I wanted to see if using the sprite drawing functions would be any faster than using lines and rectfills, so I made a single reference sprite containing all the 2x2 pixel configurations and used that to draw the image. There's really no noticeable change in performance, but now I can change the reference sprite into random garbage and get weird distorted mosaic effects. Press X to check them out!
Also added some comments to my code, fixed a bug that caused the background color to extend one horizontal pixel too far, and added the option of drawing the image's background color as transparent if a negative number is given as the function's background color argument.
The sprite memory in PICO-8 can get a bit cramped, so I wanted to try and see if I could implement 1bpp sprites. The idea is basically that each pixel color corresponds to a specific 2x2 pixel configuration. It's not very well optimized, as it more or less draws each pixel out individually. Furthermore, it would probably need another tool to convert hand-drawn single-color sprites into the proper format (I plotted the test image in by hand, because I am legit dumb. It's a screenshot from Tetsuo: The Iron Man.)
CONTROLS:
Arrow keys to pan the camera around.
Fire 1/Z to crop the image into a random size.
I mostly implemented these to see how it would run at different sizes.
Here are the pixel configurations that each color value represents.
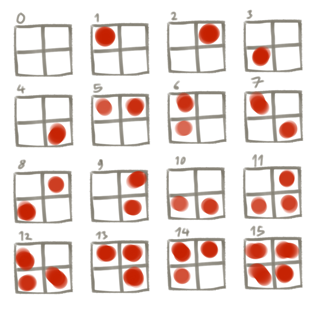



Cool. :)
I made a small processing program to create the 1bit images.
Just change the image name, run the sketch, set the threshold by moving the mouse left/right, and click when you feel the image looks right.
The data is copied to the clipboard (or if not, you can copy it from the console window).
Paste it over the first 64 lines of the gfx data in the cartrigde from this thread, and you can enjoy your low-res, low-color picture. :)
Processing code follows:
import java.awt.;
import java.awt.event.;
import java.awt.datatransfer.;
import javax.swing.;
import java.io.*;
PImage img, dimg;
StringDict converter;
void setup() {
img = loadImage("jaybob128.jpg");
size(img.width, img.height);
dimg = createImage(img.width, img.height, RGB);
converter = new StringDict();
converter.set("0000","0");
converter.set("1000","1");
converter.set("0100","2");
converter.set("0010","3");
converter.set("0001","4");
converter.set("1100","5");
converter.set("1010","6");
converter.set("1001","7");
converter.set("0110","8");
converter.set("0101","9");
converter.set("0011","a");
converter.set("0111","b");
converter.set("1011","c");
converter.set("1101","d");
converter.set("1110","e");
converter.set("1111","f");
}
void draw() {
dimg.copy(img, 0,0,img.width,img.height,0,0,img.width,img.height);
dimg.filter(THRESHOLD, (float)mouseX/(float)width);
image(dimg, 0, 0);
}
void mousePressed() {
println("Saving...");
println("123456789.123456789.123456789.123456789.123456789.123456789.1234");
String simg = "";
int counter = 0;
dimg.loadPixels();
for(int y = 0;y<dimg.height;y+=2) {
for(int x = 0;x<dimg.height;x+=2) {
String s = "";
s += brightness(dimg.pixels[ydimg.width+x])>0?1:0;
s += brightness(dimg.pixels[ydimg.width+x+1])>0?1:0;
s += brightness(dimg.pixels[(y+1)dimg.width+x])>0?1:0;
s += brightness(dimg.pixels[(y+1)dimg.width+x+1])>0?1:0;
simg += converter.get(s);
counter++;
if(counter==img.width/2) {
for(int i = counter;i<128;i++) {
simg += "0";
}
simg += "\n";
counter = 0;
}
}
}
StringSelection data = new StringSelection(simg);
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
clipboard.setContents(data, data);
println(simg);
}



Dang, very nice! That's way cleaner than whatever it is that I was thinking of doing.
[Please log in to post a comment]