
I'm working on a bullet pattern function that shoots out bullets in a direction, at a speed, etc...nothing fancy and nothing new to me, all my games have bullets! BUT this time some bullets are magically moving mid-stream and I can't figure out why.
See here...
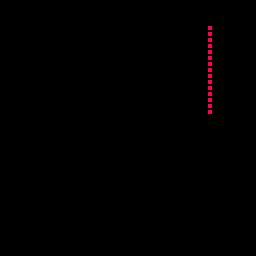
I've narrowed it down to happening when bullets from the line ahead of it get deleted from the table. When a bullet goes off screen, I'm removing it from the table so as to not get overloaded and so on. Normal stuff. But when that deletion happens, the next bullet in the table magically moves out of line yet all the rest follow orders.
If there are 15 bullets in every line, why would the 16th bullet move when the ones ahead of it get deleted? Is it something to do with table order? Or some process not happening due to deletion time?
It's like the addition math gets skipped at that moment. Very weird...but also something I'm sure that is very simple I'm overlooking. Lord knows I've spent way too much time on whittling this down the simplest version and I'm not sure how to refactor any more. A man can only bang his head so much :)
Any thoughts, insights, or suggestions are appreciated.
Code for the example above.
function add_bullet(x,y,a,s) local dx=cos(a)*s local dy=sin(a)*s add(bullets,{x=x,y=y,dx=dx,dy=dy}) end function bullet_update() for k,o in pairs(bullets) do o.x+=o.dx o.y+=o.dy if o.x>130 or o.x<-5 or o.y>130 or o.y<-60 then del(bullets,o) end end end function bullet_draw() for k,o in pairs(bullets) do rectfill(o.x,o.y,o.x+1,o.y+1,8) end end function _init() fr=0 bullets={} end function _update60() bullet_update() fr+=1 if fr==50 then -- this is here to repeating demo only for i=1,15 do add_bullet(120,10+3*i,.5,1) end fr=0 end end function _draw() cls() bullet_draw() end |



classic ‘del’ in array while iterating over the collection
that’s ‘undefined’ behavior (in practice - it usually skips a item)
use all() or for i=#bullets,1,-1 do … deli(bullets,i) … end to get correct behavior



Dammit...I didn't think about all() instead of pairs() - I guess I don't make much distinction between the two. I think I just default to pairs() so if I need the key I have it.
Thanks, Fred. Appreciate your experienced insight as always. Sorry it wasn't more challenging ;P
[Please log in to post a comment]