This cart has devkit mode enabled and requires a mouse and keyboard.
It's intended to be run locally; not all feature work correctly in the web player.
A simple path editor for creating animation paths for game objects. The paths created are simple interpolated paths not bezier or similar curves. The path is guaranteed to pass through all of the control points. You don't have as much control over the exact shape of the path but you can still get some pretty nice results.
Features
- Save and Load path files in a binary format to .p8 files so they can be easily changed later.
- Export paths and related functions to a .txt file which can be
#include
d in your project. - When exported, paths are translated so the first control point is at (0, 0) so you can easily add any offset allowing you to use the same path in multiple locations without having to change the path itself.
- When you save a path file the filename is saved in persistent cart data and that file will be automatically loaded the next time you start the editor. (Assumes the editor and path file are in the same directory.)
- Path files contain multiple paths so you can keep all paths for a single project in a single file.
- Path names are editable so you can give them meaningful names.
- Create open or closed paths.
- A timeline allows you to adjust the animation timing of individual sections of the path as well as the total duration (in seconds) of the animation.
- Import sprite and map data from another cart. (Assumes cart is in the same directory as the editor.)
- Select sprites to preview path animation. (Currently a single, static, 8x8 sprite can be selected per path. This is just for preview purposes: selected sprites are saved in path files but are not exported.)
- Adjust map position either by dragging it or via text input. (As with sprites this is just for preview purposes. Each path can have the map displayed at a different position and those postiions are saved in the path file but not exported.)
- If you don't like my colour choices, the first tab of the .p8.png contains configuration data which you can edit to better suit your needs/tastes. (You can also suppress the help message which pops up when you run the cart from here if you want to.) I'm not sure I made everything configurable so, if I've missed something you'd like to change the colour of, feel free to let me know and I'll add it in.
Command Summary
(also available in the editor by pressing 'h')
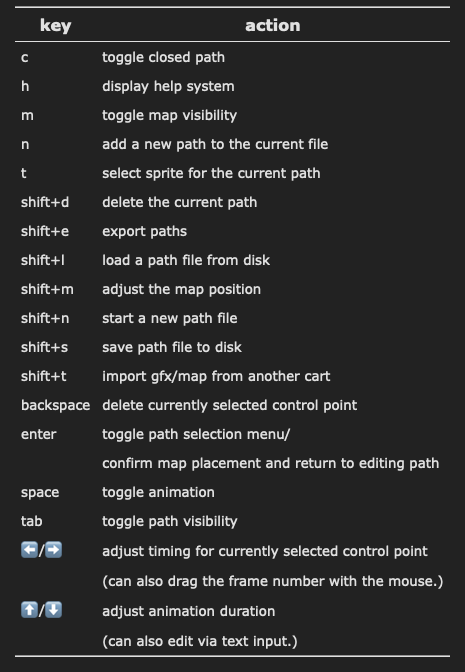
Example
A simple example using a single path to animate two squares at different offsets and with different timing.
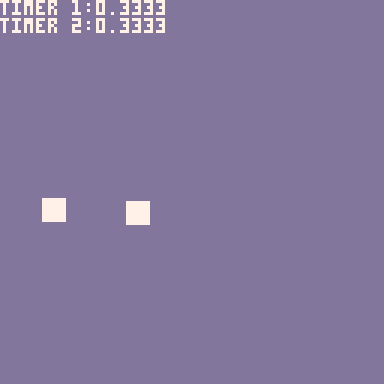
Code:
[Please log in to post a comment]