Hi,
I'm learning at coding so i have tons of questions... so i will use this thread to try to find help. Thanks in advance!
I'm trying to make a new game and i need that a 1-block 8x8 sprite moves tracing a circular path.
Any help?
Thanks & sorry for my bad english



I feel your pain...while I'm not new a coding, Pico8 was the first time in a long time that I've need to actually program something - no helpers, no plugins, just good old fashioned programming.
And that means math...which I've had to learn slowly to make the games I want. It's been a long, slow road for me but as I've been learning formulas and making functions, every game gets a little easier and cooler.
That being said...here's what I use to have a object/sprite rotate around a single point in a circle. It's not really "tracing" a path...I'm sure there's a way but I'm not smart enough to figure that out. But this snippet will get your sprite to rotate/orbit around a point at a given speed and distance from the center.
--"planet" that a moon will go around planet={x=64,y=64,rad=50} --moon that rotates around the planet moon={ x=0, y=0, speed=.006, --speed of rotation ang=0 --starting angle of rotationcle (0=right,.25=up,.5=left,.75=down) } function _update() moon.ang+=moon.speed moon.x = cos(moon.ang)*planet.rad+planet.x; moon.y = sin(moon.ang)*planet.rad+planet.y; end function _draw() cls() circ(planet.x,planet.y,planet.rad,5) --just a reference print("\140",moon.x,moon.y,10) --draw your sprite here end |



Wow great! It works like a charm.
PD: Maths.... I wish they had told me that mathematics are
essential for videogames XDDD I had paid more attention back in school


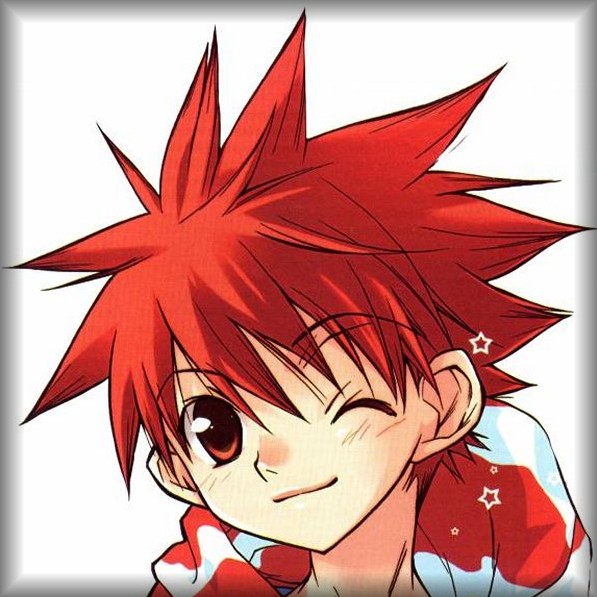
I can add to this.
Math is VERY essential ! Highly essential. Critically essential.
That and the ability to extrapolate data or interpret what might be easily understood to a person - to write it out where a computer can also understand and do it.
If you want to be master of this PICO language, I suggest you read every little bit of the "pico-8.txt" included with your purchased PICO language.
For extra credit, you can go here and get some good ideas on coding too.
https://www.lua.org/pil/contents.html
You might challenge yourself with a few sample codes.
[1] Write code that moves a sprite left, right, up, and down when you hit any of the 4-arrow keys.
[2] Write code to bounce a ball around on the screen. The edges reflect it.
[3] Write code to play some simple music you write.
Advanced.
[4] Generate a simple tiled map and scroll it from right to left.



Another maths problem...
Sprite A wants to go to where sprite B is tracing a fluid diagonal... not a irregular one like in the gif.
I want a smooth diagonal...
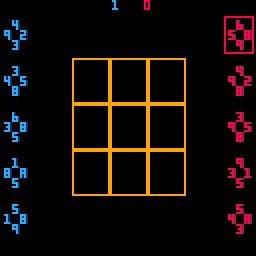
Any sugestions?


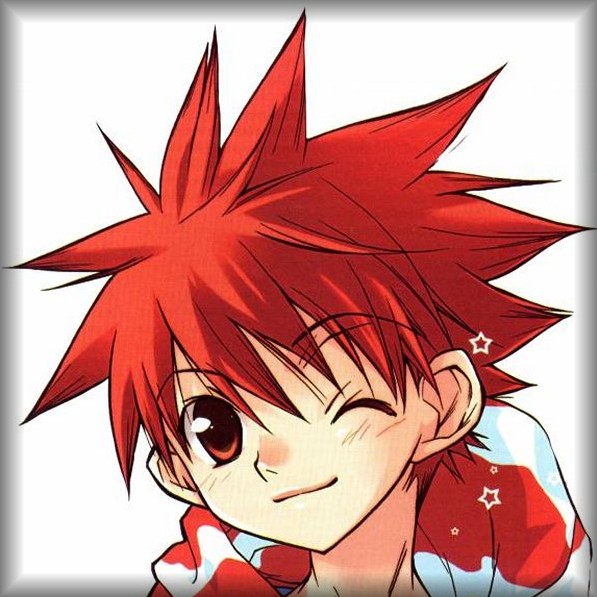
You and me both, Hokutoy. I have need of a diagonal tracing array. I wrote one years ago. I don't need it just yet, but one tool I'm working on will REQUIRE it - not just for cosmetic display.



@Hokutoy, regarding diagonal movement: The atan2 function is what you're looking for. Use it to get an angle to a destination, then use the sin and cos functions to turn that angle into y and x components.
Here's a method in my current project that does this:
function movetowardspoint(actor, destx, desty) if actor.z > 0 then return end local dx = actor.x - destx local dy = actor.y - desty local distp = sqrt(abs(dx)^2 + abs(dy)^2) if distp > 4 then local o = atan2(dx, dy) local pvx = cos(o) * actor.dv local pvy = sin(o) * actor.dv actor.vx -= pvx actor.vy -= pvy if dx > 4 then actor.flipsprite = true elseif dx < -4 then actor.flipsprite = false end end end |


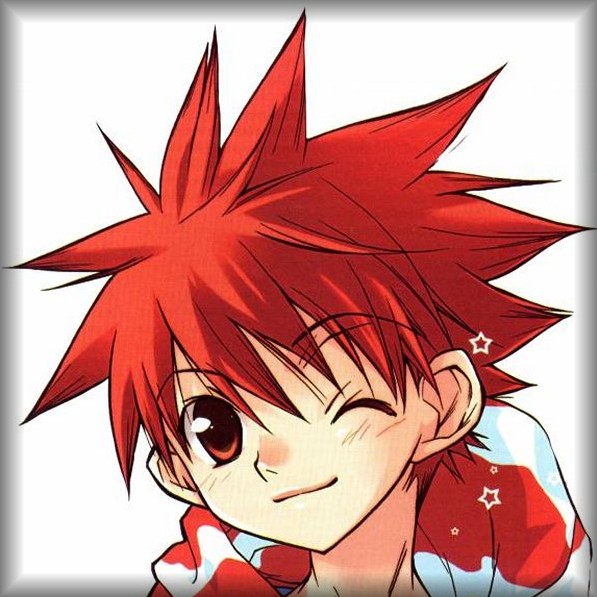
Well now Fweez, that's a real brainweave. I seem to remember figuring out years ago by trial and error a way to draw from any point to any point using simple floating point math - no need for Sin(), Cos(), Atan2(), or Sqrt().
I think I wrote it in QBasic somewheres around here ... Ah ! There it is. Converting.
32-lines, 137 tokens. Floating point calculations only.
-- draw line between two random -- points w/o complex formulas -- by dw817 cls() rect(0,0,127,127,13) ::again:: x1=flr(rnd(124))+2 y1=flr(rnd(124))+2 x2=flr(rnd(124))+2 y2=flr(rnd(124))+2 -- ^ pick random coordinates a=abs(x1-x2) b=abs(y1-y2) if a>b then b=b/a a=1 else a=a/b b=1 end pset(x2,y2,8) repeat pset(x1,y1,7) if x1<x2 then x1+=a else x1-=a end if y1<y2 then y1+=b else y1-=b end flip() until abs(x1-x2)<1 and abs(y1-y2)<1 goto again |


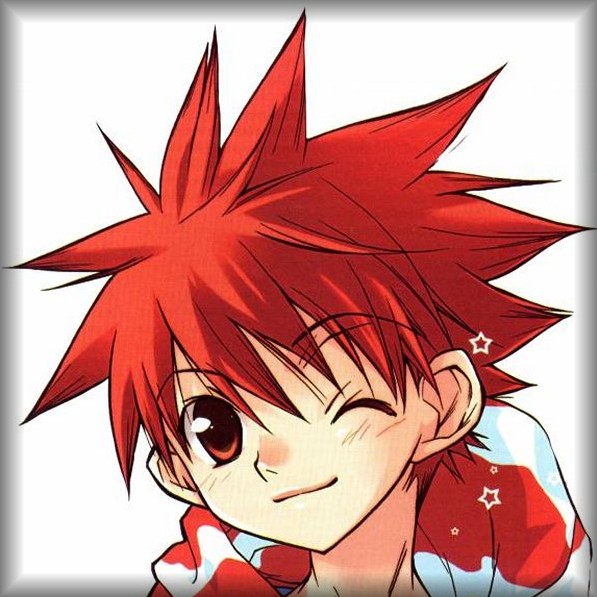
Hokutoy, did you ever get your cart posted ? Did you understand how mine works without using SIN(), COS(), or SQRT() ?



Yes dw817 it'a a nice aproach!
My cart is not posted or finished... too many real life things at the moment... i need some free time for coding :\
And the ::again:: --> flip() goto again is something new for me so it was great to know it!
Thanks


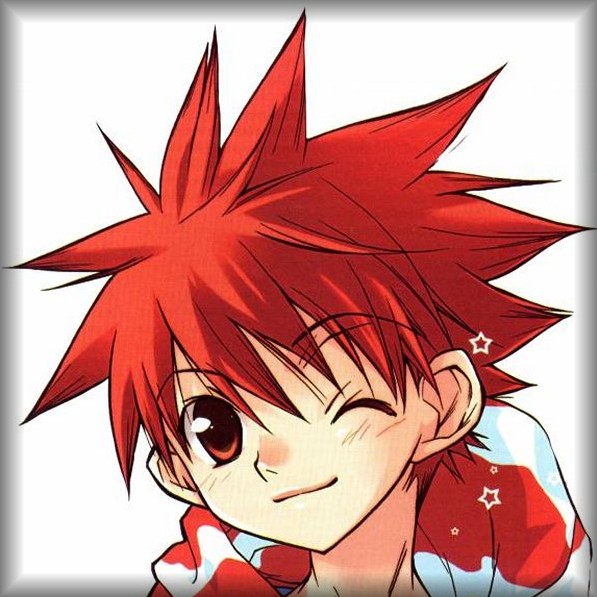
Hi, Hokutoy:
If you're not knowing.
FLIP() slows down your program and tries to prevent against and flickering. It's not as good as FLIP() for some other languages I've used as graphics can and will spill through if there are a lot of them.
Best to use FLIP() at parts in your code where you do want to show what updates to the screen you've made at that time.
GOTO uses double colon "::" front and back labels. GOTO is a marvelous command. With it, you can exit any loop, no matter how tight and GO TO where you need to.
Here's hoping you find time for your cart - I really like the initial animation you posted and it appears you have some kind of mathematical numbers game in mind.
Definite interest there ! :)
[Please log in to post a comment]