Hello!
I'm learning Pico8 and programming in general, so I'm trying some very very simple things.
I've made a triangle using lines, and using the arrow keys I can move one of the vertices of the triangle.
How can I make it so if I push button5 the arrow keys stop moving that vertex and start moving the second one, press again and changes to the third one and finally press again and changes to the first.
Thank you so much!
So far this is the code I have:
function _init() x1=100 y1=20 x2=40 y2=40 x3=60 y3=60 c=7 end function _update60() cls(1) if btn(0) then x1-=1 end if btn(1) then x1+=1 end if btn(2) then y1-=1 end if btn(3) then y1+=1 end end function _draw() line(x1,y1,x2,y2,c) line(x2,y2,x3,y3,c) line(x3,y3,x1,y1,c) end |



In general, you would use a variable to keep track of which vertex is being moved, behave conditionally on this variable when the directional buttons are pressed, and update this variable when button 5 is pressed.
One way to do it (though probably not the best way) would look something like this:
function _init() x1=100 y1=20 x2=40 y2=40 x3=60 y3=60 c=7 vertex=1 end function _update60() cls(1) if btn(0) then if vertex == 1 then x1 -= 1 elseif vertex == 2 then x2 -= 1 else x3 -= 1 end end -- and so on ... if btn(5) then vertex += 1 if vertex == 4 then vertex = 1 end end end |
You might notice that this would require a large quantity of code to handle all the cases with if statements. I would do this a different way: store the vertex coordinates in a table, then use the "vertex" variable to index into that table:
function _init() vertexes = { {x=100, y=20}, {x=40, y=40}, {x=60, y=60} } c = 7 vertex = 1 end function _update60() cls(1) if btn(0) then vertexes[vertex].x -= 1 end -- and so on... end function _draw() line(vertexes[1].x, vertexes[1].y, vertexes[2].x, vertexes[2].y, c) -- and so on... end |
A table is a value that can contain other values. It can use numeric indexes or labeled indexes. In this case, I'm using a table containing all of the vertexes with numeric indexes (1, 2, or 3), and each vertex is represented by a table with labeled indexes (x, y). Now when I update a coordinate, I don't need an if statement for each case. I can just use the numeric index in the vertex variable to refer to the currently selected vertex, then use the x and y labels to refer to the coordinate values for that vertex.



Great advice, and I'd definitely go with the table option.
One small note: I'd use btnp (http://pico-8.wikia.com/wiki/Btnp) rather than btn for button 5 otherwise you'll get unexpected results.
Add print(vertex,0,0,9) under the cls(1) and you'll see what I mean.


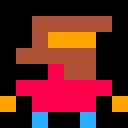
Wow!!! Now I get it!
"then use the "vertex" variable to index into that table"
This is what I didn't understand but now seems obvious: you can substitute ANY number by a variable, even the index number of a table!
I know it's a super noob thing to say but I didn't know it until now...
You can even change the button number by a variable so instead of saying btn(0) you can say btn(b) and modify it with a variable... so cool...
Thanks a lot dddaaannn!
ps: you are right neilpopham! You need to put btnp or the triangle goes crazy!
ps2: I wish I had pico8/lua when I was a kid haha
[Please log in to post a comment]