Hello, I decided to make a post because I have a couple of programming questions on how to execute or fix up particular things. As I mentioned in the thread title, I am a complete novice when it comes to programming so a couple of these questions might be dumb or excessive or a little hard to convey.
Weeks ago, I got a Pocket CHIP, and been currently enjoying trying out all the games, and trying to put a game together of my own for the first time ever. I’m currently putting together a game with my girlfriend as a player character (serving as the showoff motivator), which I do intend to just share the whole thing with her only. Maybe I’ll try the challenge of re-skinning the mechanics to publish to the site, though I thought I might be getting of myself there, haha. The game is a one-stage shooter, trying to fend off enemies as long as possible. Something relatively simple and not too crazy. I got the assets set, got my sound effects, tunes and learned some of the core basics through the Squashy tutorial in fanzine #1, and managed to a few of the basic parts working. The main problems lie with assembling it altogether and it feels so very daunting.
Since the resources for pico-8 is relatively outside of studying the game code (which 98% of is over my head), there’s a few parts I couldn’t find concrete answers to, and hoping you guys will give me a step in the right direction or point out which of the four fanzine’s addresses my problem, which I could’ve overlooked.
Here are the main things I want to resolve:
~Button input at specific moments~
This has been driving me nuts the past few days. So basically, the game will have multiple screens where the layout is essentially titlescreen > excuse plot blurb > main game > game over (then back to titlescreen). I set the titlescreen at the _init function and btn(4) to advance the screen. I used this wikia entry (http://pico-8.wikia.com/wiki/Multiple_States_System) for the multiple screen idea and managed to setup drawing the different screens.
Here lies my problem. Btn(4) is used for multiple things and it seems to be interfere with one another. I want it to be used for both advancing the screen and as the shooting button, but it seems to do one thing only or tries to do all at once. For instance, I played around with the setup where if I btnp(4) it suppose to go to the next screen. I did the if statement twice and used an and indicator to specify which screen btnp(4) goes to depending on mode I’m in. It either skips the second screen and jumps straight to the game while firing a single bullet or does not advance from the second screen.
I haven’t had luck trying to remedy the solution. I wonder if there is some way to assign a button for multi-purpose, depending on what part of the game I’m at, while disabling the other instances of btnp(4) in a function. Maybe it’s something to do with having all the other functions in the _update function screwing around.
~Bullet Limit~
I managed to construct a bullet via the dom8verse tutorial in fanzine #3 so the shooting itself is ok thus far. I didn’t implement the hitbox yet so hopefully that will go ok.
Only minor issue is that if I hold the button while in one place or strafing left to right, it’s a rain of rapid bullets. Makes things potentially too easy. I wonder if there is a way to limit the number of bullets that shows on the screen at once. Say, I want only 3 to appear at the screen at one time. Is there a way to manipulate that?
~Random enemy spawns~
I couldn’t find some info on it that breaks it down, and didn’t attempt it yet. I presume that will be the most challenging aspect. The way I envision it, there are two enemy sprites (with a simple 2 frame animation), and would spawn somewhere around y=128ish axis while their x position is random. What would be a good code to first determine which sprite gets picked then pick out where in the x axis they come from.
For movement, I’ll try to study up and tweak the pong ball movement to mess with walking patterns so hopefully that will turn out ok… I hope. Then there’s figuring out collision detection with moving targets which I did not really look into just yet.
Optional bonus:
~Saving high score and name input~
I thought it would be a neat idea to do that, but do not know how to, well particularly inputting letters in x strings. I’d like to know how to do that, but not really a big deal the parts mentioned above are a much bigger deal.
It’s probably a bit much to ask at once since this seems to be practically building a game. I apologize about it. I just dunno how to tackle all this since I’m an absolute beginner, relying on a few scattered cheat sheets, and can’t seem to find the solutions on my own. The concept seemed relatively simple in my head, but actually attempting it and realizing some of the complexities, guess I went a little over my head with all of this. Thank you very much for your time guys.


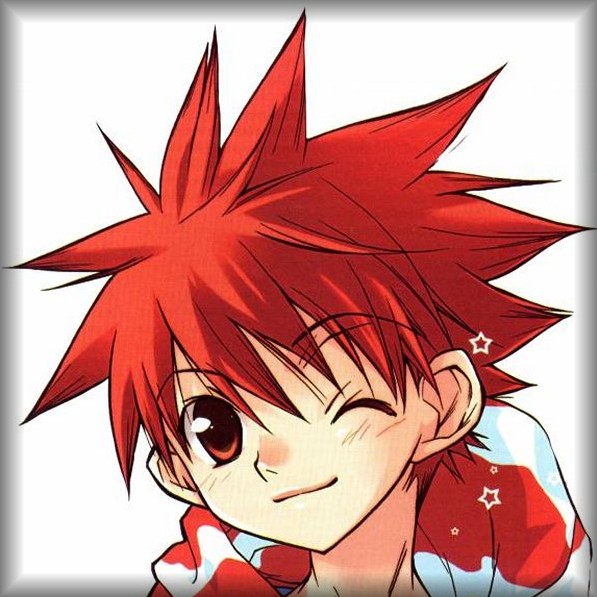
Mister J, may I recommend you take a look at the HELLO program included with PICO-8. Running that program and looking at the source answered several of my questions as for how to get started.
I'm still learning too. :)



Button input at specific moments: It sounds like you have the right idea and just have a bug in your conditional code. Something like this should work:
function _update() if mode == 0 then if btnp(4) then mode = 1 end -- (other mode 0 updates go here) elseif mode == 1 then if btnp(4) then -- (shoot bullet) end -- (other mode 1 updates go here) end end |
Bullet limit: This is just a matter of checking the number of active bullets on the screen when deciding whether to start a new one. A typical way to implement bullets is to track the location of each bullet in an entry in a Lua table. If you do it this way, you can just count the number of entries in the table for the check:
bullets = {} function _update() -- ... if btnp(4) and #bullets < 3 then add(bullets, {player_x, player_y}) end for bullet in all(bullets) do -- bullet moves up 3 pixels per frame bullet[2] -= 3 -- (test for bullet collision here) if bullet[2] < 0 then del(bullets, bullet) end end -- ... end |
Random enemy spawns: I can't tell if you're asking about how to determine where the enemy spawns or when the enemy spawns. You want to use the rnd() function. For something like a pixel location, you want to use flr() on the result to make it an integer. For example, to select a random number between 0 and 127 for the x coordinate:
enemy_x = flr(rnd(128)) |
Saving high score and name input: For saving data between sessions, look into the cartdata feature. For implementing high score name/initials entry, you'll want to create a string of all the possible letters, then use sub() with a number to select the letter that corresponds with the number (and change the number based on user input). You can just store the number for each letter, then refer to the lookup table whenever you want to print the letter.
General advice: You are correct that this is a lot to take on at once. Just get one thing working at a time, and keep things simple.
Good luck!



Sorry for the late post. Been busy with other things to make much progress.
-dw817-
I checked out the Hello World demo again. It didn't help out with the current scenario, but I learned some little things, and it's additional things I can keep in mind for the future.
-dddaaannn-
Thank you very very much for the breakdown. Yeah, it is best for get one thing working at a time, and keep it simple. Been doing the former, and I only mentioning all the troubled parts or concerns to keep it in one thread instead of continually making new ones.
For the results, I tried the setup with the different modes, and for some reason, ends up freezing the game if I go to another screen. For now I will work around and assign other buttons, and hope I'll get it right one day for a multi-purpose button. I managed to limit the bullets, but on the 2nd part of the code (the for all) I seem to get some error resulting in nil value when I shoot. Maybe I'll reconstruct the shooting stuff.
And sorry I wasn't clear on the third part. I'd like to know how to time the random times and for the code you gave me, I got the idea of it. Just need to figure out how to assign sprites to it.
Again, thank you so much for your time with this. I'm very slowly working on it. Besides not that much time, the errors and troubleshooting is a bit of a motivational killer so far. Definitely a learning experience.



I can't post anything too involved at the mo as I'm on my iPad but I did want to chip in and suggest a good place to start with learning would be the "picozines"
https://sectordub.itch.io/pico-8-fanzine-1
They have guides and examples with code, will be a lot easier/more useful than trying to deconstruct existing games at least to start with. Diving into existing carts can be pretty intimidating I've found, until you have a bit of an idea what is going on!
Also, so peeps have a baseline of where to pitch advice, what (if any) programming know how do you have already? Do you understand the various loops, use of functions, arrays/tables, object orientated ... ?



I pretty much little very programming experience. I only did visual basic for a few months, and that was a couple of years ago. Thus far, I got the basic idea of some commands, functions, loops, a few of the basic pico-8 functions and quirks and basic understanding of tables and for loops, but not how to utilize and implement them. I'll be a pain about this whole thing, haha.
Yeah, I did the first three tutorials on fanzine 1. Squashy was a great breakdown of the fundamentals of the program and I tweaked numbers around to see what does what. I'll slowly do the other tutorials while i'm cherry picking some sections on learning some of the mechanics I'm interested in implementing. I need to slow things down a little and figure out a method or thought process that works best for me. It just got tempting to try a project already when I was drawing assets for the fun of it, and felt compelled to already try assembling it.
And on a random note, at least I fixed up my button issue. I looked occasionally arts and see if I can find a code I'm able to understand. For the screen switching, I understood how Wizardish implemented it and replicated it for my own. Progress is progress.
[Please log in to post a comment]