I rewrote my checkerboard demo for the Pico-8 Fantasy Console. This program is amazing. It is probably the closest thing to QBasic which I used to use. However it uses a slightly modified form of Lua that also has graphics commands. In a way it is a lot like using Love2D but I am still quite new to using Pico-8. One of the most notable features is that it has built in ability to record video to a gif file.
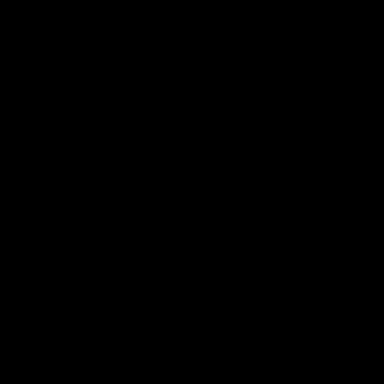
Pico-8 is almost like a mini DOS or Linux distribution except that all the programs are written in Lua. It comes with a full manual explaining the features which is available as a text file and also a page on the official website here:
https://www.lexaloffle.com/dl/docs/pico-8_manual.html
I do think that Pico-8 would be a great tool to teach people computer programming for the first time. It would allow learning Lua but also allowing a new person to start with graphics programming.


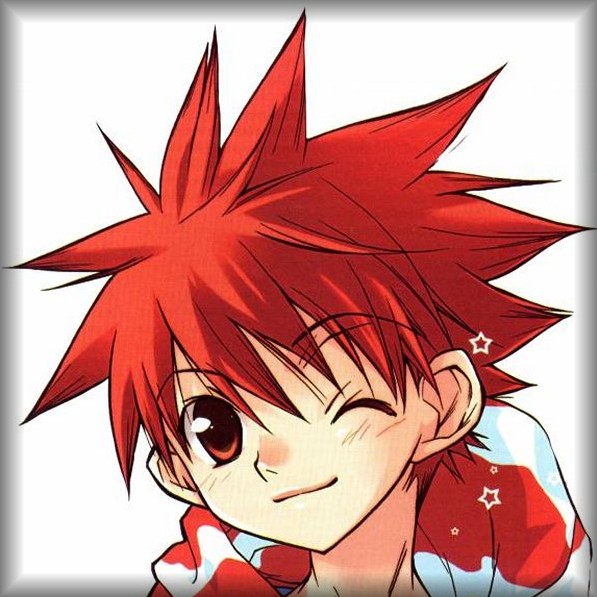
Hi @chastitywhiterose:
Not to detract you from your original work, however, this can be a bit smaller. :)
cls() for i=0,7 do for j=0,7 do if (j+i)%2==0 then rectfill(j*16,i*16,j*16+15,i*16+15,7) end end end |
Also you can use flip()
to give a bit of time back to the CPU and repeat
to make loops. _init()
_draw()
and _update()
are not required if you want to get tiny coding.
x=8 repeat cls() circfill(x,8,7) x=x+1 flip() until forever |
Making use of _init()
and _update()
are pretty standard programming practices though.
. . .
As for QBasic. I wrote a RPG Maker in that many years ago, QBasic 4.5. Good times, then I went to GFA, Blitz, and finally - here. Although I miss the 1280x720 resolution and 24-bit colors - so I have been programming in Blitz of recent.


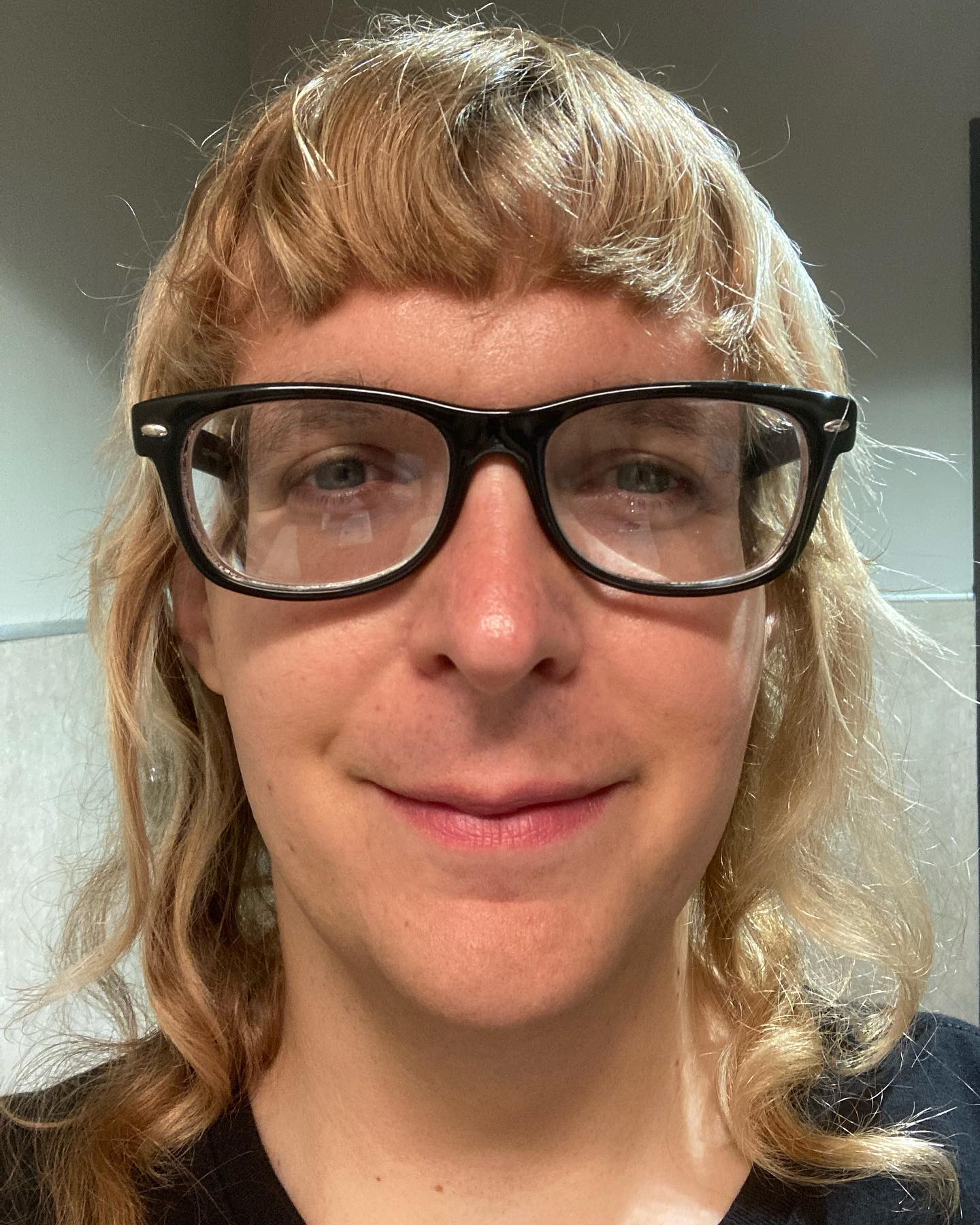
You're totally right that it can be way smaller. However I write in a way that is easy for me to understand. I always use while loops and never for loops for instance even though I can see their utility. I directly translated old C code into this checkerboard solution.
I am interested in the idea of trying to write something that doesn't use _init _draw and _update. I would try it just for fun. Strangely enough I never used functions like that in my C code but only when I was learning Lua because I started with Love2D and it introduced things that same way.


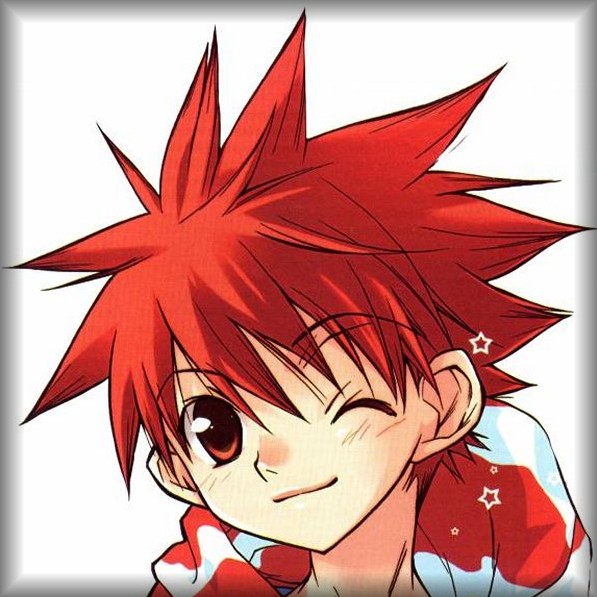
Hi @chastitywhiterose:
For small coding that does not use _init() or _update() you might be interested in looking at what are called Tweetcarts or Tweettweets.
They specialize in being 560- or 280-characters or less. Enough to place into Twitter.
https://demobasics.pixienop.net/tweetcarts/
https://twitter.com/Pico8Tweetjam
I'm from an age where every single character counted. Pico-8 itself has limitations. You can only have up to 65535 characters in your code and are limited to 8192-tokens.
To post online your code is compressed and after compression it must be less than or equal to 15616-characters.
Part of the love of Pico-8 is working within these limitations and developing incredible and astounding games, demos, and tools to assist others in coding.


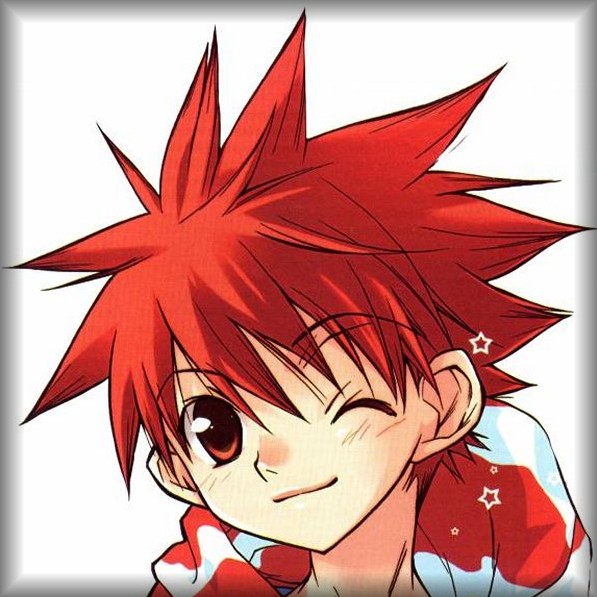
Well I'm sorta the opposite, @chastitywhiterose. I'm pretty bad at coding so I need all the memory I can get. for instance, this is some code I'm working on now.
-- draw the red line starting -- from the left side of the -- screen to the right, -- depending upon how big -- variable "a" is. line(0,110,a,110,8) -- make the line longer since -- you are holding ❎. a=a+1 -- if it reaches the right -- side: if a==128 then -- reset high-score. hisc=0 |
I need tons of remarks to help remind what the heck I'm doing - and it's also beneficial as I tend to keep those when I release something to the BBS.
I once hoped that Pico-8 would use zero bytes of memory for remarks, that would be most helpful to everyone I think. But no, instead we get code like:
kf=1-kf k=nil if a==0 and b==0 then for i=0,3 do n=i+kf*3 if btn(bnn[n]) then k=bnn[n] end end sp=1 if (btn(4)) sp=2 e=flr((x-1)/5)*5+3 f=flr((y-1)/5)*5+3 m=maze[e][f] if (m==1) maze[e][f]=6 end |
which can be hard to follow if there is no documentation explaining what's going on. Yet in many cases there is no room to do that because of the 64k coding limitation.


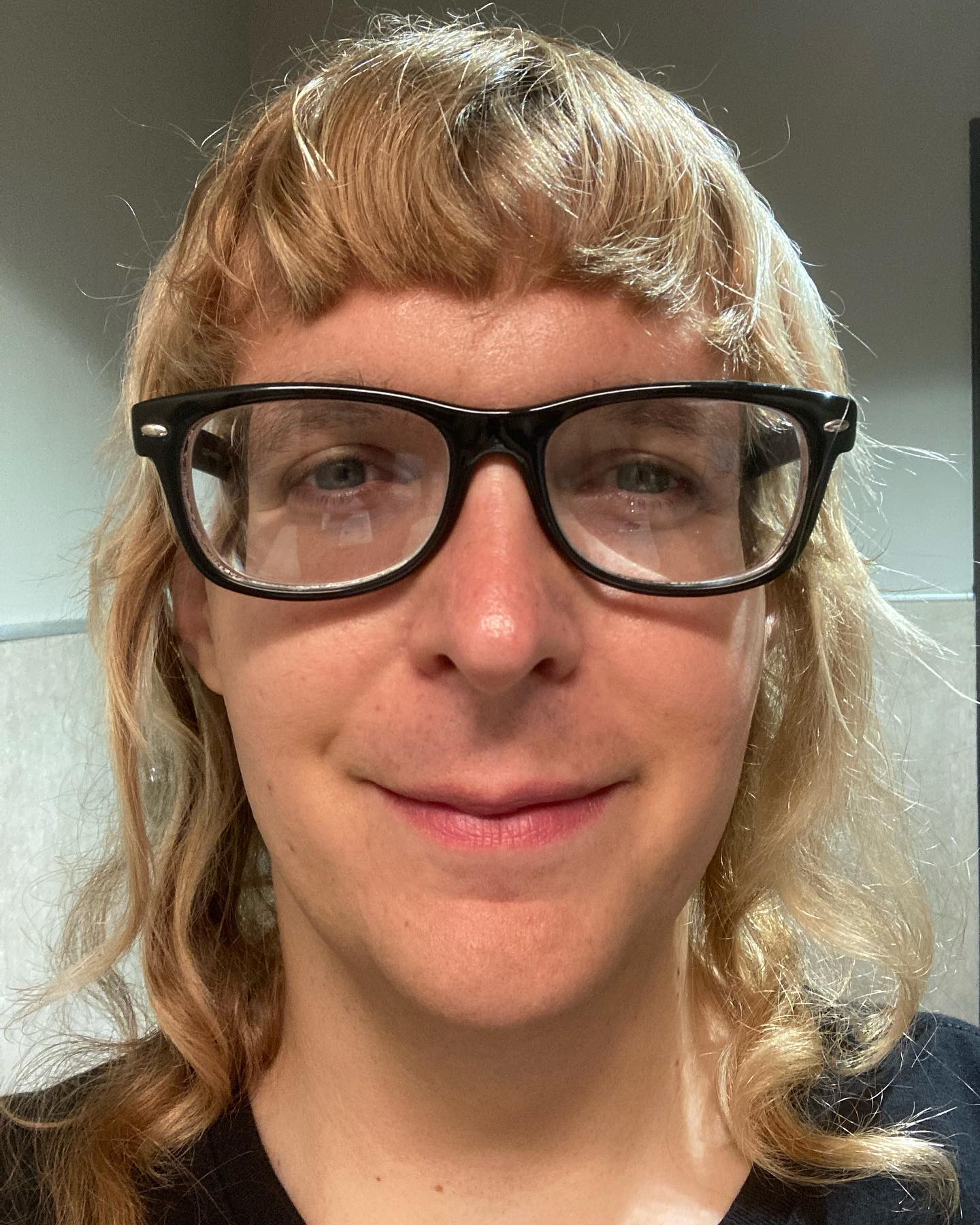
I agree there shouldn’t be a penalty for comments because that leads to unreadable code. Comments are the sign of a good programmer because you need to remember how something works.
In fact I should comment more than I do but I’m bad at explaining things even if I know how they work.


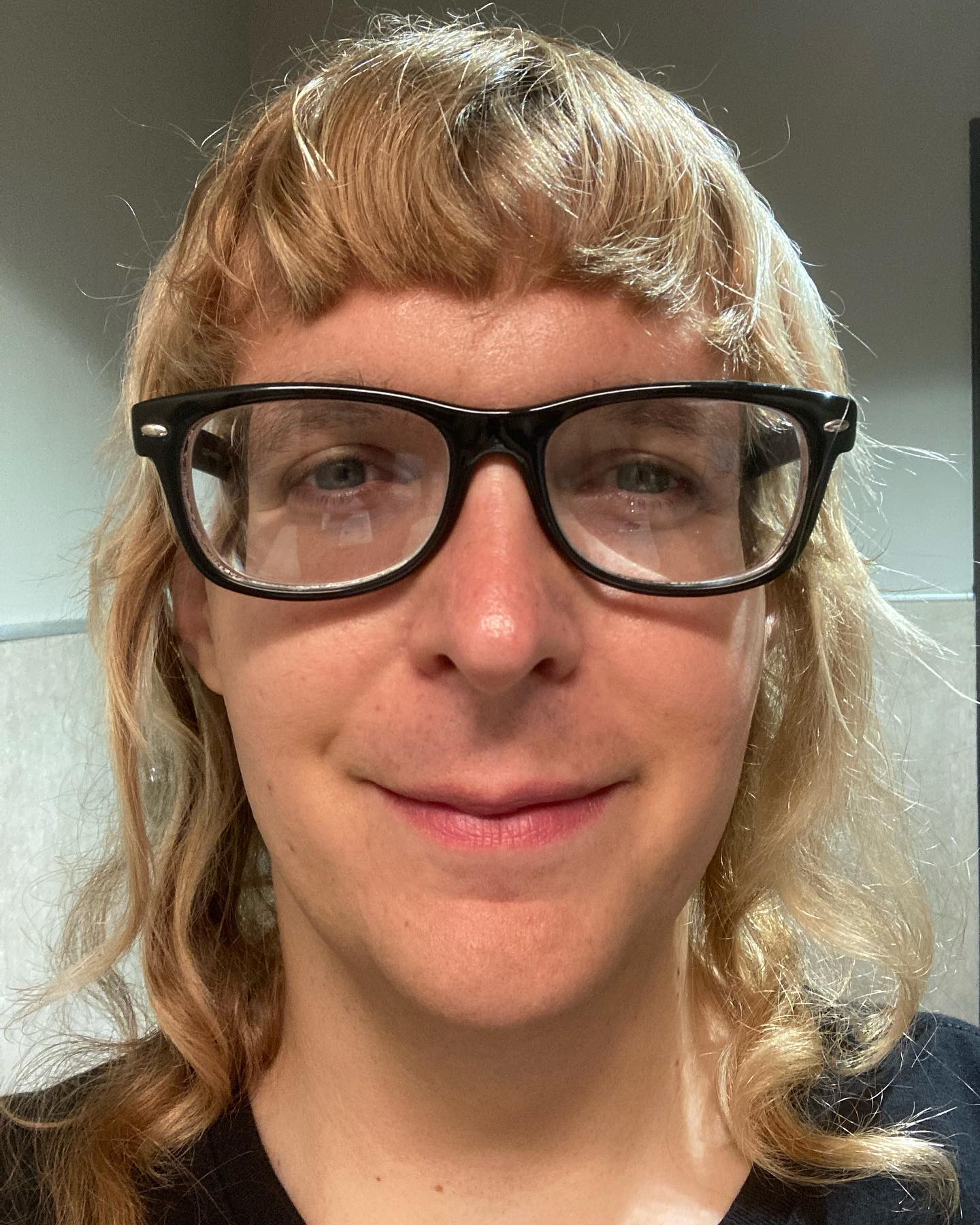
I come from a mostly C background and I have a deep understanding of pointer arithmetic and how to manipulate one dimensional arrays as if they were two dimensional.
It’s almost like I’m just clever enough to do things that are probably bad practice and yet they work for me. Just like my Tetris game I made uses some really wacky code.
I appreciate all your comments btw. I’m glad to get some conversation from someone who understands programming. Nobody on Facebook cares.


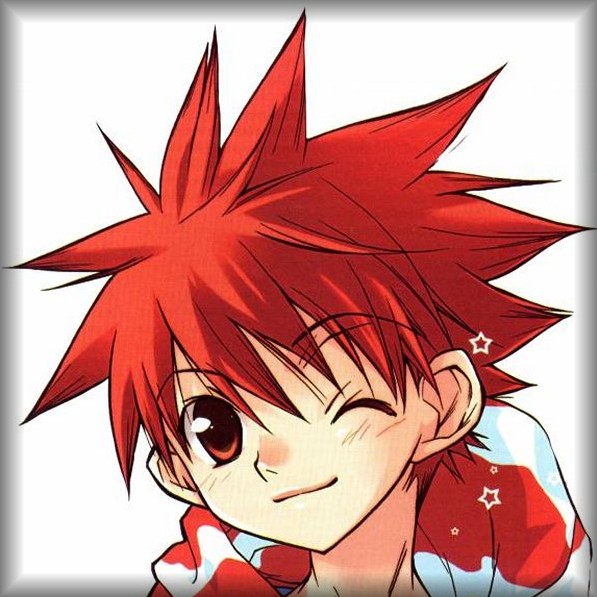
Hi @chastitywhiterose.
Happy to assist. And I, too, am glad to be talking to a fellow programmer. :)
OK as you are mentioning arrays, thought I would share.
Now 2D arrays can be tricky in Pico-8. Here for instance is a 2d checkerboard.
board={} for i=0,7 do board[i]={} for j=0,7 do board[i][j]=0 end end |
You access it via: var=board[a][b]
or board[a][b]=var
You can also swap variables in a single line. a,b=b,a
And yes, most BASIC languages would not permit that.
Arrays can get pretty crazy too if you consider BASIC standards.
fruit={}
fruit["apple"]=86.3
calc={}
calc[-34.2]="calculator"
Any array read that does not contain a value does not return 0 (zero) it instead returns NIL.


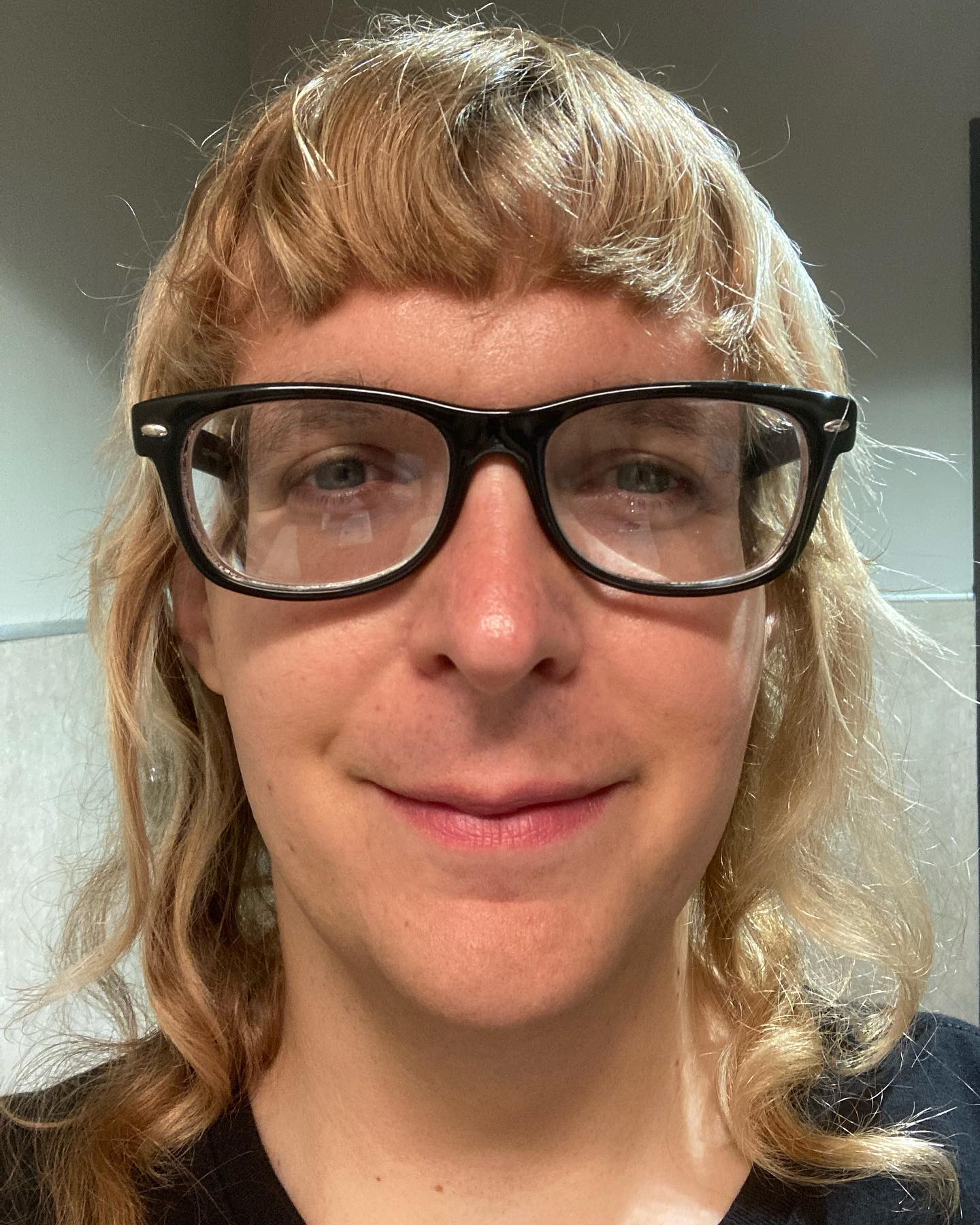
Looks like 2D arrays work similar to C if you set them up the right way first.
The reason I use my own style of one dimensional arrays as if they were 2D is because of some languages not supporting 2D arrays like bash. I used to be into bash scripting for a lot of fun but now I like Lua better as a quick easy scripting language even though I don't know it near as well as other languages. I'm a total noob to it but I have been reading Programming in Lua whenever I'm not at work or doing what my mom wants.
[Please log in to post a comment]