I'm trying to make a version of Snake and I have two tables of tables I need values pared from and removed. the code:
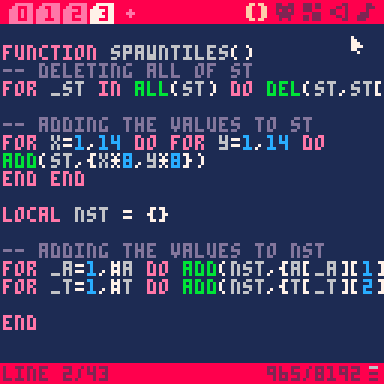
Now I need to delete all of the matching values in ST and NST but both tables could be up to a length of 196 and this game uses update60(). Any advice would be greatly appreciated, thanks.


Hi @twoThirds_Evil:
Writing a snake game is pretty interesting, isn't it ?
Here now I made a thorough tutorial on how to write your own snake game that is chock full of comments explaining every single step of the way.






Also the test cart is:
I just need an efficient way to spawn the apples not on the snake


ah! it is advisable to avoid iterating over a table (sequence) all the time, indeed. what I would do is store the positions of the snake segments in a table (key-value, using x+y*w
to make the key), so that when you spawn a tomato, it’s one efficient operation to check if there is a snake segment there.
(there are certainly better ways to do this by storing vectors that make up the current shape of the snake and using some math with that, but I’m confortable with tables and not math)


Could you potentially just use pget() to grab the color of the pixel where the center of the tomato would be (tomatox+4, tomatoy+4 would probably be close enough) and if it's the snake's color, it's invalid for the tomato? This might be too simple for what you're trying to do, but as long as the snake isn't the same color as the floor, it could work.




@2bitchuck thanks! I totally forgot about pget. Thanks for reminding me.


you didn’t get what I was saying!
make another table that’s not a sequence ({ tailpiece1, tailpiece2, tailpiece2 }
) but a normal keyed table ({ [0]=true, [15]=true, [31]=true }
), then when you generate random coordinates for an apple, it takes one operation to check if these coordinates have a tail piece (if tbl[coords] then
)




I wouldn't be afraid of scanning a 196-element table every once in a while. You're not creating apples on every frame.
For example, you might have a 196-element table where a cell is 0 if it is empty, 1 if it's an apple, and 2 or more for various types of snake pieces. Testing if specific tile is empty would just be board[row * 14 + column] == 0
. To pick an empty location at random for a new apple:
empties = {} for i=0,195 do if (board[i] == 0) add(empties, i) end new_apple = empties[flr(rnd()*#empties) + 1] new_apple_row = new_apple / 14 new_apple_column = new_apple % 14 |
From a quick test it looks like this runs in about 1/83rd of a single frame at 60 fps (0.0002 seconds).


@dddaaannn thanks. If a pget system didn't work I would have gone with your idea, but I found a solution with:
function spawntiles() -- deleting all of st for _st=1,#st do del(st,st[1]) end -- adding the values to st for x=1,14 do for y=1,14 do if( pget(x*8+3, y*8+1)!=11 and pget(x*8+3, y*8+2)!=11) add(st, {x*8,y*8}) end end end |
Then calling spawntiles() in newapple() like this:
function newapple() spawntiles() if #st!=0 then local n = st[flr(rnd(#st))+1] local x = n[1] local y = n[2] local t = (pos(x-hx)+pos(y-hy))/2 add(a,{x,y,t,true}) end end |
Then making pget work with:
local new = false for _a=1,#a do if(a[_a][1]==hx and a[_a][2]==hy and a[_a][4]) a[_a][4]=false new=true if a[_a][4] then spr(5,a[_a][1],a[_a][2]) else rect(a[_a][1]+1,a[_a][2]+1,a[_a][1]+6,a[_a][2]+6,11) end end rectfill(t[#t][2]+2,t[#t][3]+2,hx+5,hy+5,11) spr(1,hx,hy) if(new)newapple() |
Thanks, everyone for your replies and help!
[Please log in to post a comment]