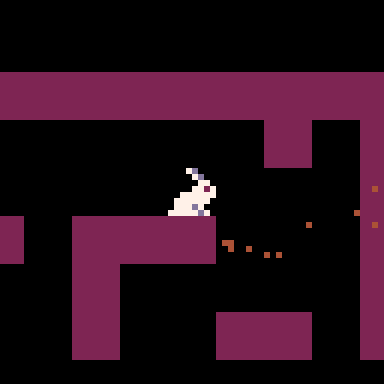
Hello everybody,
I'm trying to implement collision and knock-back(?) for the particles in my game. I've got it sort of working, but--as you can see from the gif above--some particles behave oddly. Some drip down the tiles, others get caught in mid-air.
I was hoping some kind soul could take a look at my code and tell me if there is a better way of going about this. I'm guessing my issue is a result of how I'm checking for collisions. Thanks!
parts = {} function make_px() for i=1,10 do p={} if not p1.left then p.x=p1.x+7 else p.x=p1.x end p.y=p1.y+7 p.c=4 if not p1.left then p.dx=1+rnd(2) else p.dx=-1-rnd(2) end p.dy=rnd(1)-rnd(.5) p.age=rnd(2) add(parts,p) end end function update_px() local g = .05 --check pixels left, right, above, and below. If there is a collision, add knock-back for p in all(parts) do if (fget(mget((p.x-1)/8,p.y/8),0)) p.dy*=-.5 p.dx*=-.5 if (fget(mget((p.x+1)/8,p.y/8),0)) p.dy*=-.5 p.dx*=-.5 if (fget(mget((p.x)/8,p.y-1/8),0)) p.dy*=-.5 p.dx*=-.5 if (fget(mget((p.x)/8,p.y+1/8),0)) p.dy*=-.5 p.dx*=-.5 p.x+=p.dx p.dy-=g p.y-=p.dy p.age+=.1 if (p.age>5) del(parts,p) --delete old particles end end function draw_parts() for p in all(parts) do pset(p.x,p.y,p.c) end end |

2

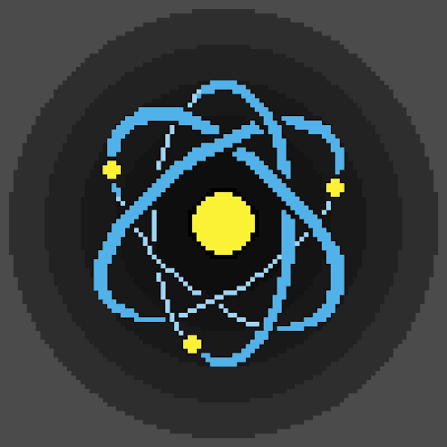
hers an improved approach function update_px()
local g = 0.05
for p in all(parts) do
-- Predict new position
local new_x = p.x + p.dx
local new_y = p.y + p.dy - g
-- Check horizontal collision
if fget(mget((new_x)/8, p.y/8), 0) then
-- Collided horizontally
p.dx *= -0.5
new_x = p.x -- revert horizontal movement
end
-- Check vertical collision
if fget(mget(p.x/8, (new_y)/8), 0) then
-- Collided vertically
p.dy *= -0.5
new_y = p.y -- revert vertical movement
else
-- No collision, apply gravity and move
p.x = new_x
p.y = new_y
p.dy -= g
end
p.age += 0.1
if p.age > 5 then del(parts, p) end
end
end


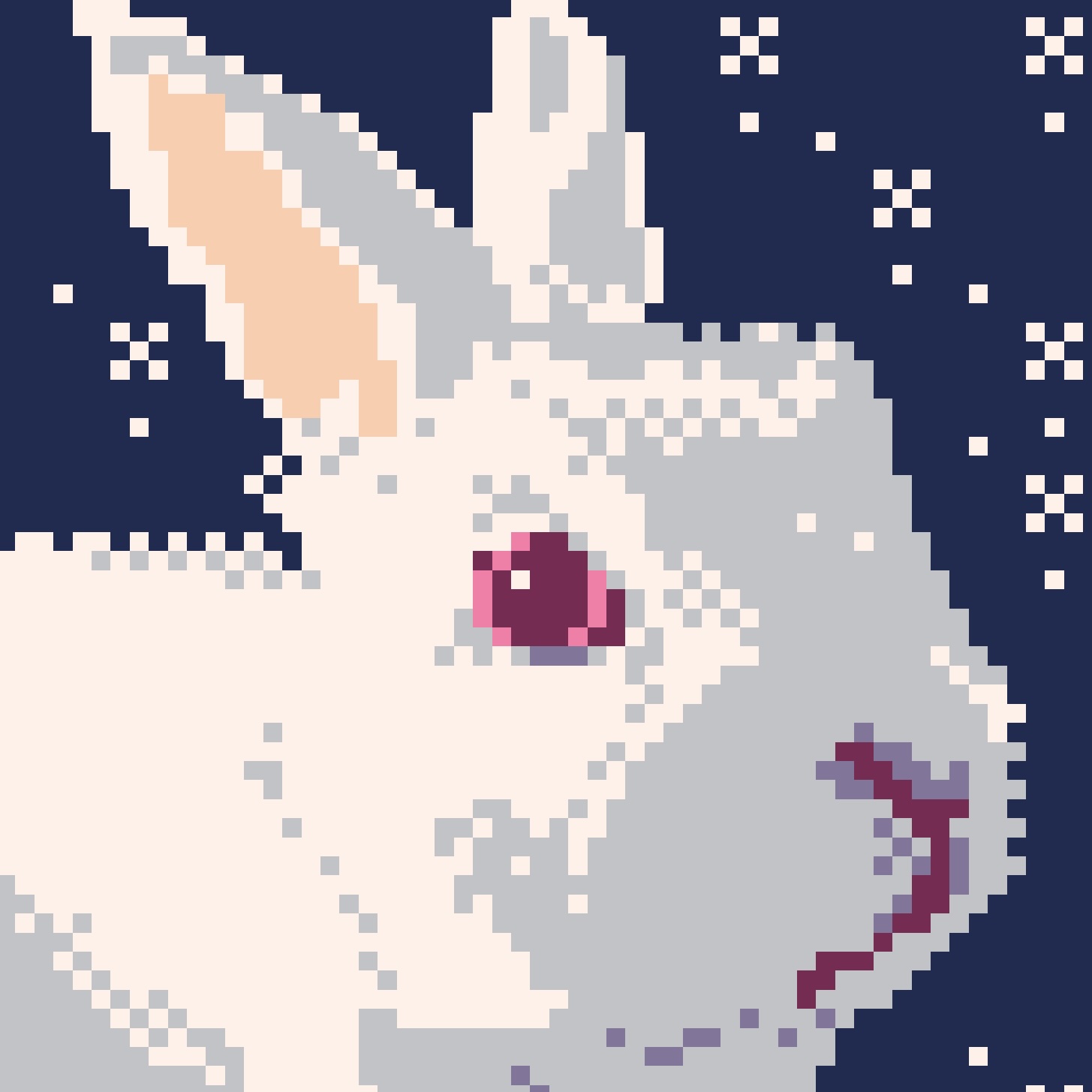
Thank you @AtomicPico! Works beautifully! Just had to make sure gravity was being added rather than subtracted.
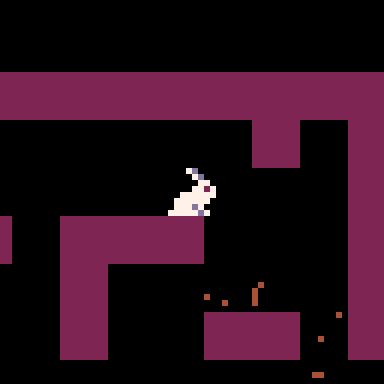
[Please log in to post a comment]