Embarrassing question but please be gentle. I'm very new to all this.
I have this code: (Lower down there). It's a bit mock up really for this question.
Lower down still in my code I'm working on a collision detection bit that needs, among other things, the value of x.
As you can see, it's all referencing 'self' and I have NO IDEA how to get the x value from this line:
spr(17,self.x,self.y,1,1,false)
Would someone please help a noob out.
Many thanks
Peej
function add_new_enemy() add(enemy,{ x=80, y=96, w=8, h=8, dx=rnd(2)-1, dy=rnd(2)-1, life=20, draw=function(self) spr(17,self.x,self.y,1,1,false) end, update=function(self) self.x+=self.dx self.y+=self.dy self.life-=1 if self.life<0 then del(enemy,self) end end }) end |


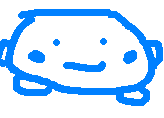
So there are two ideas there: you have a table that is a sequence of things ('enemy', with things at index '1', '2', etc — would probably be better named 'enemies'), and in that table you have other tables (with values accessible by the indices 'x', 'y', 'w', etc)
Example to get the first enemy from the table:
-- get thing from a sequence table enemy1 = enemy[1] -- get thing from a mapping table spr(17, enemy1["x"], enemy1["y"]) -- or using the dot shortcut (only works for string indices) spr(17, enemy1.x, enemy1.y) |
Now, you don’t want to do that for each enemy, so a loop can be used to do something for each item in the sequence:
for en in all(enemy) do -- 'en' is set to enemy[1] then enemy[2] etc until last spr(17, en.x, en.y) end |
The last thing is that a table can also contain functions. In the example you have, each enemy added to the enemy sequence table has 'x', 'y', etc but also 'draw' and 'update', meaning you can do this:
-- call a function that’s inside a table, passing the table for 'self' param enemy1.draw(enemy1) -- color is a shortcut to pass self automatically enemy1:draw() |
So putting all this together, this is how games typically update and draw multiple enemies or particles or items:
function _init() enemies = {} end function add_new_enemy() add(enemies, {etc etc}) end function _update() -- call functions to check buttons, call add_new_enemy if some condition, etc update_player() update_world() for en in all(enemies) do en:update() end end function _draw() draw_background() draw_player() for en in all(enemies) do en:draw() end end |
Hope this helps!



Sorry to take so long thanking you for this reply, merwok. Great detail there and very helpful.
Cheers


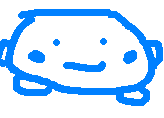
No worry! Someone has written this nice explainer recently: https://www.lexaloffle.com/bbs/?tid=44686
[Please log in to post a comment]