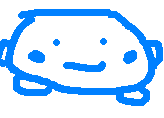
We can only give general answers about camera and coordinates in pico8, but without seeing any GIF or code it’s hard to help more! Can you add your cart here? You can also join the discord server and ask in the help channel.






@Peej
There's nothing in the code you posted in this comment which explains your camera glitch but you can safely get rid of
0+cam_x |
and just replace it with
cam_x |
I also noticed that you're calling map(0, 0) which you can just call as map() and it will default to 0, 0. Neither is a big deal but it saves you a couple tokens.
Your camera glitch isn't caused by the enemies as such but it is related to stomping. You can see the problem without enemies by commenting out the line
camera(cam_x,0) |
in your view_camera() function. (You can also get rid of another 0: camera(cam_x) does the right thing.) The camera won't track the character now so walk towards the right side of the screen and stomp. Even without the enemy nearby your glitch happens. As far as I can tell this is because when you stomp you turn off camera tracking and when the stomp is finished you turn camera tracking back on and the camera recentres on the character. The reason you probably thought it was the enemies, and the reason it didn't glitch every time, is probably because if the enemy hits you while stomping you fly backwards. The camera doesn't track that movement but when the stomp finishes the camera recentres. If the enemy doesn't hit you, the camera doesn't need to adjust.
The easiest fix is to just change view_camera() to this:
function view_camera() cam_x=plyr.x-68 if cam_x<map_start then cam_x=map_start end if cam_x>map_end-128 then cam_x=map_end-128 end camera(cam_x) end |
Now the character will always be tracked even when getting hit by the enemies so it won't need to adjust after the stomp. But maybe that's not what you want? In which case your camera tracking code will need to take into account that the player position may have changed and adjust smoothly instead of with a sudden cut.
More generally, I think you're sometimes re-using variables in your logic checks that are somewhat confusing in terms of what exactly they're supposed to be doing. For instance:
if not cam_track and plyr.stomps==0 then plyr.boost=3.4 shake() -- etc.... end |
It took me a couple minutes to figure out why killing an enemy should depend on whether or not the camera was tracking the player and why your stomp code was turning camera tracking off at all. With the fix I gave above you wouldn't actually need the cam_track variable at all (because now the camera always tracks) so you could rename cam_track to something like stomp_done or do_stomp or something—and maybe make it an attribute of the player table—which would make the condition a bit more sensible.
if not stomp_done and plyr.stomps==0 then -- or plyr.stomp_done or whatever... plyr.boost=3.4 shake() -- etc.... end |
Hope that helps.



Thank you @jasondelaat for another in-depth reply.
I recall that I have the cam track switched off when the character does the stomp/double jump thing because it was inducing a sort of motion sickness, bouncing the screen twice in quick succession.
May be I over thought that one.
In that bit:
if not cam_track and plyr.stomps==0 then plyr.boost=3.4 shake() -- etc.... end |
It's saying "I know you've turned the camera track off, so you must be double jumping, and I'm going to count down from 2 to 0 jumps left. When you get to 0 jumps left, shake the screen after the double jump!".
You've hit the nail on head though, and found the problem.
I've just gotta either add the tracking all the time, which will break the logic behind using the cam track to know I'm double jumping or basically re-write that whole section.
Cheers for the tips though.
Very much appreciated.



@Peej
Have you changed the camera tracking code since you started? You seem to be only tracking the camera x-coordinate so I'm not sure where the double bouncing thing would come from unless you were also tracking the y-coordinate at some point and then removed it?
But anyway, if you want to keep it how it is mostly I'd suggest just storing the characters position in a couple temporary variables before turning off camera tracking. Then, before you turn tracking back on, you can check if the current position is the same as the old position. If it is then just go ahead and turn it back on and if not then you can do a loop to scroll the camera back over to the character and then turn tracking back on. Shouldn't require too much extra work.



Thank you @jasondelaat
I included the first suggestions you made. It now works perfectly.
I even sped up the stomp section to make it slightly easier to kill an enemy, stomping with less height gain.
It's coming together quite nicely now thanks to your help.
Cheers.



Just realised, @jasondelaat. Yes, it took me a long time:
Because the camera has focus on the character all the time, the shake doesn't work.
[Please log in to post a comment]