Just came across another nasty little thing about PICO. When referring to an array, it does not round down to the nearest integer when you make a regular array. Try the following:
cls() a={} for i=0,7 do a[i]=i+1 end for i=0,7,.5 do print(a[i]) end |
The results are:
1 NIL 2 NIL 3 NIL 4 NIL 5 NIL 6 NIL 7 NIL 8
Let's try the same thing in BlitzMAX:
Strict Local i#,a[8] ' must be +1 of total (0-7) For i#=0 To 7 a#[i]=i+1 Next For i#=0 To 7 Step .5 Print a#[i] Next |
The # represents that this is a floating point variable.
Results in this case are:
1 1 2 2 3 3 4 4 5 5 6 6 7 7 8
Which is the standard reply from this and many other BASIC programming languages.
Now apparently this does mean that it is possible to have fractional arrays. Something I've never seen until now.



Lua and pico have tables. Tables are not arrays, though you can make a table behave like an array. Also this is not basic.
Tables can have any index! This makes them neat and powerful and useful! And for convenience you get integer indexes, starting at 1, if you don't specify an index.
Also, if you want complete control of your code, don't let the interpreter round for you.


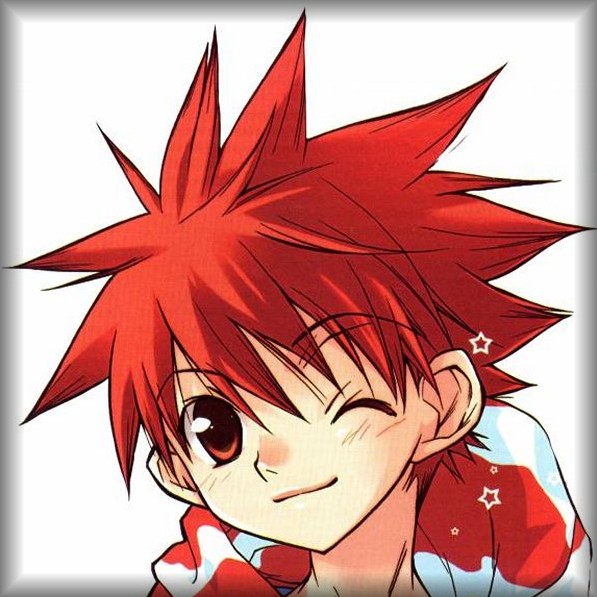
I have found you only get a starting index of 1 if you define your array in a table, Rytrasmi:
britec={1,13,14,11,9,6,7,7,14,6,6,6,7,12,15,15}
I know some BASIC languages had the ability of:
SetStartArray= and then you could enter zero or one.
BlitzMAX defaults to zero, which I think it should be.
But you are not limited to one if you define the array yourself or create it by other means.
dice={}
dice[0]=1
dice[1]=2
dice[2]=3
dice[3]=4
dice[4]=5
dice[5]=6
As it was, I needed to use FLR() to retrieve the value of a changed coordinate on the screen, which I had stored using integers 0-127 and 0-127.
I noticed you cannot have an array that is:
for i=0,7 do for j=0,7 do board[j,i]=0 end end |
So just how do you get a 2-dimensional array easily in PICO without multiplying inside of a 1-dimensional array ?



Dimensions are a construct that's only going to lead you astray. Also..."I have found you only get a starting index of 1 if you define your array in a table"...yes, of course, you must define your array in a table because tables are the only data structure that Lua has.
Here's how you get a 2d array in a Lua table:
a={ {1,4}, {2,9} } |
And here's how you address it:
print(a[1][2]) |
The above would output 4.


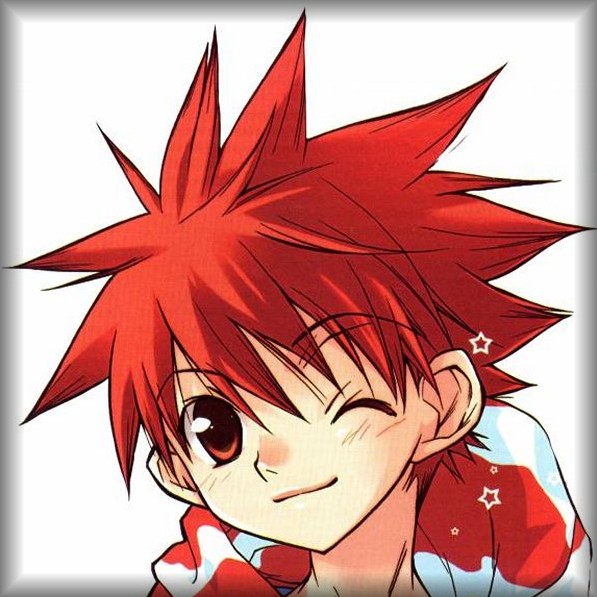
How would I do the following using board[][] ?
board={} for i=0,7 do for j=0,7 do board[j,i]=0 end end |



Try this.
board={} for i=0,7 do board[i]={} for j=0,7 do board[i][j]=0 end end |
So, board is a table with 8 items. Each item is a table of 8 zeros. It looks like this:
{
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
}
If you say board[m], you get the m-th "row", which is a table. If you say board[m][n], you get the n-th element of the m-th row.
If this seems weird and unnecessary, keep in mind that when basic says:
integer board[999][999] |
You'd reserved 1,000,000 integers and that memory is used. It's like 1MB or more of emptiness. In Lua you only pay for what you need. You can say:
board[0][0]=100 board[999][999]=100 |
and you've only taken 2 integers of memory. For sparsely populated arrays, this is a huge memory improvement.
Also, you're not limited to 1 data type per array, as you would be in basic. Tables accept whatever you give them:
guy={ {"bob",x=5,y=2,power={1,2,3}}, {"olga",x=21,y=44,power={3,3,3}}, {"peter",x=5,y=2,power="lots"} } |


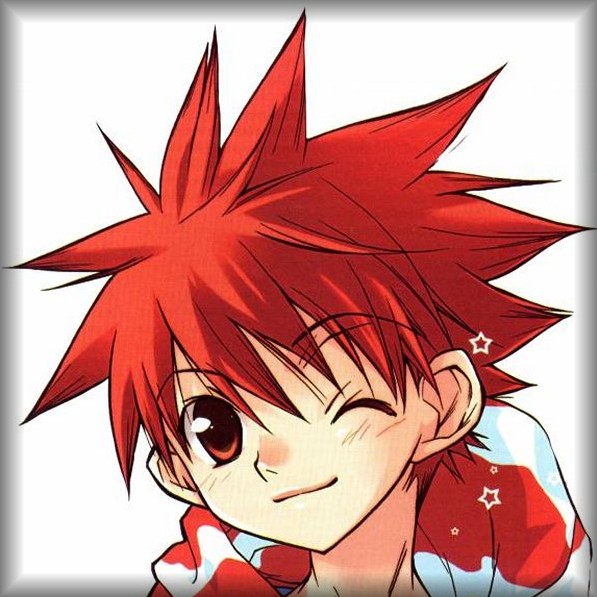
Is there a way to do it with I being the first FOR, J being the 2nd, and the array is handled THUS: board[j,i] ?



j and i are just placeholder names...call them whatever you want. Here, they're swapped:
board={} for j=0,7 do board[j]={} for i=0,7 do board[j][i]=0 end end |
You need a particular letter to come first?? Why?


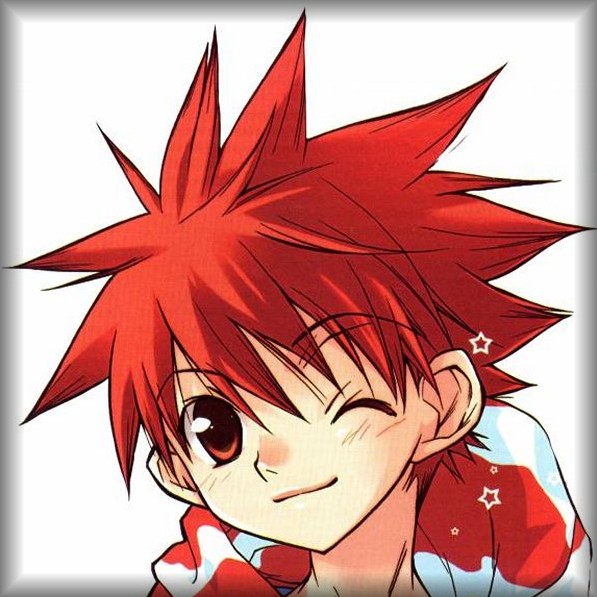
I've always had I be vertical and J be horizontal. I would always have the outer FOR/NEXT loop be vertical and I, and J was the inner FOR/NEXT loop to cover horizontal.
That and I've always had horizontal be the first argument in a 2-dimensional array and vertical be second.
So - I guess it's a challenge. :) Can it be done ?



Yes, of course it can be done. Though I will say that in math/physics convention, i->x->horizontal and j->y->vertical, with i and j being the notation for vector components on the x and y axes, respectively.
So, where you could have initialized each item of the board table with an empty "i" table as you traversed the i,j loops, you now need to initialize each item of the board table with an empty "j" table before you traverse the loops.
board={} for j=0,7 do board[j]={} end for i=0,7 do for j=0,7 do board[j][i]=0 end end |
But whatever floats your boat.


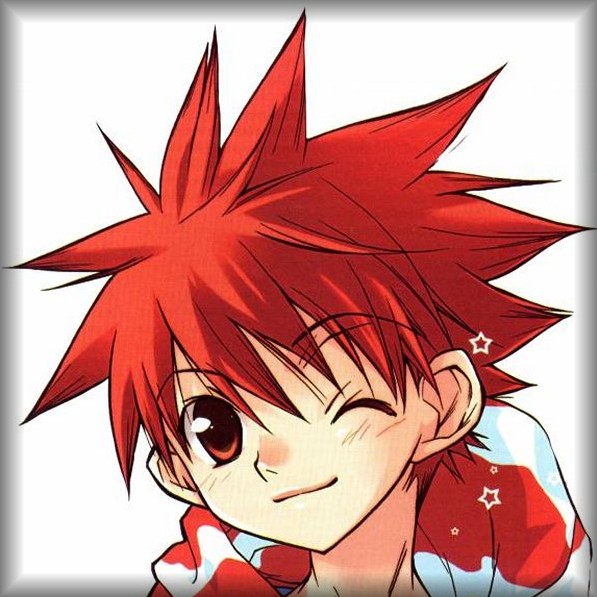
Hmm ... I like the code that you wrote and that it can be done. It's just there is no way to simply do:
for i=0,7 do for j=0,7 do board[j][i]=0 end end |
?
Because =I= can definitely see how this could be done in an alternative future game making language.
MEM CONTENTS: BLANK
MEM CONTENTS AFTER running code above:
board[0,0]=0
board[0,1]=0
...
board[0,7]=0
board[1,0]=0
board[1,1]=0
board[1,2]=0
...
board[7,6]=0
board[7,7]=0
TOTAL: 64 elements created and added to LIST.



::Shakes head::
You've got a very powerful language in front of you, one that's used to script some of the biggest titles available and some of the smallest (i.e., pico). I suggest you learn it. The techniques Lua teaches are transferable to many other languages, both modern and old. Yet, you seem hyper-concerned with syntax...I mean, I've mentioned several cool things about Lua in this thread and you're conclusion is to make a new language that abides by your particular syntax habits? The fact that [i] and [j] need to be swapped justifies a new language?
Forest for the trees, man...


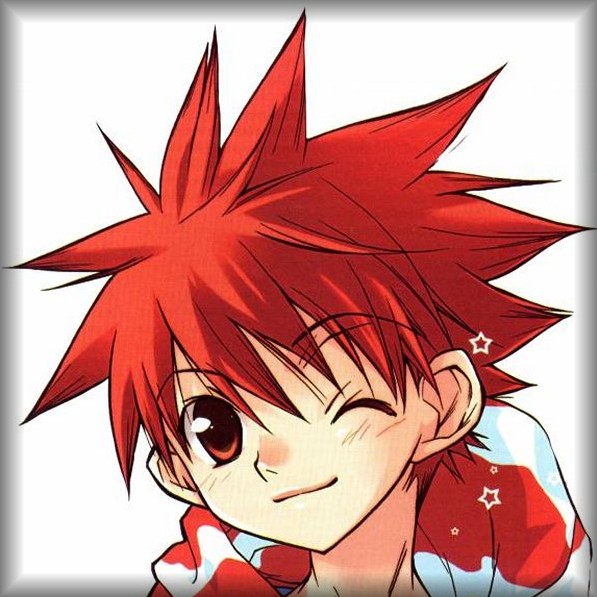
It's just daydreaming, Rytrasmi. Don't fret it. :) You gave a working solution. I will definitely look it over and thanks !
It should be possible to make a function that 'initializes' a double array as you have so I don't have to use 3 FOR/NEXT loops. I'll do some experimentation.



OK, then!
Though this begs the question, why even initialize the array at all? If having 0 everywhere means something (like ocean on a map), then fine initialize away. But if 0 means nothing, then just test for nil when you need to:
board={} --do stuff here, including messing with i,j values --add a new horizontal if the one you want doesn't exist if board[j]==nil then board[j]={} end --do more stuff here, including messing with i,j values --now we want to set a j,i coordinate to a value of 5 if board[j]~=nil then board[j][i]=5 end |


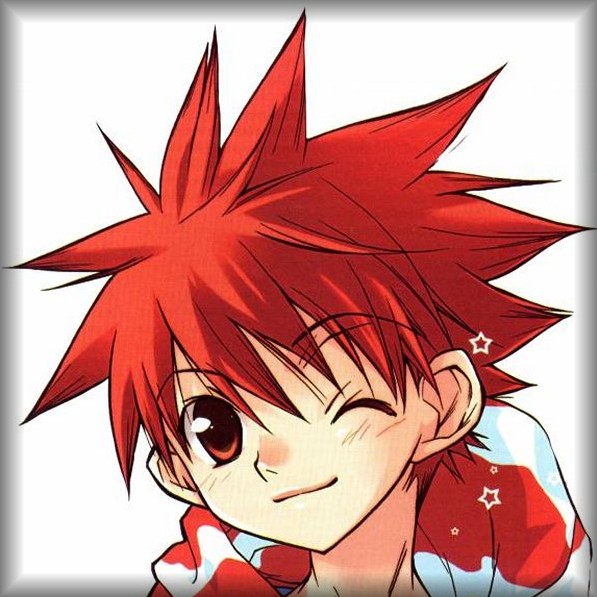
Zero is just an example. Let me assure you it would contain a value of significance. I just chose zero to flesh out the array.
[Please log in to post a comment]