A question about dialogue boxes!
I'm new to both coding and Pico so bear with me, maybe there's no right answer but would appreciate suggestions.
Say you have a standard type of RPG game with a character who moves, and you approach or talk to an NPC, triggering a dialogue box on top of what's already on the screen (just like any old pokemon or dragon quest game). What would be an efficient way to do this? Load a new scene but don't clear the screen? Disable the player character's movement and actions while a list of text cycles through?
I've made a clunky textbox function (see image) but there must be a better way.
Any ideas or links to successful examples would be great.
Thanks in advance to anyone who answers!
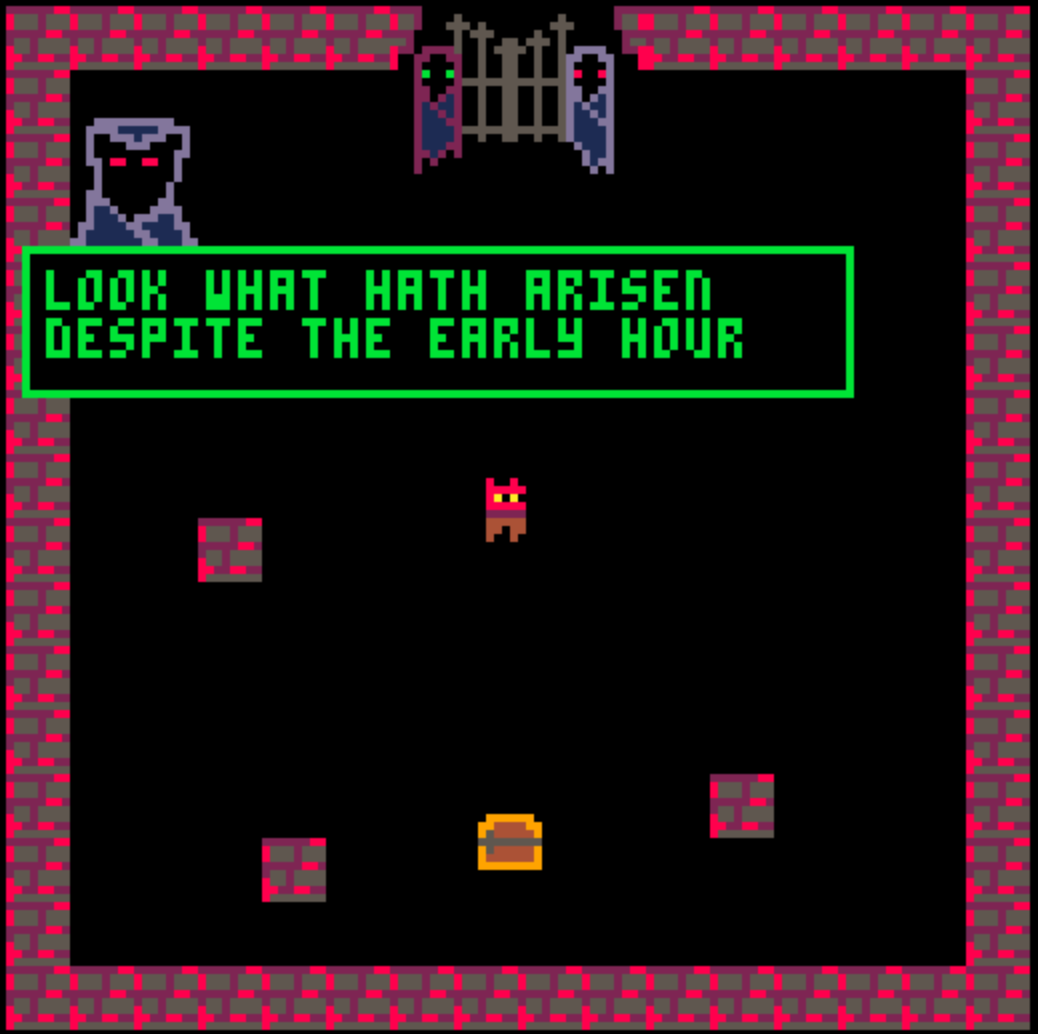


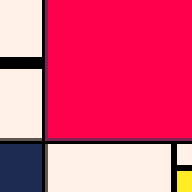
Here's one way you can consider to approach this.
Suppose your normal gameplay loop code looks like:
function game_update() -- usual gameplay update code... end function game_draw() -- usual draw code... end -- gets called from title screen function start_game() -- do some init... _update=game_update _draw=game_draw end |
You'd have some means of detecting of when you encounter an npc
function game_update() -- usual gameplay update code -- ... -- dpad left, for example if btnp(0) then -- some kind of npc detection code local npc=get_npc_at(player.x-1,player.y) if npc then -- not nil means there was an npc talk_to(npc) else -- normal move logic -- check for walls etc player.x-=1 end end -- etc... end |
That would cause a temporary switch out from the normal gameplay loop functions for dialogue handling
function talk_to(npc) dialogue = npc.dialogue -- a list of "things to say" d_step = 1 _update=dialogue_update _draw=nil -- no need to constantly draw at 30/60 fps dialogue_draw() end |
Those functions would be something like
function dialogue_update() if btnp(4) then d_step+=1 -- next chunk of speech if d_step>#dialogue then -- end of conversation -- back to the usual gameplay _update=game_update _draw=game_draw return end dialogue_draw() end end function dialogue_draw() game_draw() -- underlying game graphics as backdrop local ds=dialogue[d_step] -- data -- draw character "talking head" picture from packed spr args -- having this per step lets you move things around the screen -- or have a conversation involving multiple npcs spr(unpack(ds.spr)) -- dialogue textbox rectfill(ds.x0,ds.y0,ds.x1,ds.y1,ds.bgcolor) rect(ds.x0,ds.y0,ds.x1,ds.y1,ds.bordercolor) print(ds.text,ds.tx,ds.ty,ds.tcolor) end |



Thankyou Cakepie! Multiple npc conversation is what I was hoping to work out so that's great. This is all so much simpler than my code so far. Time for some revisions


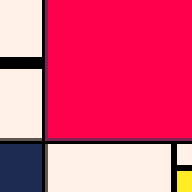
Glad to hear that was useful!
Do note that I've just edited the code to add an early return in dialogue_update to prevent an out of bounds error.



Another user posted a dialog library cartridge last year, this might be useful for you or give you some ideas for your own. https://www.lexaloffle.com/bbs/?pid=82185#p
[Please log in to post a comment]