

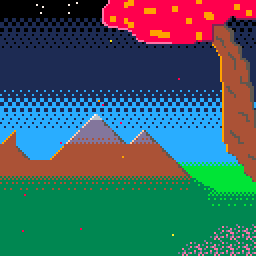
So it looks like you are detecting collision correctly. I'd suggest thinking about how to prevent movement that causes an intersection rather than fixing an intersection that has already happened. Does that make sense?



For me, I learned collision detection on an Youtube-Video from User "Uncle Matt", maybe it is useful for you too^^.
Playlist (especially Video No. 5):
https://www.youtube.com/playlist?list=PL_T5GuTZSAoC63B8nVmstVNJA5odk-ViH



Basically what you want to do is change whatever move event you have from
[i][b] [Pseudo-Code] [/i][/b] If(key_pressed) then move_key_direction(key_pressed) end |
to this instead
[i][b] [Pseudo-Code] [/i][/b] If(key_pressed) then if(no_collision_at(where_player_would_be_next))then move_key_direction(key_pressed) else move_to_point_of_collison() move_speed=0 end end |



Oh wow I hadnt realized people had commented omg
Thanks for the suggestions!!



Thank you all so much, your advice was super helpful!! (Also realizing "if not x then" was possible instead of just "if x then" made things a lot easier)
I'm having still trying to figure out how to make the collision more accurate - there are still areas where you can slip through solid blocks or where the wrong movement direction gets blocked.
(no gravity/acceleration this time just to keep things more simple. sprites inspired by hyper light drifter)


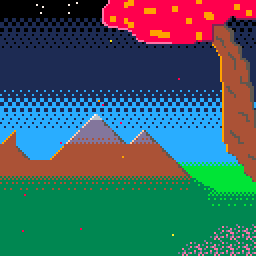
Nice screen scrolling.
You might want to look at your mget's. Not all of them are in the correct direction.
However, there might be a better place to look where you are going than in the mget's.
A couple unimportant organizational things to think about:
The stuff you are initializing at the top could probably go into the empty _init() function instead.
You call hero() in _draw(), but maybe it would fit better in _update() since it updates the player's position.
It looks like you are calling solid() just to update tx and ty. Perhaps you could write a function that converts pixels to tiles instead.
It's nice to see you found some time to take another crack at it. (-:
Good luck again!



Thanks!
I tried putting hero() in _update() originally, but for some reason it hides the hero sprite.
I thought solid() was already converting pixels to tiles with this??
tx = ((px-(px%8))/8) ty = ((py-(py%8))/8) |
For the sections of collision I tried basing it off of the player's pixels rather than the player's tile, but for some reason it didn't work at all
if not fget(mget(px-1,ty),0) then px -=1 |


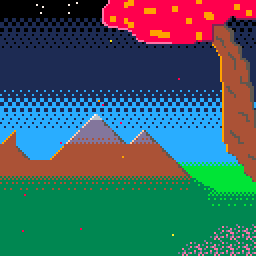
Solid() is doing that, I'm suggesting making a function to convert from pixels to tiles since you don't always need to do the rest of solid whenever you want to convert from pixels to tiles, which can end up being useful in a lot of places.
You're close. mget needs tile positions and not pixels (so px won't work), but you can get the tile position of the pixel in question .
The reason putting hero() in _update() wasn't drawing the sprite is because you call spr in hero() and since _update() always happens before _draw(), it would draw the sprite, then the bg and map on top of it.
Here is my version of your code doing all the things I've mentioned. Hidden in case you don't want to be spoiled on solving collision.
[Please log in to post a comment]