
I have a tile object which contains the sprite number and other properties. I want to update different properties at different times, instead of passing all properties all the time.
First I tried this approach:
function update_tile(values) tile = values end |
but of course that unsets anything that you don't pass in values
Then I settled on the following approach:
function update_tile(values) for k,v in pairs(values) do tile[k] = v end end |
Am I correct that this is the right way to do it, or is there a better (code readability or less tokens) way to do this?



If you're passing a table, then that makes total sense to me.
Also, I'm not sure if you know, so I'll point out that Lua has a nice bit of syntactic sugar where it allows you to omit the parentheses when calling a function that takes just one inline table or string:
-- these... update_tile({x=10, y=20}) print("hello world") -- can be written this way, without or with a space update_tile{x=10, y=20} print "hello world" |
Pardon if you already knew this, I just like to share useful info on the off chance it's new to people. :)
Another method you could use for your function, which might be good as an accessory rather than a replacement, would be to take advantage of the built-in varargs functionality in lua. Taking an argument of "..." creates a local variable that is a tuple (not a table) of all args passed at and after that point.
function update_tile(k, v, ...) if k != nil then -- handle the first two args tile[k] = v -- see if there are more return update_tile(...) end end ⋮ update_tile("s", 123) update_tile("x",10, "y",20) |
It's just something you might like better in some situations. One nice side effect is that it doesn't create and delete a table in the process, so it thrashes memory a bit less. However, if you're already giving it static tables, that's not an issue.
Technical notes: The function I offer invokes Lua's tail-call/tail-recursion optimization, so that the recursion won't keep going deeper and deeper on the stack. Returning the unmodified result of the recursive function (even if it's empty) is how this is done, because it makes clear to Lua that nothing at all happens after the call, so it can just move the first two elements out of "..." and into k,v and jump back to the start of the function. It's a really elegant way to express things sometimes, without the stack overflow fear usually involved with recursion.



Took me a while to get back (night-time game dev), but wanted to thank you for confirming and clarifying here. It was very helpful :)


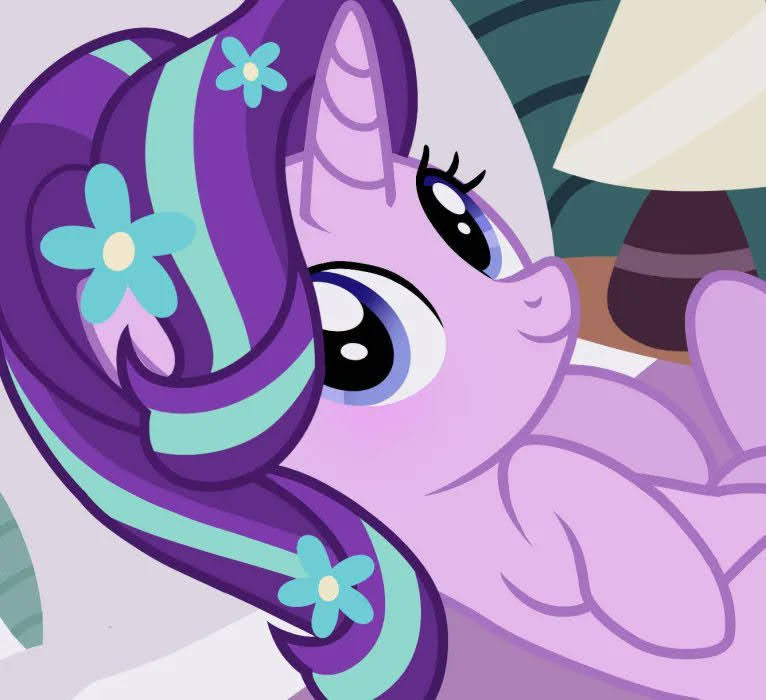
Looks like you don't need it in your case, but you can also check if an argument is provided with
if values then
or more strictly
if values~=nil then
[Please log in to post a comment]