I was making a game and i don't understand how collision works thanks.
EDIT: I have read the collision demo and i was wondering if there is a less usage way to do that
I don't want to loose all my tokens.


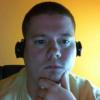
If you're just trying to do collision with map tiles, it doesn't have to be complicated at all. Set a flag on the sprites used for collisions, and use something like:
function collidemap(fx,tx,fy,ty) local a=fget(mget(fx/8,fy/8),0) local b=fget(mget(fx/8,ty/8),0) local c=fget(mget(tx/8,ty/8),0) local d=fget(mget(tx/8,fy/8),0) return a or b or c or d end function playerupdate(p) local c local lx=p.x -- last x local ly=p.y -- last y if(btn(0)) p.x-=1 if(btn(1)) p.x+=1 if(btn(2)) p.y-=1 if(btn(3)) p.y+=1 c=collidemap(p.x,p.x+7,p.y,p.y+7) if(c) p.x=lx p.y=ly -- collision detected, move back to where you were end |
Note that it doesn't have to be predictive - you can allow the player to move into the wall, then move them back out of it, without it ever being noticeable because them moving into the wall and then back out of it happened in between drawn frames (in other words it all takes place before a draw is made, so they won't appear to be 'bounced back out').
This is about 145 tokens, but someone might know how to make it even smaller.


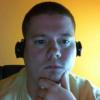
Here's a cart I made for you to demonstrate how to do it. You are free to copy the code and use it: here.
[Please log in to post a comment]