Edit: not sure how I'm expected to display multiplication using the syntax here, but assume there's a X or star or whatever between 15 and i etc.
I was trying to initiate this statement here, which I can do:
for i=1,4 do
print(i, 15i-14, 15i-14, 14)
end
But then I wanted to move the diagonal array of 4 numbers with the arrow keys around the screen. I tried the code below but keep getting errors. I'm guessing my argument is setup wrong and I can't call i as the same variable in all these statements and make it work...? Can someone help me understand the immediate problems with the code, and then afterwards if viable suggest an alternative method of doing so. I'm still learning so my immediate concern is figuring out what is wrong here specifically.
function _init()
j= {
x=15i-14,
y=15i-14
}
end
function _update()
if btn(0) then j.x-=1 end
if btn(1) then j.x+=1 end
if btn(2) then j.y-=1 end
if btn(3) then j.y+=1 end
end
function _draw()
cls(0)
for i=1,4 do
print(i,j.x,j.y,14)
end
end
Even in the API demo I copy the code for the similar statment, but when i run it on its own it doesn't work. I don't understand why this here doesn't work on its own either:
cls()
for i=0,15 do
print(i,x,2,i)
x = x + 6 + flr(i/10)*4
end



Hi
The issue isn't really with the for statement - that's working fine. The issue is that the values j.x and j.y are computed once in _init, and aren't able to remember that they were computed from i. (in fact, your script won't even run, because when the code to create j is executed, nothing knows about i, so it doesn't know what value to compute)
There are a number of ways you could fix this, but perhaps the simplest is perhaps this:
j = { x=1, y=1 } function _update() if btn(0) then j.x-=1 end if btn(1) then j.x+=1 end if btn(2) then j.y-=1 end if btn(3) then j.y+=1 end end function _draw() cls(0) for i=1,4 do print(i,15*i+j.x,15*i+j.y,14) end end |
An alternative might be to define a function to compute the x and y positions for each i, perhaps something like:
j = {x=1,y=1} function posx(i) return j.x + 15*i end function posy(i) return j.y + 15*i end function _update() if btn(0) then j.x-=1 end if btn(1) then j.x+=1 end if btn(2) then j.y-=1 end if btn(3) then j.y+=1 end end function _draw() cls(0) for i=1,4 do print(i,posx(i),posy(i),14) end end |
Hope that helps!
--alex


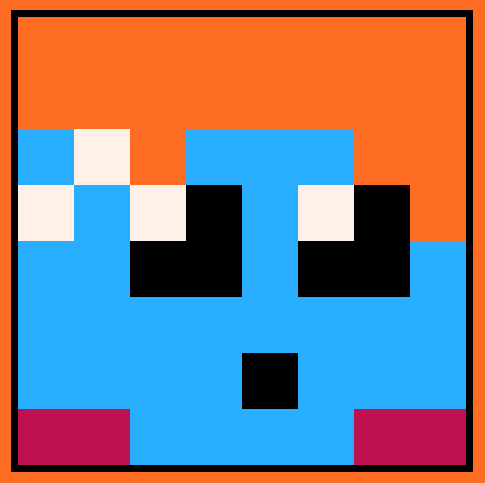
If I define i in it though shouldn't I be able to get the original code to work?
Like I see now the problem with:
cls()
for i=0,15 do
print(i,x,2,i)
x = x + 6 + flr(i/10)*4
end
Was as you described, a value wasn't defined before being used (x in this case). Setting x=0 fixes that though.
Can't I do the same with this code then:
function _init()
--assigning i a value in init this time
i=1,4
j= {
x=15i-14,
y=15i-14
}
end
function _update()
if btn(0) then j.x-=1 end
if btn(1) then j.x+=1 end
if btn(2) then j.y-=1 end
if btn(3) then j.y+=1 end
end
function _draw()
cls(0)
for i=1,4 do
print(i,j.x,j.y,14)
end
end
This generates an error though. If I change the for statement to an if statement where its only checking for a single number like:
i=1
if i ==1 then
print(i,j.x....etc
It works! So how...would I write that in similar manner to get it to work for a for loop if it works for a single number check if statement? Your code is the way I should have written it, but i'm trying to wrap my head around how things work still..especially this 'for' stuff its confusing me when or why I should use it. So I guess the question is how can I define it in init so that its able to do the calculations in draw as shown?
As well with:
cls()
x=0
for i=0,15 do
print(i,x,2,i)
x = x + 6 + flr(i/10)*4
Why is the flr(i/10)*4 calculation, something so complicated, necessary...? I get that it works fine without it when generating single digit numbers up to 9, and that its there to make sure the double digits display evenly space. But I don't get why if we're only dealing with i=10,15....why can't we just assign more space with something simpler like x+10? If we draw just i=10,15 all that's necessary is a x=x+10 for them to display properly. If we draw just i=0,9 then x+6 is all that's necessary. But if I make a setup like:
cls()
x=0
for i=0,15 do
print(i,x,2,i)
if i>10 then x=x+10
else
x = x + 6
end
end
the 10 and the 11 are wrong but everything else is fine....I don't get it. Using the flr(i/10)*4 fixes everything but I still don't get why.
[Please log in to post a comment]