Well, I'm trying to make a rhythm game and I want the enemies to change sprite (To look as they were dancing) and attack following the tempo of the current song. It's my first game and I don't know too much about code or how pico-8 works. I tried to make a T variable that add one to its current value each frame and to use a the "%" operator to sync the frame counter to the beat but it's hard to do it that way and I feel like it doesn't sync too well. Can anyone help me to code it?


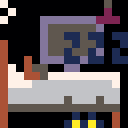
2021 December edit
Relevant quote from there:
"46..49 Index of currently playing SFX on channels 0..3 50..53 Note number (0..31) on channel 0..3 54 Currently playing pattern index 55 Total patterns played 56 Ticks played on current pattern 80..85 UTC time: year, month, day, hour, minute, second 90..95 Local time 100 Current breadcrumb label, or nil 110 Returns true when in frame-by-frame mode
ⓘ
Audio values 16..26 are the legacy version of audio state queries 46..56. It only operates on the current state of the audio mixer, which changes only ~20 times a second (depending on the host sound driver and other factors). 46..56 instead stores a history of mixer state at each tick to give a higher resolution estimate of the currently audible state."
Using the newly added stats 46..56 it should be possible to easily get accurate enough information to achieve this.
I have left my original answer (for now) but with the increases in accuracy the new stats 46..56 bring, I suspect my original answer is unnecessarily complicated for the accuracy it tries to achieve, and is therefore no longer a good answer.
Here's a cart which shows one way of doing this. I have stuck to syncing the sprites with the tempo of the current song, using the stat command to get information on this. You have said you are new to coding and to pico-8 so I didn't want to introduce much more than you were already looking at, but I can think of other ways to achieve this (perhaps someone else already has a suitable example sitting around that they can share).
I have extensively commented my code, to help you or anyone else new to coding.
Here's the code with the comments removed for anyone who finds that easier to read (I don't think I deleted anything other than comments, but if this doesn't work, see the cart instead).
Notes
Ticks and frames
Offset attacks
Direct control of SFX
Other posts to look at
Edits
Correction of numeral by 1, & change line to rectfill



I recently implemented beat sync in a cart I'm working on. I made it a generic system where you can register callbacks to occur on even subdivisions: full note, half note, quarter, eighth, sixteenth, 32nd. After including the code, just do this:
-- perform once per quarter note sync.on(4, function() do_thing() do_other_thing() end) -- or if you're only doing one thing sync.on(16, do_thing) -- every 16th-note |
Note that in this version, I set the expected speed to 16 in the library. That's because my main rhythm track uses a speed of 16, and other tracks are all multiples of that. You could modify this library to require explicit initialization with a speed of your choice, or just hardcode it to something else.
https://gist.github.com/amacdougall/a1c977e2036d529bbdb76109cffdb3ea
[Please log in to post a comment]