Aw man, I'm so sorry, I feel really silly... How on earth do you generate a random bool? I can't find any way to cast an int into a bool, so I'm down to doing the sort of stuff below:
randbool=flr(rnd(2)) if randbool==0 then randbool=false else randbool=true end |
This feels really dirty and silly. What's the established way of doing this please? And apologies in advance.
Thanks,
Pedro.



The most concise way I know is:
randbool = rnd() < 0.5 |
or you can do the following, but it takes more tokens and virtual CPU time because it creates a table of two true and false elements each time.
rndbool = rnd{false,true} |
You can avoid the wasted virtual CPU time by declaring a global:
bools = {false,true} rndbool = rnd(bools) |
but now this takes even more tokens.


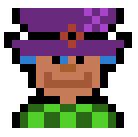
Yes that's the way to do it.
You can also do:
randbool = rnd()<.5
which is a bit shorter



Yes! You posted your message as I was editing my post to simplify my original code to be the same as yours. :-)


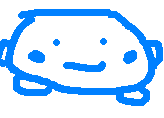
the best solution was shown in previous posts: if you need a boolean (true or false) and you have an expression that evaluates to boolean (like collides(player,other)
or rnd()<0.5
), then use that boolean directly!
but for cases when you need to check a boolean then assign something else (like strings or numbers), there is a more convenient way than your initial if/then/else/end:
message = thing>2 and "string if true" or "string if false


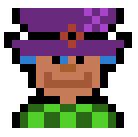
Just because we are at it, that last thing @merwok showed is called "ternary" operator in other languages.
num = x==y and 1 or 0
I discovered recently that you can chain these as much as you want
num = x==y and 1 or x>y and 2 or 3
Which means, if x==y then num=1. if x>y then num=2. Else num=3


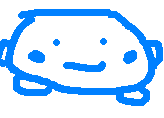
technically it is not quite a ternary operator, but the combination of two binary operators. I learned it as «the and-or trick».


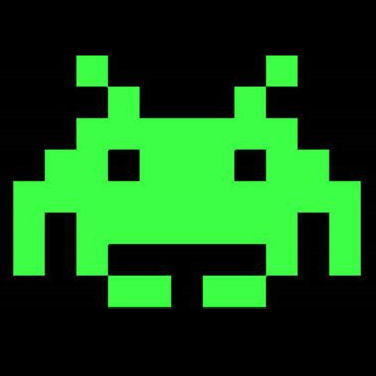
Can anyone explain how the "ternary operator" works in Lua (note I understand how it works in other languages)
Given the simple example:
num = x==y and 1 or 0 |
If x and y have the same value, the statement becomes:
num = true and 1 or 0 |
Why does this assign 1 to num ? I would have thought num would be assigned "true"


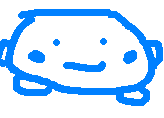
it’s explained here: https://www.lua.org/pil/3.3.html
in short: the expression evaluates to true and 1 → 1
or false or 0 → 0
[Please log in to post a comment]