Hello all! I'm having an issue with a game I'm developing, where the collision is working flawlessly with any side other than the left side. for some reason it just wont work with the warps in game and I'm not sure why. left side collisions are working everywhere else, namely with stopping the player walking thru the walls. but not for the warps in game, which are supposed to work kind of like Pokémon's teleporting system. Frankly, I'm stumped and would appreciate if someone could take a look at this. Linked are not only the relevant code in the game, but a test cartridge as well to see what the problem is.
the main files i believe the bug should be happening in is input.lua, collision.lua, and game_manager.lua
collision.lua
--collsion for the map function collide_map(obj,aim,flag) --obj = table needs x,y,w,h --aim = left,right,up,down local x=obj.x local y=obj.y local w=obj.w local h=obj.h local x1=0 local y1=0 local x2=0 local y2=0 if aim=="left" then x1=x-1 y1=y x2=x y2=y+h-1 elseif aim=="right" then x1=x+w-1 y1=y x2=x+w y2=y+h-1 elseif aim=="up" then x1=x+2 y1=y-1 x2=x+w-3 y2=y elseif aim=="down" then x1=x+2 y1=y+h x2=x+w-3 y2=y+h end --pixels to tiles x1/=8 y1/=8 x2/=8 y2/=8 if fget(mget(x1,y1), flag) or fget(mget(x1,y2), flag) or fget(mget(x2,y1), flag) or fget(mget(x2,y2), flag) then return true else return false end end |
game_manager.lua
--manages all overarching functions and mechanics --ex: turns --turn structure turns = { turn = 0, moves = 4, actions = 2, end_moves = false, end_actions = false } --moves player with given direction function move(dir) --if moving left and not colliding with walls if turns.moves > 0 and dir == "left" and not collide_map(p, dir, 1) then p.x -= 8 turns.moves -= 1 printh("col: "..tostring(collide_map(p, dir, 3)), "log", false) --check if colliding with left side warp !NOT WORKING! if collide_map(p, dir, 3) then -- move map printh("moving map left", "log", false) _map.last_x = _map.x _map.x -= 1 printh("x:".._map.x, "log", false) p.x = 120 end --if moving left and not colliding with walls elseif turns.moves > 0 and dir == "right" and not collide_map(p, dir, 1) then p.x += 8 turns.moves -= 1 --check if colliding with right side warp !WORKING! if collide_map(p, dir, 3) then -- move map printh("moving map right", "log", false) _map.last_x = _map.x _map.x += 1 printh("x:".._map.x, "log", false) p.x = 8 end elseif turns.moves > 0 and dir == "up" and not collide_map(p, dir, 1) then p.y -= 8 turns.moves -= 1 elseif turns.moves > 0 and dir == "down" and not collide_map(p, dir, 1) then p.y += 8 turns.moves -= 1 else if turns.actions > 0 and turns.moves <= 0 then turns.end_moves = true elseif turns.actions <= 0 then turns.end_actions = true end end end function end_turn() turns.turn += 1 turns.moves = 4 turns.actions = 2 turns.end_moves = false turns.end_actions = false end function draw_turns() if turns.end_moves then print("out of moves", 32, 0) elseif turns.end_actions then print("out of actions", 32, 0) end print("turn: "..turns.turn, 0, 0) print("moves: "..turns.moves, 0, 8) print("actions: "..turns.actions, 0, 16) end |
map.lua
-- map functionality, using the x and y in _map to determine which room the player is in _map = { x = 0, y = 0, last_x = 0, last_y = 0 } --draws the map function draw_map() local x = 0 local y = 0 if _map.x ~= 0 then x = (_map.x - _map.last_x)*(_map.x+15) end if _map.y ~= 0 then y = (_map.y - _map.last_x)*(_map.x+15) end printh("draw_x:"..x, "log", false) map(x, y) end |
input.lua
--input variables input_frozen = false --input function --gets input for all buttons in the game and provides logic behind them function input() if btnp(5) and not menu.menu_opened then open_menu() elseif btnp(5) and menu.menu_opened then close_menu() end if not input_frozen then if btnp(0) then move("left") end if btnp(1) then move("right") end if btnp(2) then move("up") end if btnp(3) then move("down") end if btnp(4) then end_turn() end end end |
player.lua
--player class, contains all information relavent to the player character --structure containing all variables relevant to the player p = { sp = 1, x = 64, y = 64, w = 8, h = 8 } --draws the player to the screen function draw_player() spr(p.sp, p.x, p.y) end |
menu.lua
--menu class containing all logic for the in game menu --structure containing all variabkes relevant to the menu menu = { menu_opened = false } --opens the menu function open_menu() input_frozen = true menu.menu_opened = true end --closes the menu function close_menu() input_frozen = false menu.menu_opened = false end -- draws the menu function draw_menu() cls(0) map(112, 48) print("menu", 59, 6) end |
main.lua
--default functions draw, update, and init function _init() --setting player p.sp = 1 p.x = 64 p.y = 64 --resetting menu and input menu.menu_opened = false input_frozen = false end function _draw() if menu.menu_opened then draw_menu() elseif not menu.menu_opened then cls(3) draw_map() draw_player() draw_turns() end end function _update() input() end |
here is a test cartridge to see the issue at play
controls:
left: move left
right: move right
up: move up
down: move down
x: open menu
z: advance turn
to recreate the bug, you can walk through the warp on the right of the screen from spawn (orange arrows)
but when you make it to the other side, you cannot warp back even though the logic should let you.
thanks in advance for the help. I know the code is not as great as it could be, I'm relatively new to lua and pico-8 in general. thanks again!


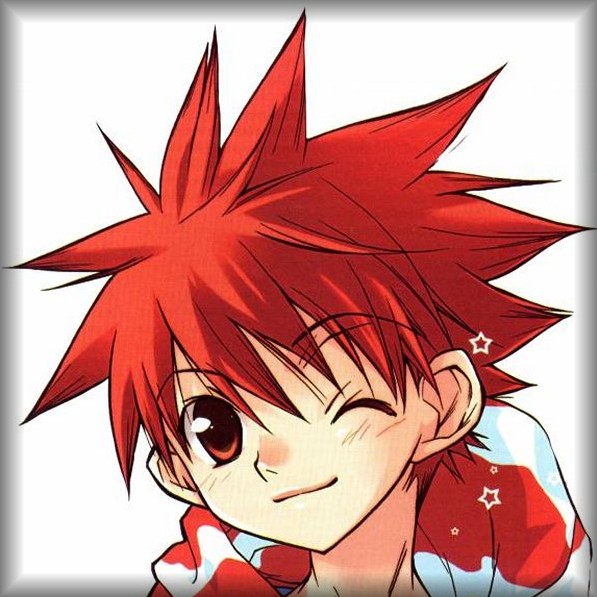
Actually, @alpacus, I am running into collisions with the grass - so there is a problem existing.
I will give you a hint. The easiest way to handle a collision is to let the X+Y coords touch the obstacle. If is atop an object, then set X+Y to last X+Y easily accomplished via:
x2=x y2=y { move X+Y } if { collision of X+Y } then x=x2 y=y2 end |
[Please log in to post a comment]