Okay, there is a thing called fate, and there is a thing called destiny. WOW ! Check this out. Minutes BEFORE I posted this, I found my old compressor revived and updated by Jasper.
https://www.lexaloffle.com/bbs/?tid=27892
Hmm ... I sure hope the work he did doesn't completely mirror what I've been slaving days over. :)
Okay, yes, new compressor. How does it work ? Very simple.
It takes a memory location and length and converts it from 8-bit binary data to 6-bit text data.
But it does more than that, it also compresses that 6-bit data and with a LEVEL in mind.
Likely you won't need anything more complex than 2, but it can go all the way up to 255 if you need it to.
Here's how to use it, for instance, to make a logo screen for your game.
From command mode use: IMPORT IMAGE.PNG
Which is a 128x128 custom image either using the 16-color palette of PICO or not. If not, it will convert internally, and it may not be what you want, so consider using the palette.
In your program type: BIT8TO6CLIP(0,8192)
and RUN.
This will compress all of that image you imported and record the compressed data to your clipboard.
Back to your sourcecode, choose a free line in your program and press CTRL-V to paste it. Done !
To display this, beneath the definition, use BIT6TO8(T,24576)
You can also use my routines to compress and decompress sprite or map data. Just give it the appropriate memory locations and memory lengths.
If you have any further questions or need more example code, please let me know.
Also look to this useful tool from the lab, SRAM SMASHER.
https://www.lexaloffle.com/bbs/?tid=31632
Hope This Helps !


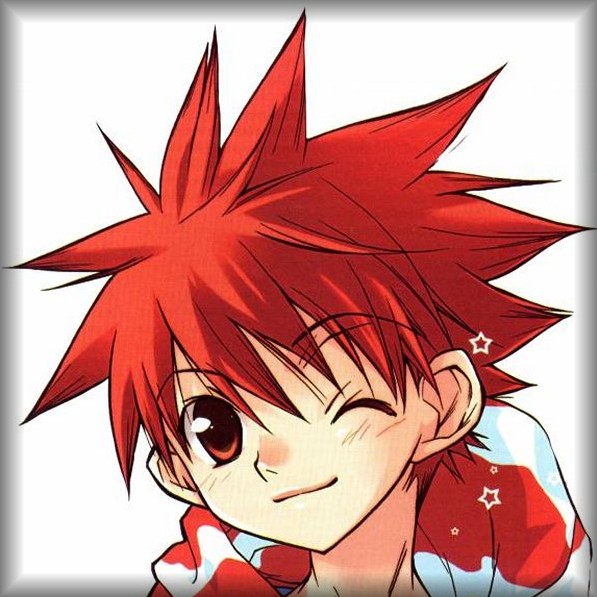
Here is a BARE BONES program to get you started.
Contains only the functions needed for converting 6-bit and 8-bit images and data to compressed strings and back again. It is also set for the default compression level of 2. Likely you don't need anything more complex than this for 16-color elements.
134 lines of code
582 tokens
2.5k total characters (2493) of available 64k
.
-- "bones" -------------------- -- bare minimum code ---------- -- main program --------------- function main() cls() info() print"" print"put your program here." print"" end--main() -- init global variables ------ function initglobal() chr6x,asc6x={},{} local b6=".abcdefghijklmnopqrstuvwxyz1234567890!@#$%^&*()`~-_=+[]{};':,<>?" local i,c for i=0,63 do c=sub(b6,i+1,i+1) chr6x[i]=c asc6x[c]=i end compressdepth=2 end--initglobal() -- functions ------------------ -- convert integer a to char-6 function chr6(a) local r=chr6x[a] if (r=="" or r==nil) r="." return r end--chr6(.) -- test bit #b in a function btst(a,b) local r=false if (band(a,2^b)>0) r=true return r end--btst(..) -- return asc-6 of string a -- from character position b function fnca(a,b) local r=asc6x[sub(a,b,b)] if (r=="" or r==nil) r=0 return r end--fnca(..) -- return string a repeats of b function strng(a,b) local i,r=0,"" for i=1,a do r=r..b end return r end--strng(..) -- convert compressed 6-bit -- string to 8-bit binary -- memory function bit6to8(t,m) local i,d,e,f,n,p=0,0,0,0,0,1 repeat if sub(t,p,p)=="/" then d=fnca(t,p+1) e=fnca(t,p+2)+64*fnca(t,p+3) t=sub(t,1,p-1)..strng(e,sub(t,p+4,p+4+d-1))..sub(t,p+d+4) p+=d*e-1 end p+=1 until p>=#t p=1 d=0 e=0 for i=1,#t do c=fnca(t,i) for n=0,5 do if (btst(c,n)) e+=2^d d+=1 if (d==8) poke(m+f,e) d=0 e=0 f+=1 end end end--bit6to8(..) -- convert 8-bit binary memory -- area to compressed 6-bit -- clipboard ready sourcecode function bit8to6clip(i,m) bit8to6(i,m,0) end--bit8to6clip(...) -- convert 8-bit binary memory -- area to compressed 6-bit -- string or save to clipboard function bit8to6(i,m,f) local j,k,l,p,n,c,t=0,0,0,0,0,0,"" m+=i-1 for j=i,m do p=peek(j) for k=0,7 do if (btst(p,k)) c+=2^l l+=1 if (l==6 or (j==m and k==7)) t=t..chr6(c) c=0 l=0 end end for i=1,compressdepth do j=1 repeat c=sub(t,j,j+i-1) d=sub(t,j,j) n=0 p=j if d=="/" then j+=i+3 else repeat ok=1 if (c==sub(t,p,p+i-1)) n+=1 p+=i ok=0 until ok==1 or n==4095 end if n>0 and n!=nil then a="/"..chr6(i)..chr6(n%64)..chr6(flr(n/64))..c if #a<i*n then t=sub(t,1,j-1)..a..sub(t,j+i*n) j+=#a-1 end end j+=1 until j>#t-i end if f==0 then printh("t=\""..t.."\"\n","@clip") else return t end end initglobal() main() |


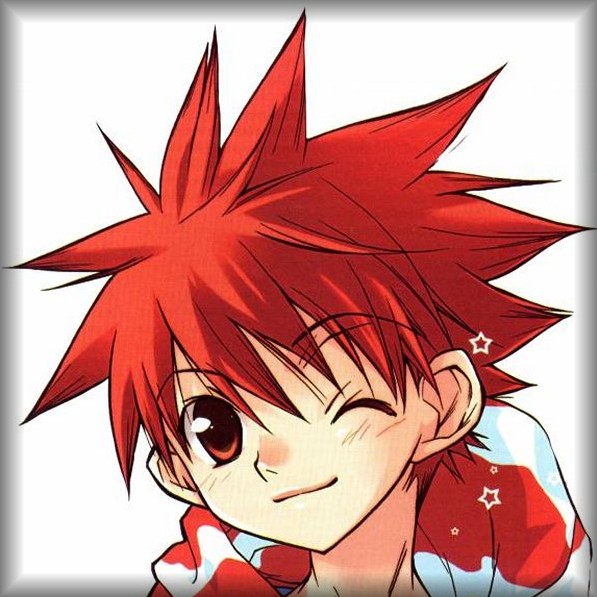
Glad you like it. May I say I really like your Avatar. Small pixels but you can definitely make out the figure, holding a gun ?


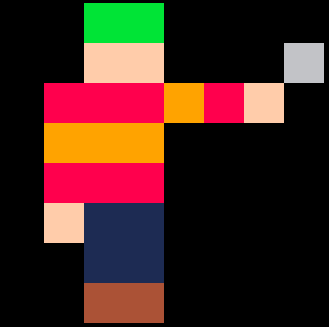
yep thanks! This is the player avatar in the first level of my game. When I finish the game, i'll let you know (even if it's my very first game)
:)
[Please log in to post a comment]