I made my first game in PICO -- a snake game! Biggest mistake: not going with the most intuitive approach to making the game from the start. I had a really crazy system of labeling coordinates on the screen that wound up backfiring, so I had to resort to what is admittedly a much more sensible way of making the game -- I now have tables for x positions and y positions of the snake and use those to keep track of everything. Play it on Itch here https://geojax.itch.io/snakehero and give me some feedback if you have any!!! Thanks!


Hey Decidetto! Thank you for the kind words! My first method was:
1) the screen was divided into 4x4 tiles, meaning the screen itself was now 32x32 tiles
2) I made a table called SCREEN with indexes (indices?) 0 through 32^2. This meant that I had a value in SCREEN for each tile on the screen -- in fact, the value for each tile was the color for that tile.
3)I draw the screen every frame based on that table. With a for loop, I go through each value. If it is 7, a draw a white rectangle there (the snake); 8, I draw a red square (the food); and 11, a green square (this formed a wall border around the screen). Example: if SCREEN[31]=7, then the tile in the top-right corner of the screen is white.
So that's how the screen is drawn. On top of that, there is another table called SNAKE that stores all the indexes at which the snake is drawn. Every frame, I set all the values of SCREEN to zero, then set the values corresponding with SNAKE to 7, and then drew that on the screen.
OK, phew. Last thing that applies to your question is the movement, and this is what made me pursue a different method: To move left or right, all I had to do was subtract or add 1 from the position of the snake's head. Moving vertically though? I had to add or subtract 32 from the position of the snake's head. Think about a square grid displaying numbers from 1-100(like this:https://www.google.com/search?q=number+1-100&safe=active&client=firefox-b-1-d&channel=tus3&sxsrf=ALeKk015EZEgAL_k89VBZR2U-cxjXm2G-A:1594423715824&source=lnms&tbm=isch&sa=X&ved=2ahUKEwiqys-Z68PqAhXULH0KHbgRCYwQ_AUoAXoECA0QAw&biw=1504&bih=929&dpr=1.5#imgrc=kaGDCCh7YepaOM). To move up or down a row, you add or subtract ten to the square you are on. It's the same logic.
So what was the problem? When the snake was moving vertically, I had very little control over it's movement -- If I moved any less than 32, it would not move vertically anymore, only horizontally. Just like you can't display a fraction of a pixel, my system was not letting me move the snake any slower than one tile per frame. Thus it was moving way too fast to play the game and didn't even look very smooth.
That's a lot and I'm not sure I explained it very well, so please reach out to me with questions if you have any!
P.S. Thanks again for the compliments on the music! I got the idea from 8-Bit Music Theory's Chibi Robo video. Here's a link to his awesome channel: https://www.youtube.com/channel/UCeZLO2VgbZHeDcongKzzfOw


PPS my second method also backfired -- in THAT method I had a table that contained an instruction for each segment of the snake that would be passed down every frame. So if the head moved left, then the next segment would receive an instruction to move left, and then the next one, etc. until the head moved a different direction and then they'd all follow suit. This method got out of hand fast though as different parts of the snake would just go their own way. Something must have been wrong in my conditionals.


Haha, your first method is very similar to how I'm storing tile data for a game I'm currently making: everything is just one big, one-dimensional array that is interpreted as two-dimensional by way of modulo and floor operators.
I did notice its weakness in how you wouldn't be able to draw things at intermediate tile positions that way, but luckily I'm making a block-based puzzle game and the array only represents the current state of the board. Currently moving pieces are not tied to the board and free to go wherever.
What was the result of the failed second method? A snake that would slide to the side instead of turning?
By the way, I noticed that if you very quickly press two directions such that the snake does a 180-degree turn, you can make it overlap itself. If you're REALLY fast with the timing so that it turns twice on the same pixel, you just die on the spot.


@Decidetto the first method wound up with segments of the snake moving off the screen and abandoning the head, and then they would come back on screen later seemingly at random. This was due to my strange set of conditions for assigning an 'instruction' to each segment.
I've noticed that the snake can overlap itself for awhile and can't figure out how to fix it. Turning around on the same pixel is new to me, so thanks for pointing that out -- I'll have to think about how to fix it. I thought about a time frame when input can't be taken, but that's noticeable and can lead to a feeling that the game is being unresponsive. I guess I'll upload the cart here now -- I didn't upload before since I wanted to drive traffic to my Itch page.

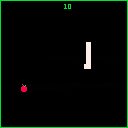
I'll have to think for a bit about how to solve these issues. Thanks for your help Decidetto!


You're very welcome! I enjoy helping with these things.
I think the "interval when input can't be taken" solution is the way to go, but you can do it slightly differently. Instead of not even processing any input that comes at the wrong time, you could queue it for use later. I believe that would solve the responsiveness problem.
Long post with proposed solutions ahead! If you prefer to think about things yourself, please disregard.
For solving the overlapping problem, you first have to ask yourself if you find it acceptable to always align the snake movement to a grid, only allowing turning when the snake head is at a corner point of the grid. This would solve both the overlapping and instant-death problems.
If you do, you could make an input queue, where you do take all the input (and play the little musical note belonging to it immediately when a button is pressed so the player knows their input is acknowledged), so it never misses an input but only processes them when it is reasonable to do so.
If you always want a player's first turn of a sequence to be immediately when the button is pressed and therefore not aligned to the grid, you can do the same as the first solution, but you'll have to extend it with a conditional check for what was the previous direction moved in and compare that to the next direction. Only if they together constitute a 180, wait the exact number of frames needed for the snake head to move past the width of its body (function of the current snake speed?) and then process the next input from the queue.
Depending on how you want to implement this, you want to go for different queue implementations. You want to either keep a short queue of only 1 or 2 entries so when additional buttons are pressed before the queued ones can be processed, you replace the last queued one with the most recent input, so the player can revise their decisions in the split second they have, or you make a longer queue so that when players press a sequence of buttons, the snake will act accordingly as soon as it can. This will likely be a balancing act between responsiveness and accurately representing a players intentions.


@Decidetto thank you so much for the advice! I like the idea of an input queue, and I think sticking to a grid is probably the best option -- this would make the game feel more organized and fair, I think. I'll go with your idea of not missing an input, but only processing input when it's the right time. I'll get back to you once I've got these things implemented (or at least partially). Thanks again for all your help! It's been extremely encouraging.



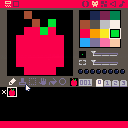
@Decidetto hey I just fixed the instakill bug! (I think -- I haven't been able to trigger it again.) Been a busy week, but I finally took a look at my input and all I had to do was change the conditionals from a bunch of if statements to a chain of if/elseif statements. I think it worked! It didn't fix the overlap issue, so I'll see what I can do about that. Here's the version where you can't instakill yourself.
It looks like a queue will still be necessary to fix the overlapping and I'm going to try implementing it right now.


That's a clever solution! The order of your "input check" if-statements now sometimes causes the snake to not turn at all when the inputs arrive at the same frame, but I don't think that is worth fixing as it would require processing the inputs and comparing them to the current direction to determine which of the two to execute and which of the two to queue. Two inputs arriving on the exact same frame probably does not happen often enough to make that worth it. (But if you wanted to be a hundred percent robust, that would be the way to go!)


@Decidetto I'm still hoping to implement an input queue since I do want the snake to stay on a grid... but this thing happens when you 'finish' a game where fixing small bugs is not as motivating as finishing the core game. I wonder if it would be better practice for me to continue flushing out this snake game or continuing on to another project. What do you think?
Also, what other game tools do you use? I've for a long time been floundering at a level a little past beginner but far under intermediate game development. I started in Unity, but I realized soon enough that there was a lot going on that I was not learning about -- for instance, having access to pre-programmed colliders and physics made me uncomfortable. So I started looking for more and more basic game engines until I was learning c++ and making tic-tac-toe in console, which was somewhat fun. How did you pick up gamedev? Just curious. I've just felt lost for a while and am curious how you picked up programming and all that.
Thanks!


Yup, I hear you. I think it might be more interesting for you to move on to something new, especially since it's hard to feel excited about Snake nowadays, unless you really do something completely different.
The musical touch was different and exciting! But I do think the diminishing returns have set in by now.
I'm sure there's plenty of other games that you might be able to think of an interesting personal twist for that would be great practice!
About how I picked up programming... first of all, thanks for your interest! Let's see.
I've done pixel art since I was 13 or something, having started by "enhancing" Sonic sprites, which involved scaling the raw pixels to 2x, then redrawing the edges, adding details and refining the shading. Looking back at the results, I'm honestly impressed I was able to do it that well back then. Since then I've always done a lot of pixel art, which has been a guiding line through my game dev journey.
Later I dabbled in Game Maker a tiny bit, but it was only in university that I was taught proper programming languages. Java, C++ and C#, then later Python (but that was mostly for neural network simulations). I made super simple games in raw Java. Tetris with pentomino pieces and a 3D type of Tetris where you try to fill a truck as optimally as possible with boxes. A lot of other projects in university gave me other relevant skills. For one project, for example, we had to program a pathfinding algorithm and have it find paths on a generated map. But I got extremely ambitious on the map generation and implemented way more terrain types than strictly necessary and set up all kinds of rules and conditions, which resulted in maps that actually looked topographically sound! Most other groups had maps that looked like random noise, haha.
Unity was a thing we used in later projects, after we had graduated from programming Java without an IDE (which, indeed, was a horrible experience and terrible idea, so I was very happy to move on to Unity). I've designed, programmed and done pixel art for a proper proof-of-concept game in Unity for a university project which got us a unanimous 10/10 and I couldn't be happier. I've also created a commercial classroom application in it from scratch and am currently using it for my Master thesis in university.
Further, my friends are a creative bunch. Many of them compose and arrange music, so I've picked that up as well, having made many arrangements of existing songs using MIDI and VSTs in digital audio workstations (like FL Studio etc.), but also in trackers such as FamiTracker and SchismTracker, and I've even learned a specific FM synthesis music programming language (PMD MML) that allowed me to program music that can be played back on 30 years old computer hardware. With that I've contributed native-format programmed recreations of legendary, previously unused and lost songs from a popular game series to a fanmade update patch that put the lost content back in.
Another friend does engine programming and he often involves me in far-reaching decisions, which has made me rather comfortable with memory maps and bitwise logic. I'm also often invlolved in game design and art direction decisions by the little hobbyist gamedev group I'm a part of with those friends.
I've never really considered myself all too experienced in game development, but having had to think of all these things to write down, it does look like a lot. I guess what it all means is that over the years I've slowly amassed lots of knowledge and experience with all aspects of game development, from game design, graphics and music to programming. I really see myself more as a creative director, though, ensuring the proper translation of the true vision of a game into a final product.
So, since those are not really steps you can easily retrace yourself without embarking on a 10-year trek, what advice would I give... I think if you want to quickly level up, so to say, you've come to the right place with PICO-8! Its reduced scope and integrated tools allow you to quickly prototype new ideas and if something doesn't work, you can abandon it without losing months of development time. @Krystman has made a great series of tutorials for a Breakout-type game and a Rogue-like game, which are wonderful reference material. The Breakout tutorial starts very basic but he quickly delves into topics that are relevant to truly everyone making games in PICO-8. Even though I'm quite experienced, I've been watching through the whole Breakout tutorial (currently around part 20) and it has taught me a lot of subtleties specific to PICO-8. I'd like to personally thank you, Krystian, for providing the push that got me to properly try PICO-8 for real! I'm enjoying it here.
As for the snake game--to recap, after this Great Wall of a post---I would indeed abandon it in favour of a fresh, new, exciting project that you can do many more cool things with. If you want to brainstorm about possible ideas, I'm always open!


@Decidetto thanks for that response! I love hearing people's stories like that. It sounds like you've been all over the world of programming and computer science! Neural networking to games to music to pixel art. I just taught myself C++ on codecademy.com. Codecademy is an OK way to start out on a language, but it really isn't comprehensive, so if I wanted to become proficient with C++, I'd have to go somewhere else (i.e. scour the internet). I think I've been confusing programming languages and actual products -- I learned C++ thinking that it would be the key to being able to make games, but no, it's just another programming language. It really seems to take a lot more than just an understanding of a programming language to make something good.
Honestly, I don't even know why I crave programming so much. At first I just wanted to make games, but as I keep learning about new ways programming is used, they all sound so fascinating to me. I'd even enjoy programming a database!
That FM synthesis music programming language sounds really cool. I found out about something called ORCA recently. The creators call it a programming language, but it's a little more than that. More like a text-based modular synthesizer or something. https://hundredrabbits.itch.io/orca I've never been able to get it to work since it won't recognize my speakers, but it seems pretty cool.
It sounds like you had a lot of fun programming that pathfinding algorithm! Does Java have graphical capabilities? I've been considering looking into it to make desktop applications, but I wonder if I should just stick with what knowledge I've got at the moment.
I started my programming journey in Unity. I quickly realized, however, that I was missing key programming and problem-solving knowledge. I also was overwhelmed by how much Unity offers, and I felt out of control. Using something like PICO-8 gives me enough control that I feel quite comfortable making games.
I just learned about bitwise logic the other day -- I had no idea what it was and looked up an article -- and it blew my mind! I'm sure there's tons of applications for it, although they're not obvious off the bad. I made a small application in C++ to help me practice guitar using them: https://www.onlinegdb.com/Bkcj2kNgw
I'm sure that's a silly way to use them but I had a lot of fun with it.
Thank you for your comment on the musical touch to my rendition of Snake! I could've added at least a little more music but I was excited to get my game online once it was working (sort of). I'm a musician before anything else, really; I've been playing classical guitar since 2012, and recently began composing instrumental music. I'm absolutely terrible with DAWs, but I'll have to figure them out sooner or later if I want any hope in the music industry.
Thanks again for your response! I'm thinking about making Flappy Bird now. I'll let you know how it goes -- I've got the basic gameplay down, I just need to add a score (which might be the most difficult part). After that, I'll look at @Krystman's breakout series which sounds like it is very good.
EDIT: quick question: how did you get started enhancing Sonic sprites? Were you hired to do it or just for fun? Actually, I can imagine that being a pretty fun afternoon. Also, did you make your profile icon? It's fantastic. I made my own icon one day messing around in Piskel. I'm pretty terrible at art, but this smiley face came out... so badly I had to keep it.
Thanks again for all your input and help and for telling me your whole story!


My parents have been really urging me to get back to music and composition, so I will probably have to call it quits on the game dev front before school starts, but I'm hoping I can keep up some programming with PICO-8 on the side.
@Decidetto I also have another question: how do you get a job as a creative director? How do you make sure a game comes out the way its creator intends? How do you make it how YOU intend? I've definitely had visions for games that came out terribly or not at all. As long as we're brainstorming, here's a couple ideas of mine:
1) a game where spikes are perpetually coming across the screen from left to right -- think Geometry Dash. Some spikes are red, some are blue. Instead of jumping over them, however, you switch between which spikes are visible. So, just before you hit a red spike, you press X, and now all the red spikes disappear, but the blue spikes are visible again! So before you hit a blue spike, you press X again, etc. You could probably build on this by adding other objects besides spikes, like a blue/red spring that jumps you onto a different track, or other Geometry Dash-type obstacles.
2) The player owns a flower shop. That's all I've got. Maybe they put together bouquets or something, but I don't know if you can make that fun. I was inspired by a Bitsy game where you bake a cake. Midnight Bakery? It was called something like that.
3) A really, really beautiful 2D runner through the woods at night running from people with flashlights. Like if Inside was 2D and an infinite runner.
Those are a few ideas of mine. Maybe when I get time I'll develop one of them.
Ooh, also I have one more question. What's it like developing an application in Unity? I've been wanting to try a hand at software development, and if that's possible in Unity, that could be pretty cool. Specifically, I want to make a speedy and accessible music notation software, since there really are no good options out there. Finale sucks, Sibelius is probably a little better, but mine would be completely focused on the composer experience, sacrificing functionality for speed and accessibility. No idea how I could get started making that though.


That's a cute chord application you made, I can totally see how you had fun with that and especially with trying to find a way to include bitwise logic in it.
I've taken a look at ORCA and it's so fascinating! I'd love to try it out.
You say it doesn't recognise your speakers, but the way it works is by generating MIDI events. Are you sure you aren't missing a device or software synthesizer to accept the output ORCA generates? Since Windows 8, Windows no longer offers access to the built-in MIDI synth, so you have to install an alternative. I myself have Coolsoft Midi Mapper, which offers a MIDI endpoint.
I actually tried ORCA between writing this previous paragraph and now, and it works for me using Coolsoft Midi Mapper, but it seems that the ORCA creators themselves offer a companion application called "Pilot" that can serve as MIDI endpoint, and there's even a 3rd-party companion application that can play back samples when instructed via MIDI CC calls from ORCA! Those companion applications might solve your problem. Have you tried them?
Java can do graphics, but all I've done is just "fill this rectangle with this colour" and such. Java in general is a horrible programming language to work with since it all runs via a slow and inefficient virtual machine that adds heaps of overhead on anything that you want to make. C# is extremely similar to Java and has the advantage of not needing a VM to run, so if you want to make desktop applications and you want something Java-like, I would instead suggest C#. I hear Qt is a good desktop application framework too, which works with C++, but I've never personally tried it.
Having made that classroom application and a desktop video player in Unity, I can vouch for the user interface functionality of Unity being easy to use; it can literally be as easy as click-and-drag. It still is a game development platform first and foremost, though, so I'm sure something like Qt, which is specifically geared toward desktop applications, is a better fit for the job, and it uses C++, so that should be perfect for you if you want to try making that music notation application.
About the sprites: when I was young, we had a few computers in the house. My dad's computer was the main PC, with Windows XP, and to gain access to the internet we would use one of those wi-fi USB dongles. My sister and I had a shared computer in the attic that we would play games on, like Jazz Jackrabbit, Rayman and the Sonic 3 & Knuckles and Sonic CD Windows ports. It was a Windows 98 PC, so it had no plug-and-play USB support meaning we couldn't connect it to our wi-fi network with those dongles and couldn't transfer data with USB flash drives either. The only plug-and-play method of transferring data onto and off of it was with 1.44 MB floppy disks, and, well, those don't hold much data. When looking for suitable files to transfer by floppy, I naturally settled on images, so I would start taking and editing screenshots in the games to edit for fun, and gathered inspritaion and other game screenshots from the internet-connected PC downstairs onto floppy disks.
The stuff I made was reworks of existing sprites, tilesets for custom levels in Jazz Jackrabbit or Sonic, completely unique sprites, like a head-on angle of the Super Emeralds seen in the Sonic 3 special stages, and a last, big category that I derived a lot of fun from: mock-ups with a hidden "error" in them. For example, in Sonic 3, the characters have 4-fingered hands for some reason, while in all the prior and later games, they have 5 on each hand. There's a moment in Sonic 3 where Sonic ducks in a cutscene to show Knuckles exiting a hidden passage, so I went ahead and sneakily edited Sonic's hand to have 5 fingers, like in all his other appearances. Similarly, I once took a screenshot when playing as Knuckles and swapped out a sprite of him holding on to a vertical pole in a water current for an unused equivalent. My joy in making those came from making the edits subtle enough that it would take people a lot of effort to even realise anything was wrong. I never did share them online, though.
My profile icon--thank you for your compliment--is from the main PICO-8 project I'm currently working on, though I prefer to stay quiet about it so as not to create any expectations. But I set a sprite from it as my avatar image as a little tease for the people who might recognise the character!
About how to get a job as creative director, the way I see it is that you would start at a game company in an entry-level, basic role, then work your way up by proving you have good design sense, are able to express your vision in clear words, and can properly manage a team of people.
The key to making sure a game comes out as intended is to write down the "experience" that you are envisioning for your players to have, before you start development. For example, should a big part of the experience be the feeling of being out of control? Or how do you want to evolve the difficulty in order to balance engagement and frustration? It is really about setting clear terms that define the main line of your envisioned product, and for every decision that comes up during development, take a step back and evaluate whether it would reinforce or detract from the vision. If it detracts, then think about ways to rework the idea so it can properly connect to the vision, or maybe reconsider the idea entirely.
I really like your game ideas! The first has me thinking of a combination of Ikaruga and VVVVVV. I'm thinking this would be great to gradually combine with platformer mechanics, so the player has to juggle the challenge of staying on the track with the challenge of properly switching states to phase through the spikes.
The third idea could actually be combined with the first, to define the story and art style of it! The people with the flashlights are what motivate your character to move through the levels, and the "spikes" you dodge are projectiles launched by the group behind you, which might have different types of properties. For example (if running left-to-right) they could have bullets that fire straight from left to right, but they could also have a type that they lob over you, onto the path ahead of you, which then explode in a column of fire of a particular colour that you need to swap for to dodge. I feel this could maybe be fun to work on together.
Is it this game you meant with the example in your second idea? https://dreamingamaris.itch.io/the-midnight-bakery
It was fun! I liked the "soft" criteria given by the characters. They didn't specify "I want this exact cake", but gave a general direction they wanted the cake to go in and left the rest open to your interpretation and judgement. That's a very refreshing and personal kind of gameplay! Especially when they're happy with the cake you thought up for them and you feel like what got you that victory was your people skills rather than your deduction skills. I got a total score of 18/21 in my session.
I'm sorry to hear you might be giving up on gamedev for the time being, but if you continue that Flappy Bird project, I'd love to see it, and if you have any music you want to show off, feel free to hit me up too! Actually, I'm sure it's really charming to others how we're essentially pen palling here, but it might be time to graduate to another form of communication if we're going to be talking much more, haha. What options do you have?
Edit: d'oh, I'm sorry I keep forgetting to @ you. Adding it in later probably won't ping you, so oh well.


@Decidetto Good idea to try migrating to another platform. What's the safest way to do so? I have Discord, email, a twitter account I never look at... my Itch page but I'm not sure there's any communication that can happen there. What works for you?


@geojax Email and Discord are options for me, though I suppose out of those two, sharing my Discord tag online where everyone can see it is less of a problem than my email address.
But I'd rather find a way to have it get into the hands of as few other people as possible. If you don't mind sharing yours openly, that'd be a great help, but otherwise I'll have to figure something out.
[Please log in to post a comment]