I'm trying to make a checkers game as a first game, and have the function loc_type(loc) which should return 1 if the location has a black piece and a 2 if the location has a white piece, and zero if it's empty.
This works perfectly well for setting up the board but I'm now trying to code selecting a piece. But when I call loc_type, it seems to think that pos = {5,7} (Which is the last white piece I spawn).
I'm really unsure how to fix this. Any thoughts?
Code for reference
--game logic function _init() -- enable mouse poke(0x5f2d, 1) curr_peice = nil val = {-1,-1} -- starting places for peices (might want to automate this?) blacks = {{0,0},{0,2},{0,4},{0,6},{1,1},{1,3},{1,5},{1,7},{2,0},{2,2},{2,4},{2,6}} whites = {{7,1},{7,3},{7,5},{7,7},{6,0},{6,2},{6,4},{6,6},{5,1},{5,3},{5,5},{5,7}} end function _draw() --clear screen cls(0) -- draw board and peices draw_board() draw_peices() -- draw cursor spr(9,curr_x*16,curr_y*16,2,2) print(val,3) if curr_peice != nil then spr(9,curr_peice[1]*16,curr_peice[2]*16,2,2) end end function _update() -- calculate current square curr_x = mid(0,stat(32)\16,7) curr_y = mid(0,stat(33)\16,7) val = loc_type({curr_x,curr_y}) end -- graphics function draw_board() for i=0,7 do for j=0,7 do -- checks if square is black is_black = ((i+j)%2) rectfill(i*16,j*16,(i+1)*16-1,(j+1)*16-1,is_black*7) end end end function draw_single(single) piece_type = loc_type(single) -- draws the peice if piece_type == 1 then spr(1,single[1]*16,single[2]*16,2,2) elseif piece_type == 2 then spr(3,single[1]*16,single[2]*16,2,2) else print("error: no peice here") end end function draw_peices() foreach(blacks,draw_single) foreach(whites,draw_single) end -- utility functions function loc_type(loc) is_black = object_in_table(loc,blacks) is_white = object_in_table(loc,whites) if is_black and is_white then print("error: two peices in the same place") return -1 end if is_black then return 1 elseif is_white then return 2 else return 0 end end function object_in_table(obj,t) for t_obj in all(t) do if t_obj == obj then return true end end return false end |


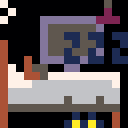
I am unclear what you mean regarding "But when I call loc_type, it seems to think that pos = {5,7} (Which is the last white piece I spawn)."
That aside:
"Lua compares tables, userdata, and functions by reference, that is, two such values are considered equal only if they are the very same object." Source: https://www.lua.org/pil/3.2.html
So two tables with the same values in are not the same table (unless they are also the same object).
In object_in_table change the check for equality from if t_obj == obj then to:
if t_obj[1]==obj[1] and t_obj[2]==obj[2] then |
Does that fix the bug, or could you explain it further?
[Please log in to post a comment]