64 values of save data ain't enough for you? Try using this! These functions will allow you to save up to 2,048* values in persistent data! Anyone's free to use it in their own projects; I'm personally releasing this under CC4-BY-SA to allow works using these functions to be sold.
*If you only accepted ON and OFF as acceptable values.
Here's a comprehensive manual on how to use these functions:
A brief on this function set:
- inttobin(value, decimal) where you throw in any acceptable number from Pico-8, as well as an indicator to pull from either the whole number space or the decimal space, and it'll give you a binary table based on that number.
- binread(table, start, range) where you throw in a binary table generated by inttobin, specify where to start extracting bits, as well as how many bits to extract, and it'll give you a new binary table of just what you selected.
- binins(table, 2nd table, start) where you throw in two tables: a destination table and the binary table you want to insert, as well as where to insert the binary table, and it'll give you a new binary table with the desired binary table inserted in. Perfect for cramming in multiple values into one!
- bintoint(table, decimal) where you throw in a binary table, as well as an indicator to write to either the whole number space or the decimal space, and it'll give you an integer based on that table. Essentially the final destination when you want to save (second-to-final if you want to use both the whole number space and the decimal space).
If there are any questions, suggestions, or bugs that I should be aware of, let me know :o
Also let me know if you use any of these functions in your own save data in your game!


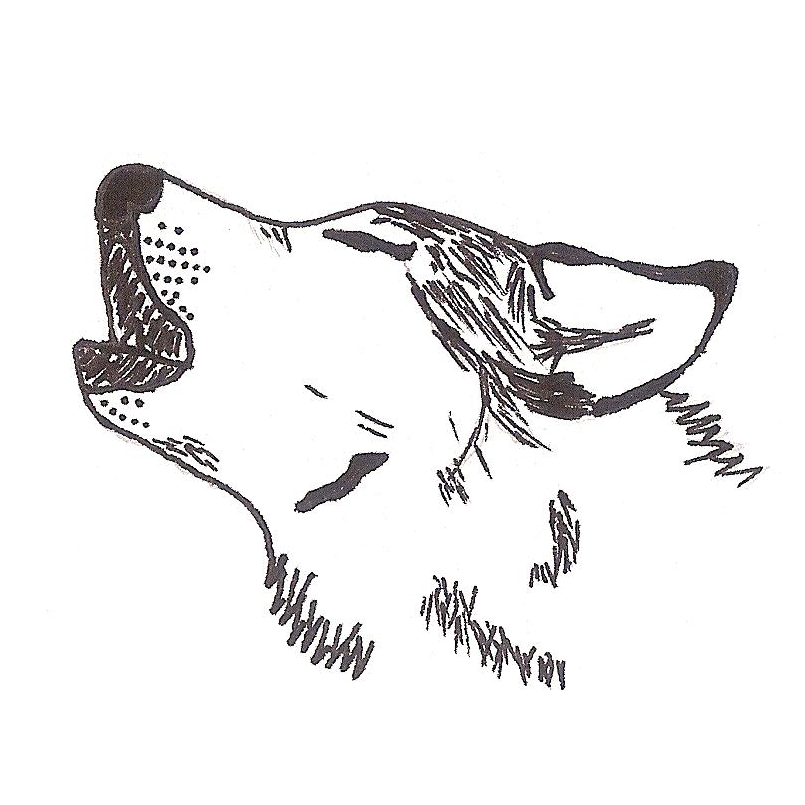
What's the license on this? :P I assume we can use it, but..can we?


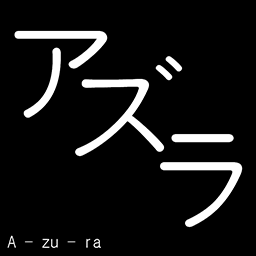
Oh, whoops
Forgot to check the CC license :o
Also yeah, anyone's free to use these functions in their game! I'll also be developing this further since there's a lot more that could be done with it. My next goal is to tap in to the decimal space so I can expand the functionality to use all 2,048 bits (256 bytes) of Pico-8's persistent data.


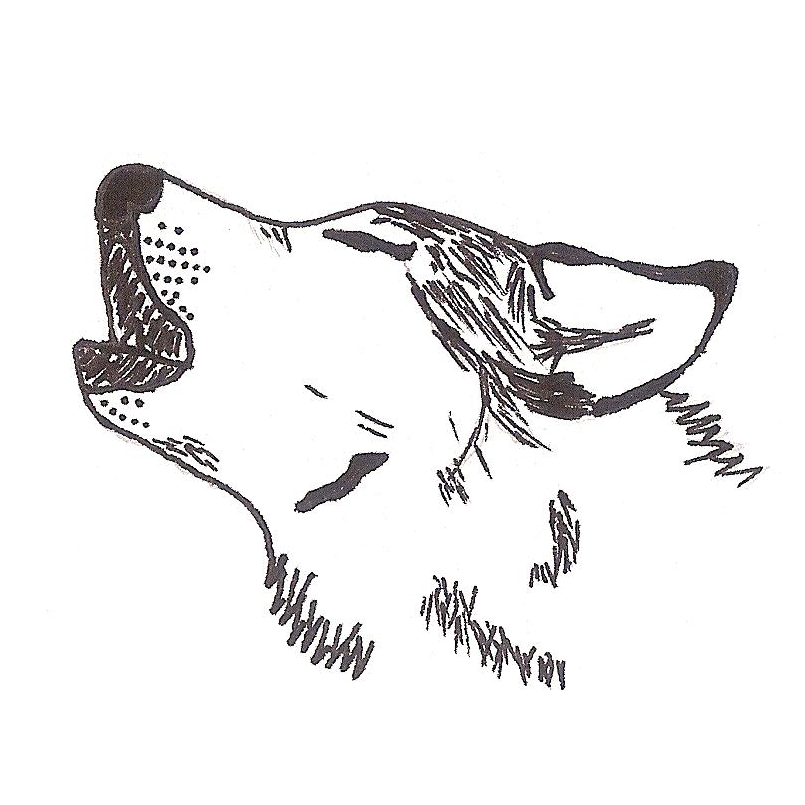
I had been wondering why you had said only 1,024 switches were possible. xD (That is until I started poking around and realized you weren't using that section.)
Would it be possible for me to be able to use it under a license allowing sale of games at pay-what-you-want with a minimum price of $0? The CC licensing on this site is for non-commercial use, but I have an idea and I would like to put it up on Itch.io and allow purchases.


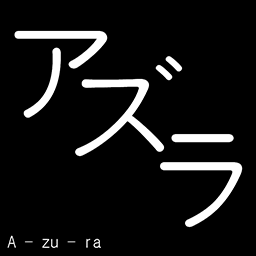
That would be fine by me. I probably might opt for CC BY-SA so if anyone really wants to use these functions and sell their work, they'll be free to do so.


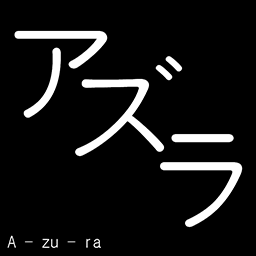
Version 1.1 is now available for use.
New to this version is the ability to read from and write to the decimal space.
The next version will focus on expanding that functionality so you can read from and write to both the whole number space and the decimal space. As of right now, you can only read from and write to one or the other, not both.


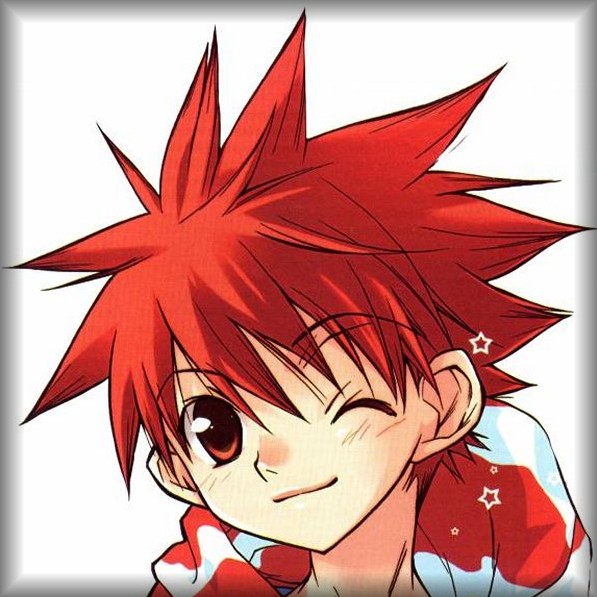
Umm ... ???
Isn't there already an ability in PICO to save more than 256-bytes of persistent data via CSTORE ?


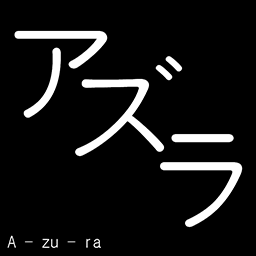
Technically you could, but if you want to update your Pico-8 application, the save data you have for one version will be lost in the next version since you're writing directly to the cart itself (unless you do cart swapping and save to another cart instead).
I meant to make these functions around the use of persistent data so developers can get more out of the persistent data's 64 value limit; it's a given that these functions can only go so far if a developer legitimately needs more than 2,048 bits/256 bytes for their own save functionality.


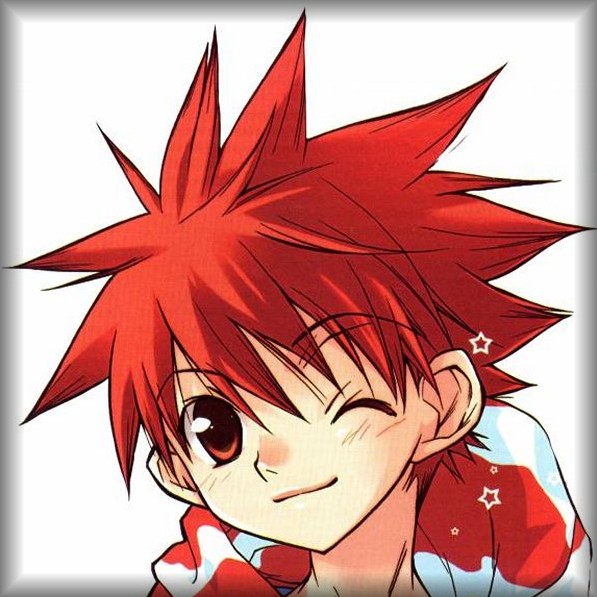
Since a notepad program I'm working on will definitely need more than 256-bytes of data for storage of text, I will certainly be experimenting with CSTORE.
Perhaps there is an easy method of persistent data despite being a different program altogether. I'll look into it.
Curiously I wrote a comprehensive bit-to-byte program that saved values for small data on the Gameboy as it only had 16k SRAM.
I labeled the elements Coin, 4D, 8D, 16D, 32D, 64D, 128D, BYTE, WORD, and LONG.
Coin being HEADS or TAILS (boolean, 1-bit).
4D value 0-3, 2-bits
8D value 0-7, 3-bits
16D value 0-15, 4-bits
32D value 0-31, 5-bits
64D value 0-63, 6-bits
128D value 0-127, 7-bits
BYTE value 0-255, 8-bits
WORD 2-bytes, 16-bits
LONG 4-bytes, 32-bits
It would retrieve or set these values by reading and writing individual bits.
Nice to see someone writing similar code for PICO.
Your CC4-BY-SA contract I believe is the standard agreement for all uploaded carts, so I wouldn't worry about it. :)


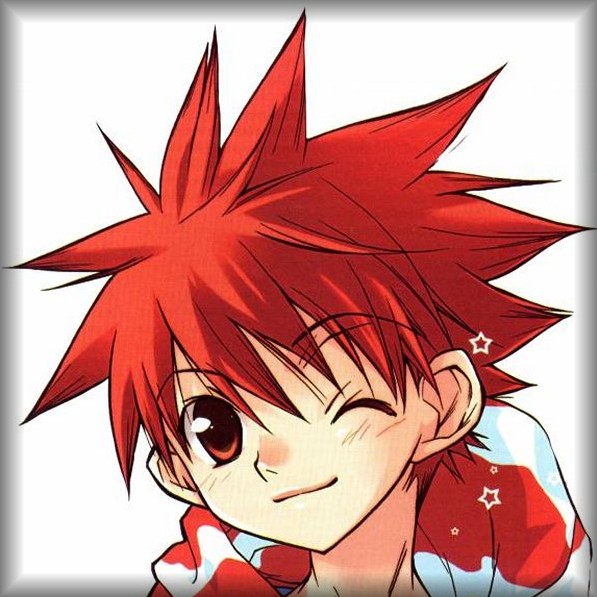
My notepad program is complete (well, almost). But you can see it uses quite a bit more than just 256-bytes, a nice 8192 total and it doesn't touch your image tiles, mapper, audio, or music. You can find that fully working cart w source HERE:
[Please log in to post a comment]