




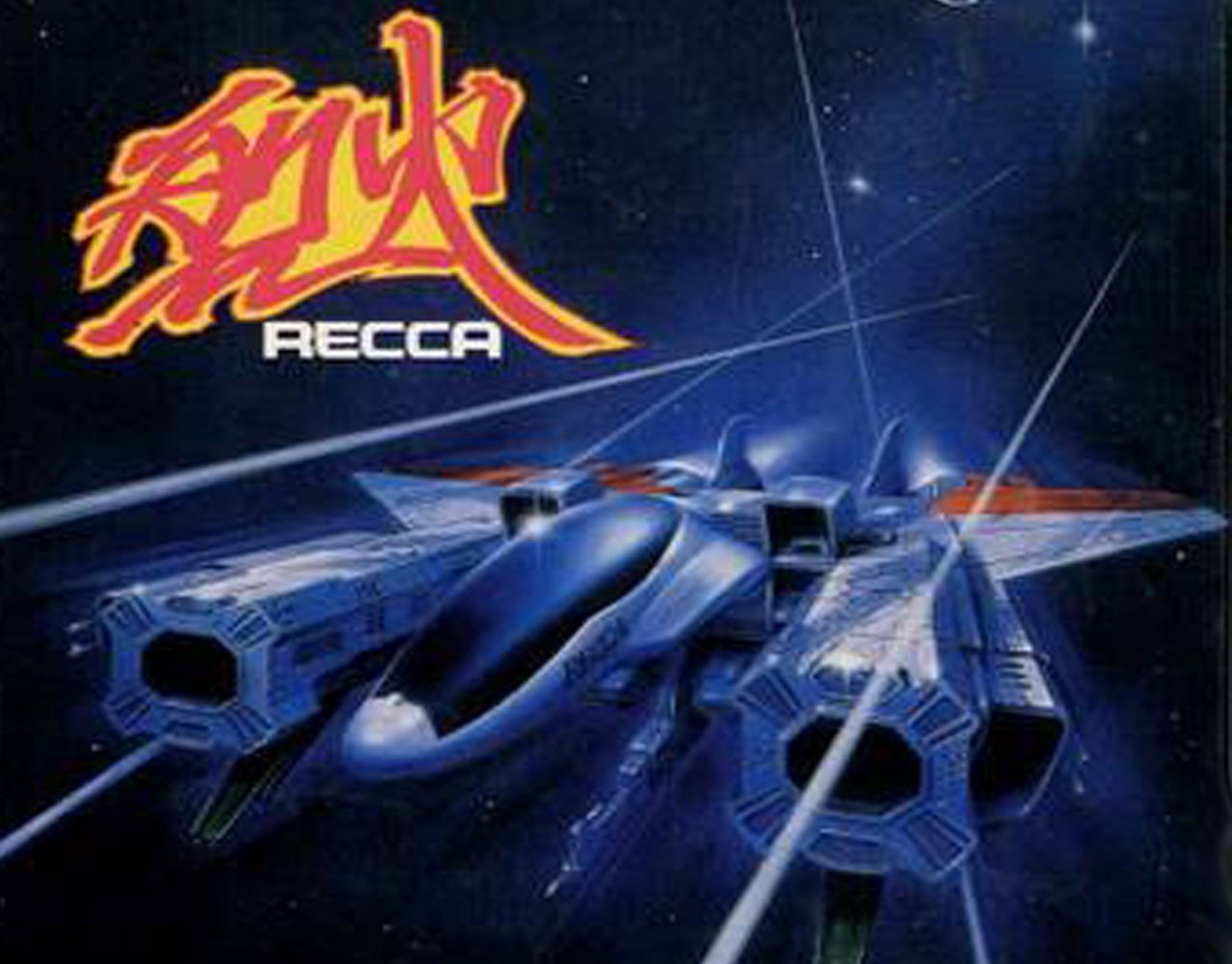
Thank you. This is really helping me understand the math/code behind making a cool vortex. Any possibility of some brief comments?



the idea behind the vortex is very simple, the code juste creates a spiral that goes up and down.
To begin, here is the code to draw a circle :
rx,ry = 20,20 //radius. change ry to 10 to have an ellipse ! cx,cy = 64,64 //position of the circle p = 10 //number of points function _draw() cls() for i=1,p do x = cos(i/p) * rx + cx y = sin(i/p) * ry + cy pset(x,y,7) end end |
Things can be more complex now. By experimenting, i found a code that makes a spiral.
First, we have to decide the number of "arms". We also create a usefull variable called "z", which now defines the radius (instead of rx, ry) and the angle (instead of i/p). I can't explain very well how it works but... it works ! here is a simplified version :
cx,cy = 64,64 //position of the circle p = 500 //number of points arms=7.2 //results are different if integer function _draw() cls() for i=1,p do z=i/arms //z is the radius and the angle x = cos(z) * z + cx y = sin(z) * z/2 + cy //divide z to have an ellipse pset(x,y,7) end end |
For the next step, we want the vortex to look like a wave. There must be a new variable, added to Y, that goes up and down. Let's create "h" (for height). To have a nice wave, we can use the cosine function, which "eats" a number between 0 and 1, and "poops" a number between -1 and 1. (pardon my language.)
a simple cosine wave looks like that :
for i=1,10 h=cos(i/10)*a //a is the amplitude of the wave end |
Applied to my code, it gives that :
cx,cy = 64,64 p = 500 arms=7.2 function _draw() cls() for i=1,p do z=i/arms x = cos(z) * z + cx y = sin(z) * z/2 + cy h = cos(i/p) * 5 pset(x,y+h,7) // h is added/substracted to y end end |
I hope it helped :)
[Please log in to post a comment]