Hello! I was working on a 2D platformer, and I was wondering about how to make enemies. The cartridge that is attached above (I hope it is attached) shows a small demo of the game thus far.
(NOTE: this is based off the Nerdy Teachers 2D platformer tutorial)
As you can see, there is collision, semisolid platforms and even some spikes that can kill the player however, I am focused on the "frog" on the middle left platform. So far I have gotten it to walk forward and change directions when hitting sprite 2 (left turns) and sprite flag 3 (right turns). But I cannot for the life of me get the frog sprite to flip when turning. More importantly I cannot get the frog to kill the player like the spikes do. If you have any ideas or advice about the enemies or just in general please crack open my code and take a look.
(NOTE: this is built off an older project of mine that I never finished, that is why there is code for "Glide" and "Slamming" and "Glidingdash" that seemingly does nothing and also why the thumbnail reads "Flemingo Quest")
P.S I am looking for a name for the little bird guy you play as, any ideas?
EDIT: Forgot to mention that the number follows the player it your current speed, used for debugging n' such
EDIT 2: After implementing the tuning code I am struggling with the frog killing the player so far I got this but
the death part does not seem to function properly.
function frog_update()
if collide_map(frog,"up",2) then
frog.turning=true
elseif collide_map(frog,"up",3) then
frog.turning=false
elseif frog.x == player.x and frog.y == player.y then
_draw=death_draw
_update=death_update
_init=death_init
end
end



It looks like your frog has a flp
variable but you're never changing it after initialization and you're not using it when drawing the frog like you are with the player sprite.
--game function draw_game() cls(12) map(0,0) spr(frog.sp,frog.x,frog.y) -- not using flp spr(player.sp,player.x,player.y,1,1,player.flp) -- using flp print(player.max_dx,player.x+-10,player.y+-10) froganim() end |
So you'll need to change that and then wherever you're detecting that the frog should change direction you'll need to update its flp
variable. So it looks like flp
should be true when the frog should be facing to the right and false when it should be facing to the left.
As for collisions with the frog: you seem to be checking map collisions for both the frog and the player but you're only checking map collisions. To get the frog and the player to collide you'll have to compare their positions to see if they overlap and then trigger the code which kills the player if they do.


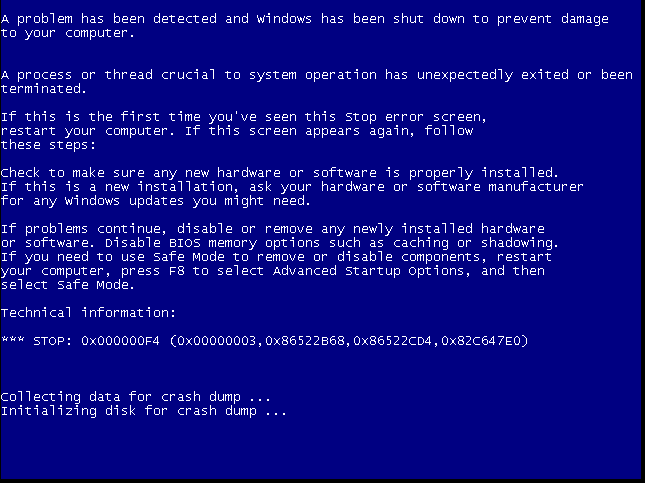
Thank you for all of your help! I am currently implementing your solutions now. I really appreciate it! Will post more updates later!


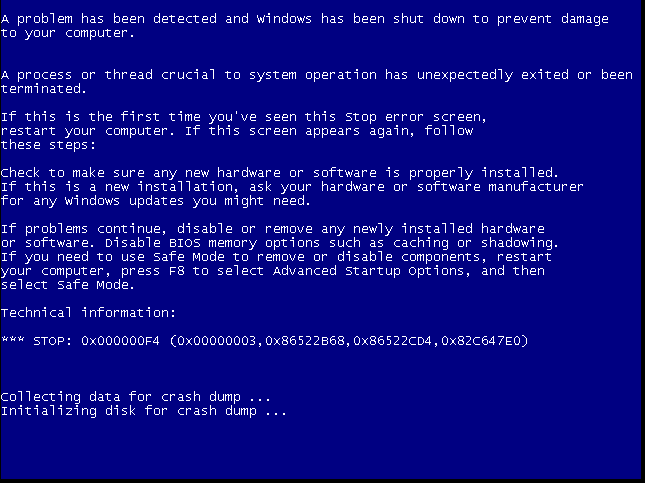
After implementing the tuning code I am struggling with the frog killing the player so far I got this but the death part does not seem to function properly.
function frog_update()
if collide_map(frog,"up",2) then
frog.turning=true
elseif collide_map(frog,"up",3) then
frog.turning=false
elseif frog.x == player.x and frog.y == player.y then
_draw=death_draw
_update=death_update
_init=death_init
end
end
Any Ideas?
Also is there any way to code it so the enemy disappears after you jump on it (i.e Mario) ?
Sorry for all of the questions, I am still relatively new.



"Sorry for all of the questions, I am still relatively new."
No need to apologize, we were all new once upon a time.
So technically, your frog/player collision code there actually will work it's just that it will only work if the frog sprite and bird sprite are aligned exactly/in exactly the same spot at the same time which is actually pretty unlikely so it will seem to not work at all or only work sometimes.
What you actually want to do is treat each sprite as an 8x8 bounding box and check if those boxes are overlapping. Here's some code that will do that:
function check_collision(o1, o2) return o1.x < o2.x + 8 and o2.x < o1.x + 8 and o1.y < o2.y + 8 and o2.y < o1.y + 8 end -- you'll call it like this somewhere in your code: check_collision(frog, player) |
Note that this code assumes that all your sprites are 8x8. If you're going to have different sized sprites you'll need to modify this code. This type of collision detection is called Axis Aligned Bounding Box (AABB). It's not the only way to detect collisions but it's one of the easiest.
To kill the frog by jumping on him:
First check for a collision between the two. If there is a collision check if the player is falling. If both of those conditions are true then you've likely collided with the frog from above so you can kill the frog. Otherwise the collision hits the player.
Hope that helps!



Oh! One more thing. You should probably break the frog/player collision code out into a separate statement. Something like this:
function frog_update() if collide_map(frog,"up",2) then frog.turning=true elseif collide_map(frog,"up",3) then frog.turning=false end if check_collision(frog, player) then _draw=death_draw _update=death_update _init=death_init end end |
The reason you want them separate is because as soon as one of the conditions in the if...elseif...
block is true all the others are ignored. So when your frog hits an edge and turns around it won't check for frog/player collisions at all until the next update. Having it as a separate if
statement means it will always be checked even when the frog is turning around.


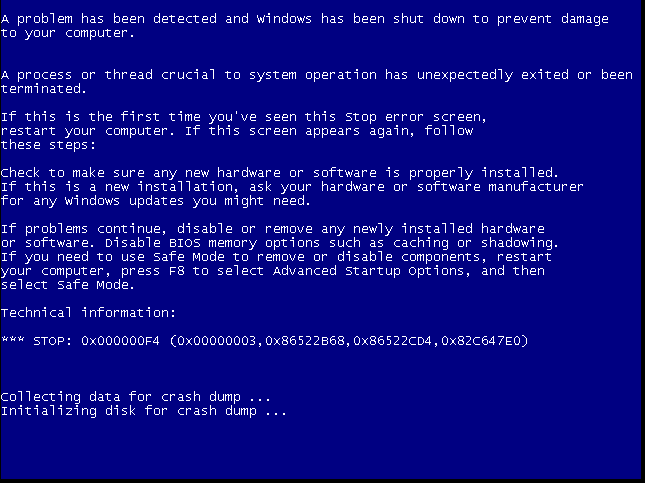
Thank you for all of your help! I really appreciate not only the code but also the explanations of how it works! Thanks again for all of your help! I am really glad how nice the PICO-8 community is =)
[Please log in to post a comment]