I have lots of different tables for different things.
Adding functions into these tables looks like a good idea, because I can write every update or draw for every type of enemy the same way. Something like this:
for e in all(enemies) do e.update() end |
The only thing I want to know, is how can I address the table within it's function?
For example:
enemy = { x=0,y=0, update = function() [b]--how to address these x and y variables from this update function?[/b] x+=1 y+=1 end } |
Writing something like e.update(e) seems stupid. Maybe what I'm talking about isn't even possible, I just couldn't find anything on this subject.
Thanks for any help


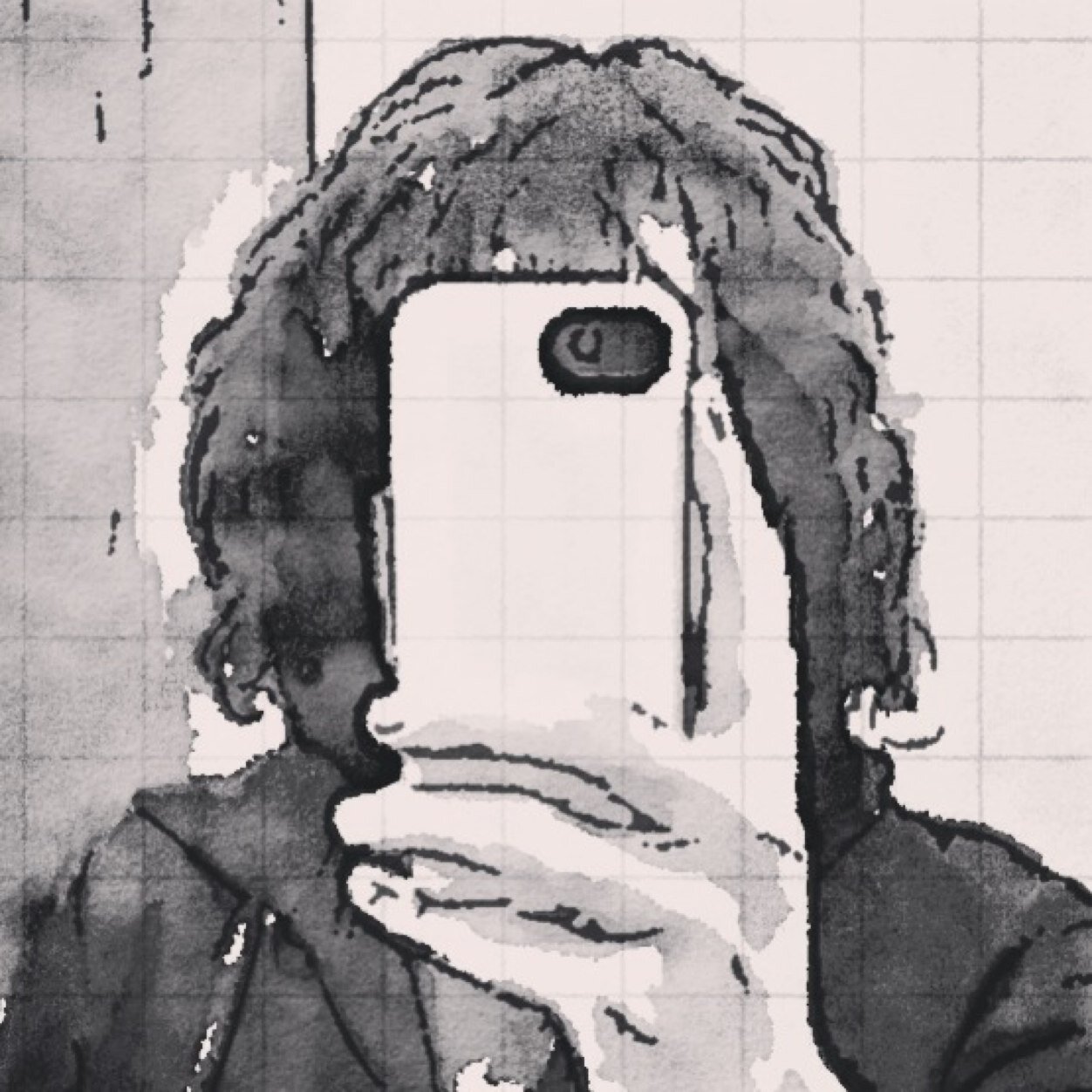
You could do something like this, which I think is called a closure:
function make_enemy(x0,y0) local e={x=x0,y=y0} e.update =function() e.x+=1 e.y+=1 end return e end entitylist={} function _init() for i=1,50 do add(entitylist,make_enemy(rnd(128),rnd(128))) end end function _update() for i=1,#entitylist do entitylist[i].update() end end |



That works, but has the disadvantage of creating a separate function object in memory for each instance. You might prefer using Lua's approach to object oriented programming. This involves several language features, including dedicated syntax for defining and calling methods, the "self" keyword, and "metatables" for setting up method inheritance.
http://pico-8.wikia.com/wiki/Setmetatable
https://www.lexaloffle.com/bbs/?tid=3342


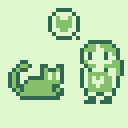
a third option:
enemy = { x=0,y=0, update = function(self) self.x+=1 self.y+=1 end } |
note that self
is not a special name, it's just a parameter like any other.
then, instead of using the dot method call syntax, you use the colon syntax:
enemy:update() |
this is shorthand for passing in the enemy object to its own method, as if you'd done this:
enemy.update(enemy) |
if i'm not mistaken, this sounds almost exactly like what you're asking for.
(side note: not well illustrated by this trivial example, but contrary to what the "expanded form" i gave may suggest, the expression to the left of the colon will be evaluated just once.)



Yeah, I see how it's eating up memory. Better find something more suitable for lua.
Thanks, everybody!
[Please log in to post a comment]