This is an implementation of an algorithm that plays snake very well. It's based on a path through all board cells and calculated shortcuts to get more efficiency.
Controls:
X - show/hide the path
Menu items:
ALG - controls which variant of the algorithm is used.
SPEED - Snake's speed, lower = faster.
SNAKE - Change snake visualisation.
The path was generated based on a Hilbert curve. It would work with any path that goes through all board cells, but the Hilbert curve has some nice properties and looks.
I have implemented two variants of this algorithm here, togglable in the menu. "Tail" algorithm tends to follow it's tail very closely when it can't get directly to food. "Blocky" algorithm curls on itself until it has a clear path to food resulting in big patches of snake body later in the game. I believe "Tail" is more efficient than "Blocky".
Algorithm explanation:
My explanations tend to be all over the place, so if you are confused about anything feel free to ask!
Inspired by: Code Bullet (YT)



This is really neat. I'm sure you're right that the "Tail" algorithm is more efficient, but I find the "Blocky" algorithm quite mesmerising to watch. Really pumping music, too.
Would there be a lot of work to draw the snake body with shaped segments so we can see just how it's filling the space?


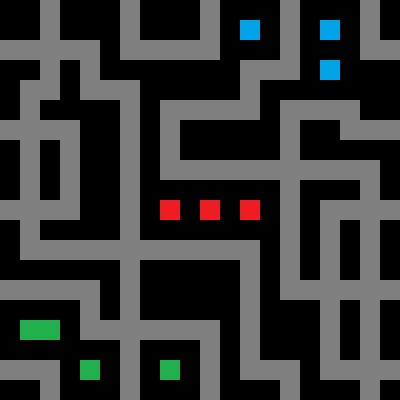
I think it would actually be a bit hard. Currently the snake is stored as a list of cells without any info on their orientations, and is drawn as a series of squares. The game code comes from a speed-coding challenge I had with a friend (I got a time of 3:34) so it's not pretty or very efficient. It's deffinitely possible and would make the visualisation way better.
Edit: It was way easier than I thought! If I have a list of body segments I can draw the snake line as a series of rectangles between the segments. The 'outline' is the same, but the rectangles are large and light blue and they overlap a lot. No need for checking orientation and still 0 sprites used!



@olus2000 : Thank you, that makes it look even neater, especially to show what the "Tail" algorithm is doing, and how the layout constantly shifts once it's mostly full. I'm glad to hear it wasn't too much work.
Thank you also for the time put into explaining it. It all seems to make sense.


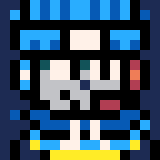
"path through all board cells"
did you mean "hamiltonian cycle"


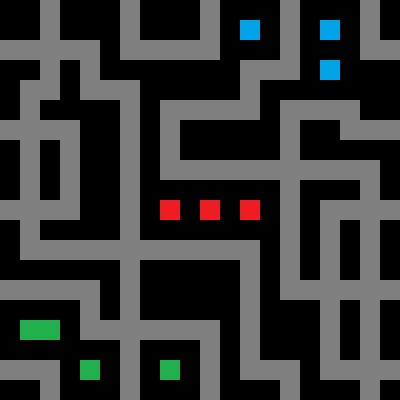
@Nbrother1607 yes, what I meant is called a Hamiltonian cycle.
I made the music myself, you can find it on my youtube page which is otherwise inactive for now.
@WoopyBoiii This was in fact inspired by CodeBullet's snake AI videos :D
[Please log in to post a comment]